Arrays are used to hold the same type of data, with a size, set at the time of creation.
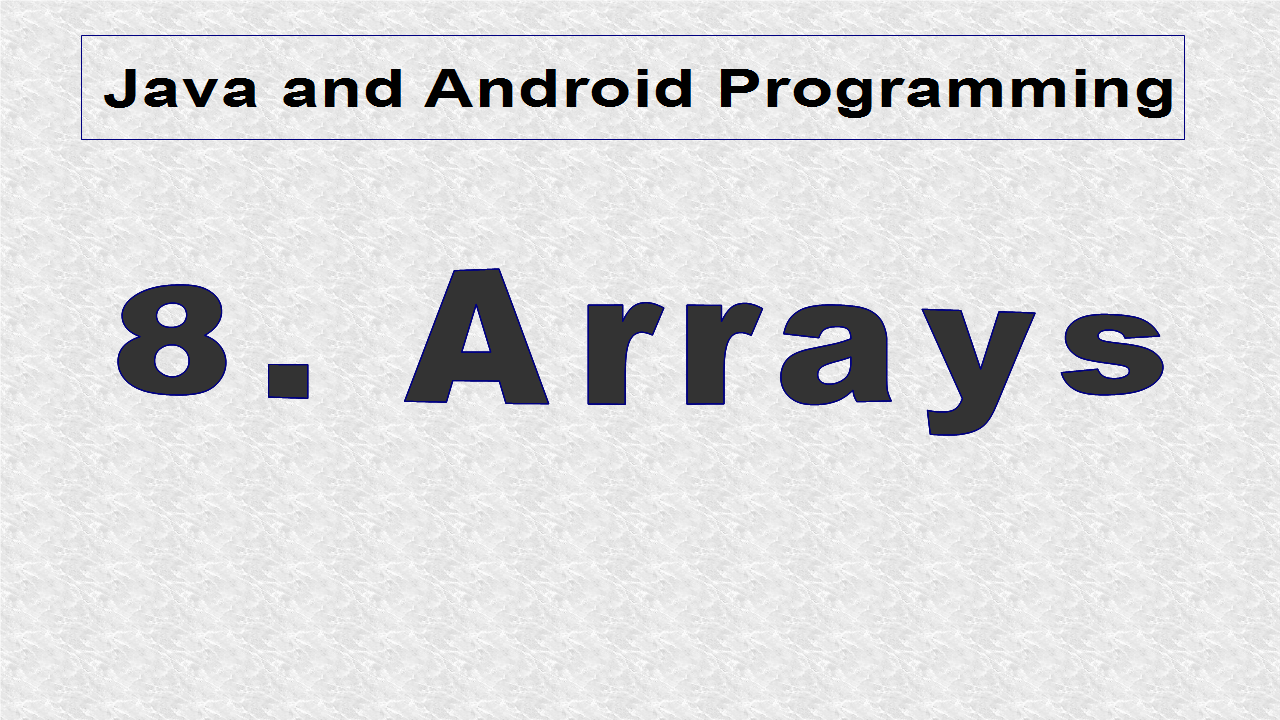
For primitive arrays, the type is a primitive.
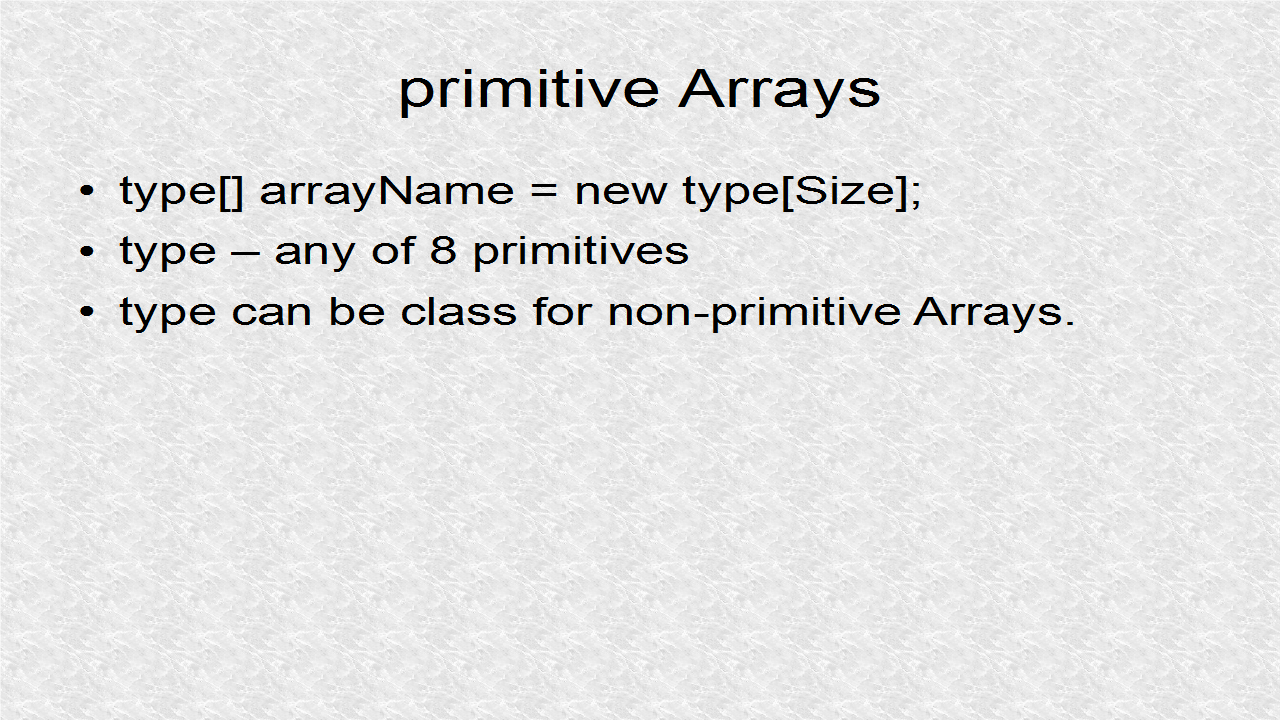
// *** 1. Start (primitives) byte[] bArr = new byte[10]; short[] sArr = new short[10]; int[] iArr = new int[10]; long[] lArr = new long[10]; boolean[] bnArr = new boolean[10]; float[] fArr = new float[10]; double[] dArr = new double[10]; char[] cArr = new char[10]; // *** 1. End
length is a field of any Array. Once the Array is created, we can get or set values using the Array name and index, within square brackets. One version of the for loop can iterate over the individual elements of the Array.
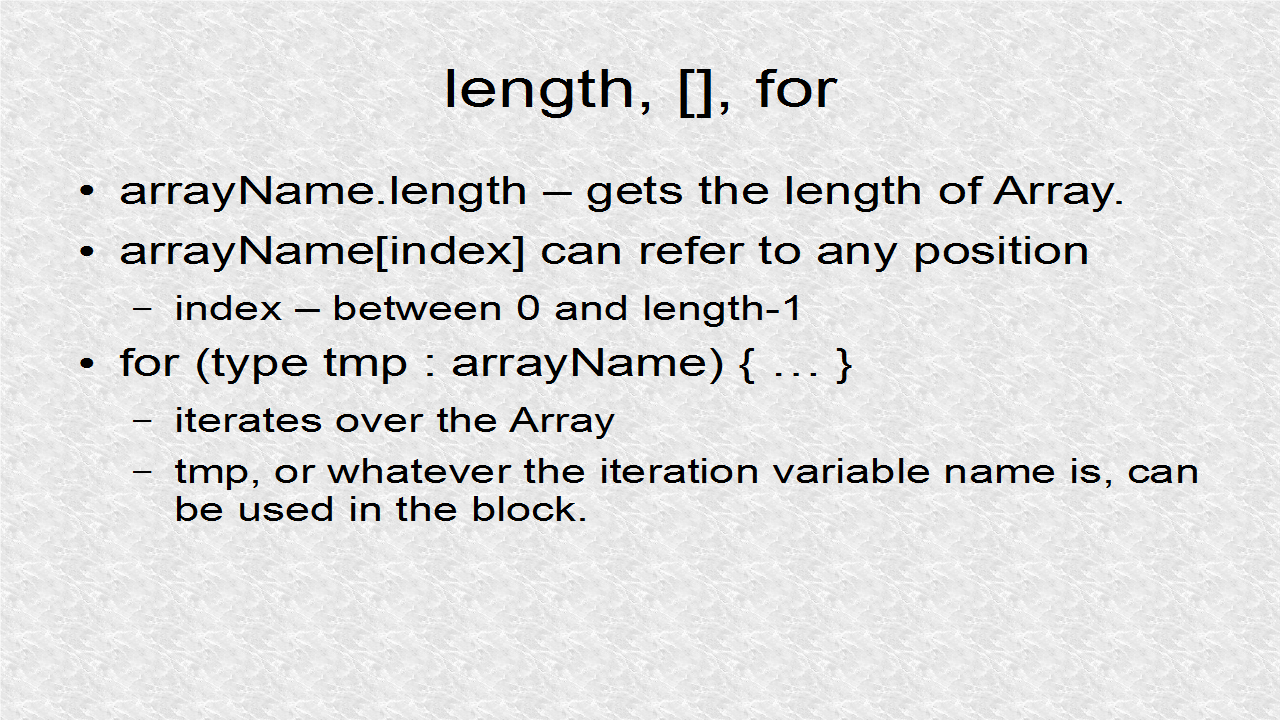
// *** 2. Start (length, [], for) System.out.println("length of iArr = " + iArr.length); iArr[0] = 9; iArr[5] = 8; for (int val: iArr) System.out.print("val = " + val + ", "); // *** 2. End
The type of Array can be a class such as built-in String or even a class we write. If we know all the values, we can put them in brackets, and the compiler will calculate the size.
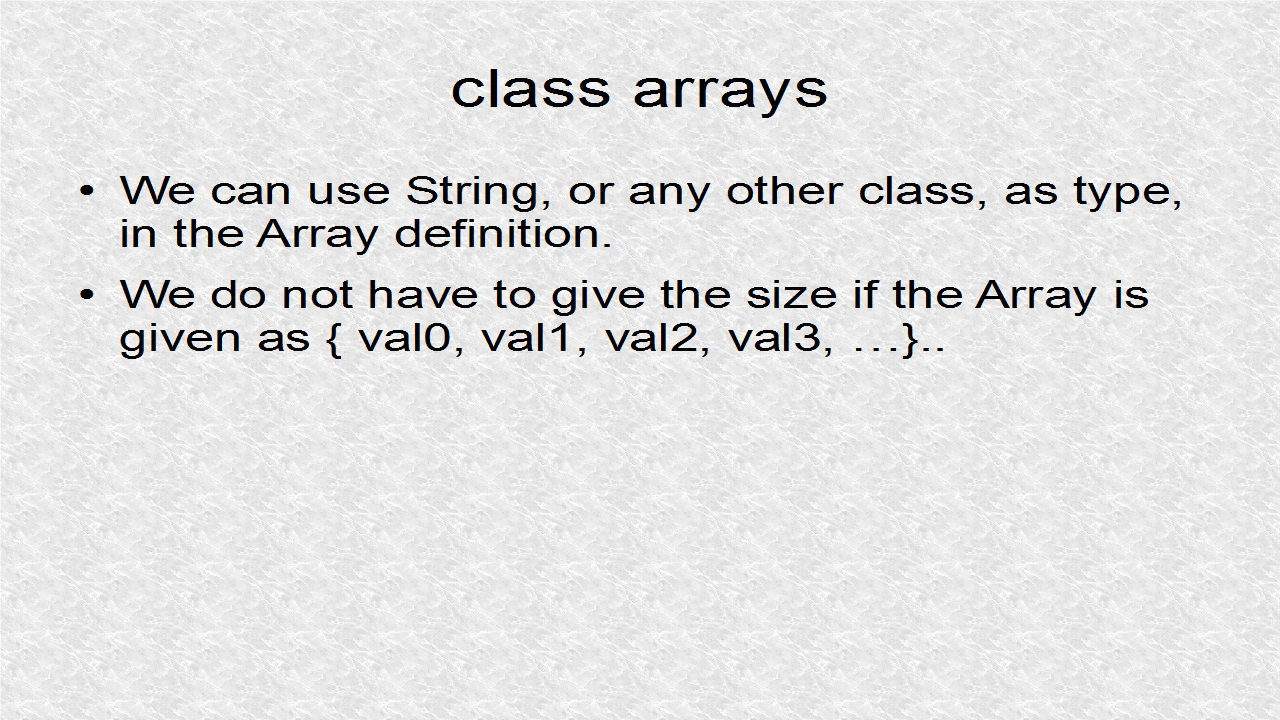
// *** 3. Start (String) System.out.println("\nStarting 3..."); String[] strs = new String [10]; strs[0] = "1 2 3"; strs[1] = "4 5 6"; strs[2] = strs[1].replace(" ", "-"); for (String s: strs) System.out.print("s = " + s + ", "); // *** 3. End
For any Array, we can use utility methods in java.util.Arrays. The use of these methods let's us avoid the use of for loops.
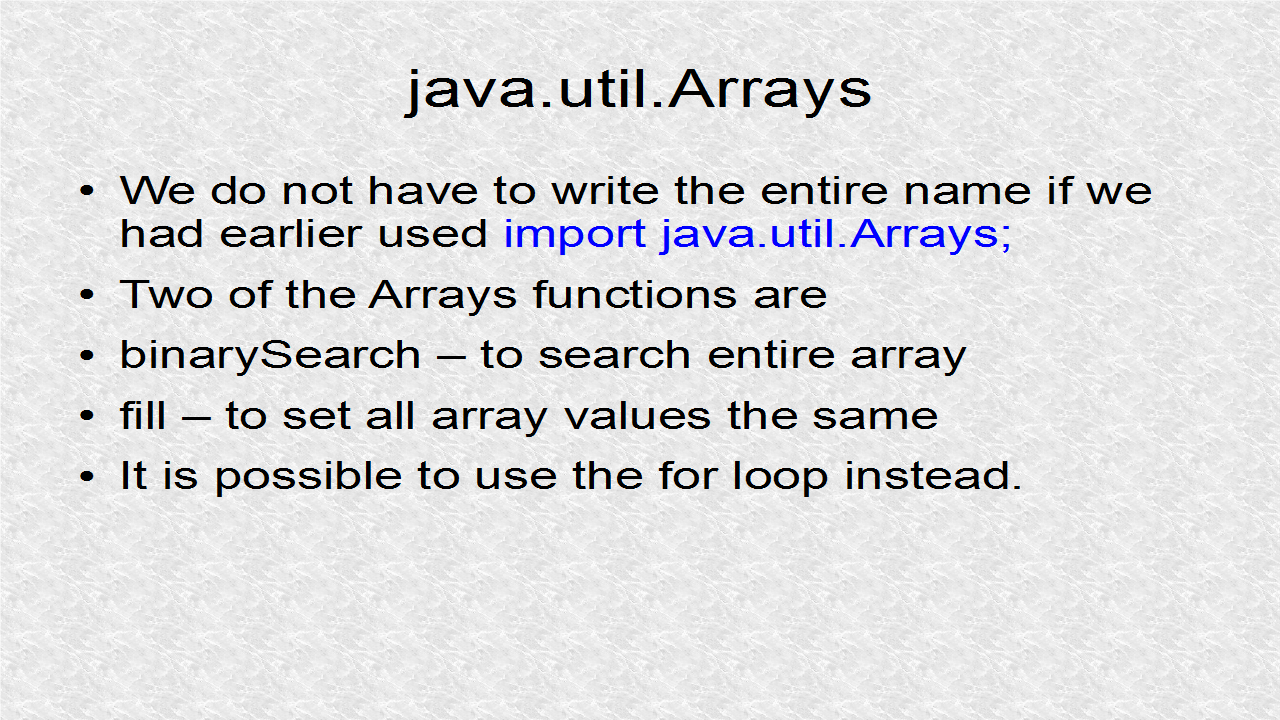
// *** 4. Start (java.util.Arrays) System.out.println("\nStarting 4..."); String[] strs2 = {"This","Hello","world"}; for (String s: strs2) System.out.print("s = " + s + ", "); int search = java.util.Arrays.binarySearch(strs2, "Hello"); System.out.println("\nsearch = " + search); java.util.Arrays.fill(strs2, "-hello-"); for (String s: strs2) System.out.print("s = " + s + ", "); // *** 4. End
// ex8.jpage // *** 1. Start (primitives) byte[] bArr = new byte[10]; short[] sArr = new short[10]; int[] iArr = new int[10]; long[] lArr = new long[10]; boolean[] bnArr = new boolean[10]; float[] fArr = new float[10]; double[] dArr = new double[10]; char[] cArr = new char[10]; // *** 1. End // *** 2. Start (length, [], for) System.out.println("length of iArr = " + iArr.length); iArr[0] = 9; iArr[5] = 8; for (int val: iArr) System.out.print("val = " + val + ", "); // *** 2. End // *** 3. Start (String) System.out.println("\nStarting 3..."); String[] strs = new String [10]; strs[0] = "1 2 3"; strs[1] = "4 5 6"; strs[2] = strs[1].replace(" ", "-"); for (String s: strs) System.out.print("s = " + s + ", "); // *** 3. End // *** 4. Start (java.util.Arrays) System.out.println("\nStarting 4..."); String[] strs2 = {"This","Hello","world"}; for (String s: strs2) System.out.print("s = " + s + ", "); int search = java.util.Arrays.binarySearch(strs2, "Hello"); System.out.println("\nsearch = " + search); java.util.Arrays.fill(strs2, "-hello-"); for (String s: strs2) System.out.print("s = " + s + ", "); // *** 4. End
No comments:
Post a Comment