A mesh can be created programmatically in jMonkeyEngine.
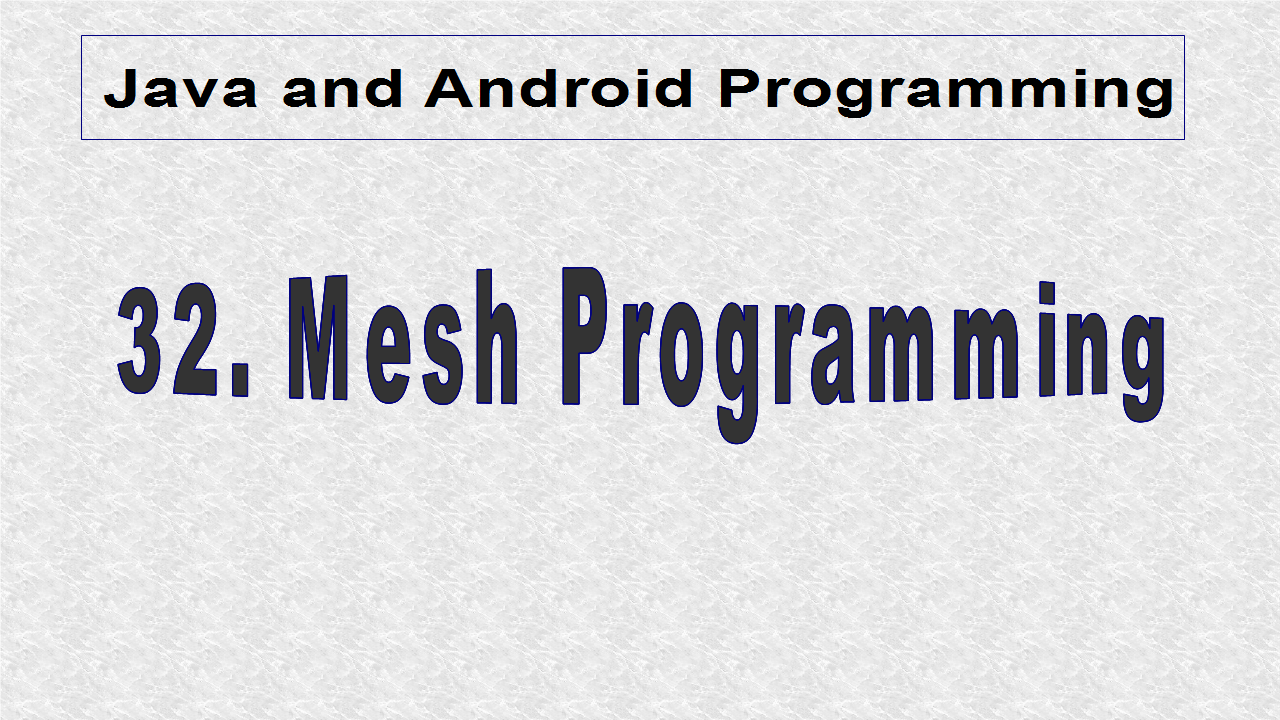
The cube has 24 vertex points with 8 physical vertex points, each with 3 normals.
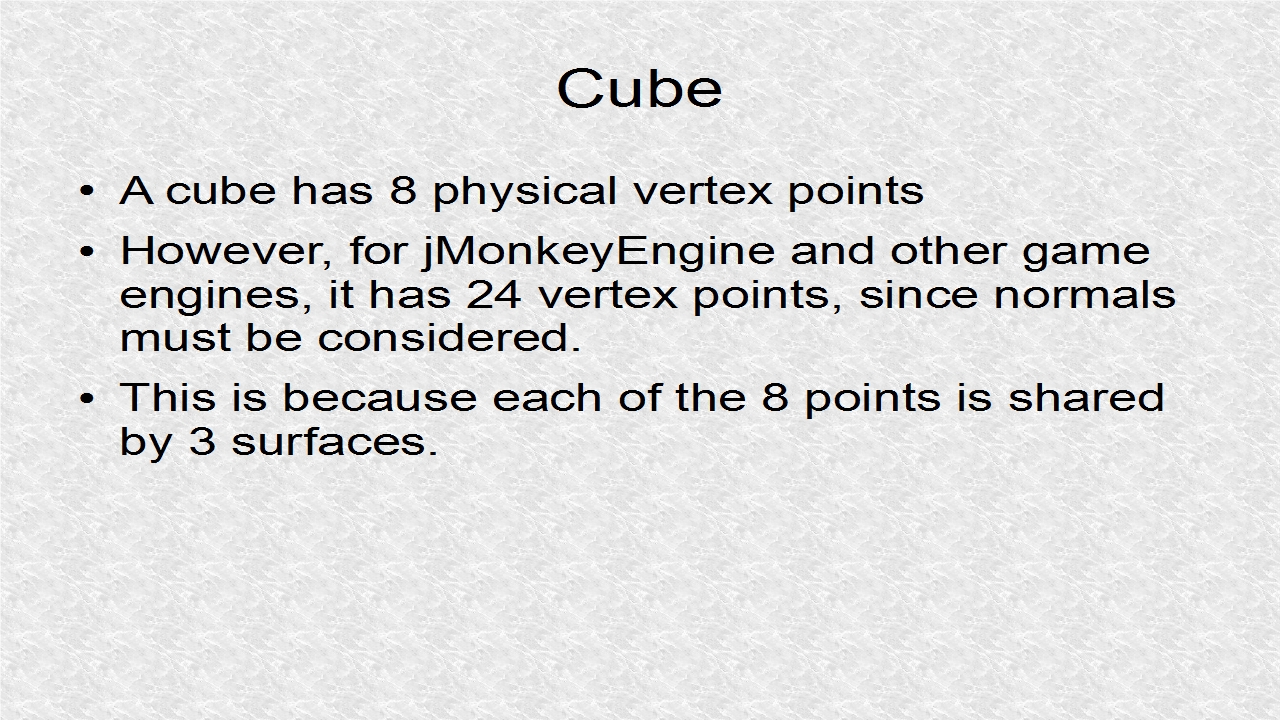
// *** 1. Start (24 pts in cube with normal) Vector3f [] vert = new Vector3f[24]; vert[0] = new Vector3f(-1, -1, -1); // -z vert[1] = new Vector3f(1, -1, -1); // -z vert[2] = new Vector3f(1, 1, -1); // -z vert[3] = new Vector3f(-1, 1, -1); // -z vert[4] = new Vector3f(1, -1, -1); // +x vert[5] = new Vector3f(1, -1, 1); // +x vert[6] = new Vector3f(1, 1, 1); // +x vert[7] = new Vector3f(1, 1, -1); // +x vert[8] = new Vector3f(1, -1, 1); // +z vert[9] = new Vector3f(-1, -1, 1); // +z vert[10] = new Vector3f(-1, 1, 1); // +z vert[11] = new Vector3f(1, 1, 1); // +z vert[12] = new Vector3f(-1, -1, 1); // -x vert[13] = new Vector3f(-1, -1, -1); // -x vert[14] = new Vector3f(-1, 1, -1); // -x vert[15] = new Vector3f(-1, 1, 1); // -x vert[16] = new Vector3f(1, 1, -1); // +y vert[17] = new Vector3f(1, 1, 1); // +y vert[18] = new Vector3f(-1, 1, 1); // +y vert[19] = new Vector3f(-1, 1, -1); // +y vert[20] = new Vector3f(-1, -1, -1); // -y vert[21] = new Vector3f(-1, -1, 1); // -y vert[22] = new Vector3f(1, -1, 1); // -y vert[23] = new Vector3f(1, -1, -1); // -y // *** 1. End
For the 24 vertex points, we have to give the normal. With the vertex information and normal information, all the 24 entries are now unique.
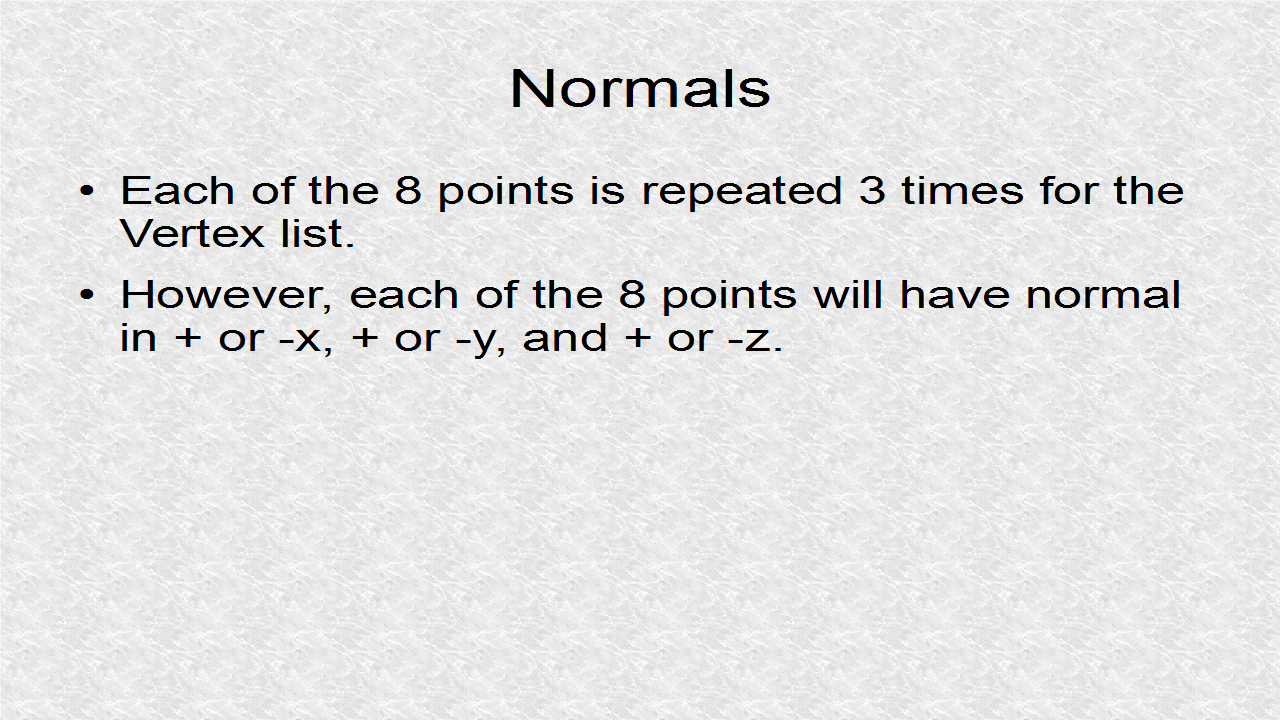
// *** 2. Start (Normals for the Verts) Vector3f[] norm = new Vector3f[24]; norm[0] = new Vector3f(0, 0, -1); // -z norm[1] = new Vector3f(0, 0, -1); // -z norm[2] = new Vector3f(0, 0, -1); // -z norm[3] = new Vector3f(0, 0, -1); // -z norm[4] = new Vector3f(1, 0, 0); // +x norm[5] = new Vector3f(1, 0, 0); // +x norm[6] = new Vector3f(1, 0, 0); // +x norm[7] = new Vector3f(1, 0, 0); // +x norm[8] = new Vector3f(0, 0, 1); // +z norm[9] = new Vector3f(0, 0, 1); // +z norm[10] = new Vector3f(0, 0, 1); // +z norm[11] = new Vector3f(0, 0, 1); // +z norm[12] = new Vector3f(-1, 0, 0); // -x norm[13] = new Vector3f(-1, 0, 0); // -x norm[14] = new Vector3f(-1, 0, 0); // -x norm[15] = new Vector3f(-1, 0, 0); // -x norm[16] = new Vector3f(0, 1, 0); // +y norm[17] = new Vector3f(0, 1, 0); // +y norm[18] = new Vector3f(0, 1, 0); // +y norm[19] = new Vector3f(0, 1, 0); // +y norm[20] = new Vector3f(0, -1, 0); // -y norm[21] = new Vector3f(0, -1, 0); // -y norm[22] = new Vector3f(0, -1, 0); // -y norm[23] = new Vector3f(0, -1, 0); // -y // *** 2. End
Inside the program, we can create our UV mapping, based on the image. Here, the image was 3 by 2, and these divisions corresponds to the 6 faces.
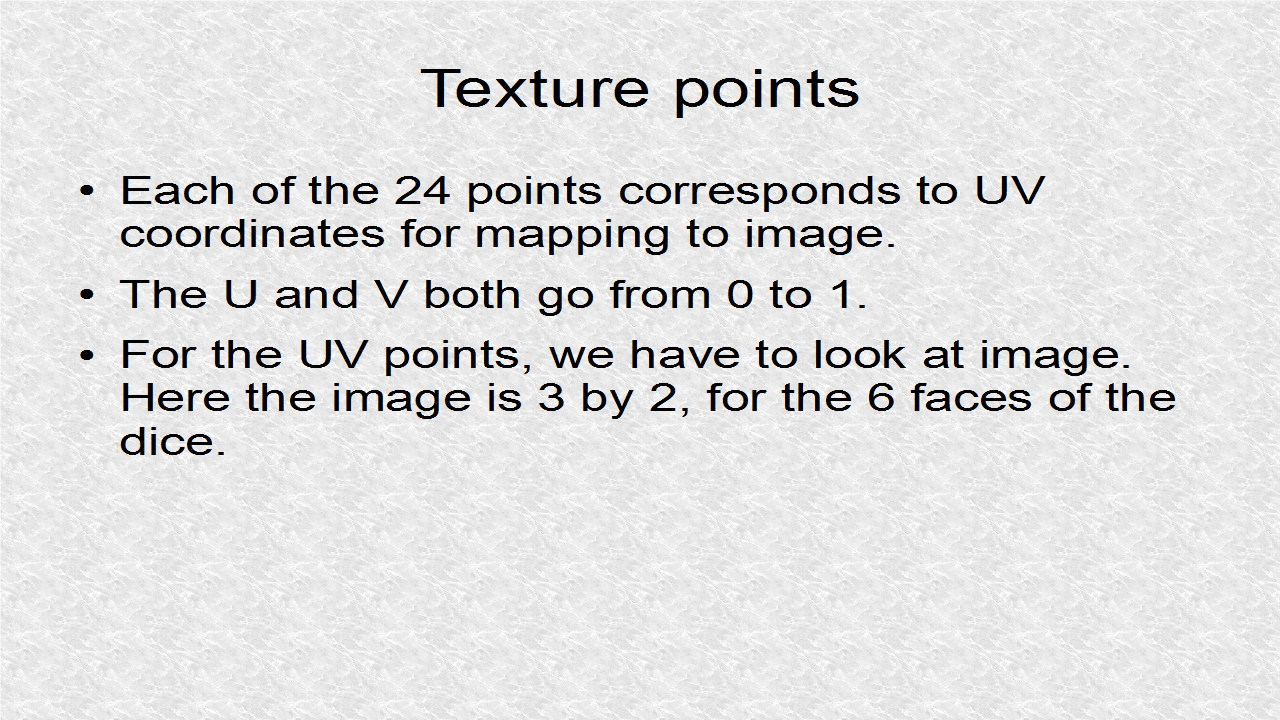
// *** 3. Start (Texture coordinates for the Verts) Vector2f [] texCoord = new Vector2f[24]; texCoord[0] = new Vector2f(0,0); texCoord[1] = new Vector2f(THIRD,0); texCoord[2] = new Vector2f(THIRD,0.5f); texCoord[3] = new Vector2f(0,0.5f); texCoord[4] = new Vector2f(THIRD,0); texCoord[5] = new Vector2f(2*THIRD,0); texCoord[6] = new Vector2f(2*THIRD,0.5f); texCoord[7] = new Vector2f(THIRD,0.5f); texCoord[8] = new Vector2f(2*THIRD,0); texCoord[9] = new Vector2f(1,0); texCoord[10] = new Vector2f(1,0.5f); texCoord[11] = new Vector2f(2*THIRD,0.5f); texCoord[12] = new Vector2f(0,0.5f); texCoord[13] = new Vector2f(THIRD,0.5f); texCoord[14] = new Vector2f(THIRD,1); texCoord[15] = new Vector2f(0,1); texCoord[16] = new Vector2f(THIRD,0.5f); texCoord[17] = new Vector2f(2*THIRD,0.5f); texCoord[18] = new Vector2f(2*THIRD,1); texCoord[19] = new Vector2f(THIRD,1); texCoord[20] = new Vector2f(2*THIRD,0.5f); texCoord[21] = new Vector2f(1,0.5f); texCoord[22] = new Vector2f(1,1); texCoord[23] = new Vector2f(2*THIRD,1); // *** 3. End
dice.png texture
For the 6 faces, we have to give the 12 counter clockwise triangles.
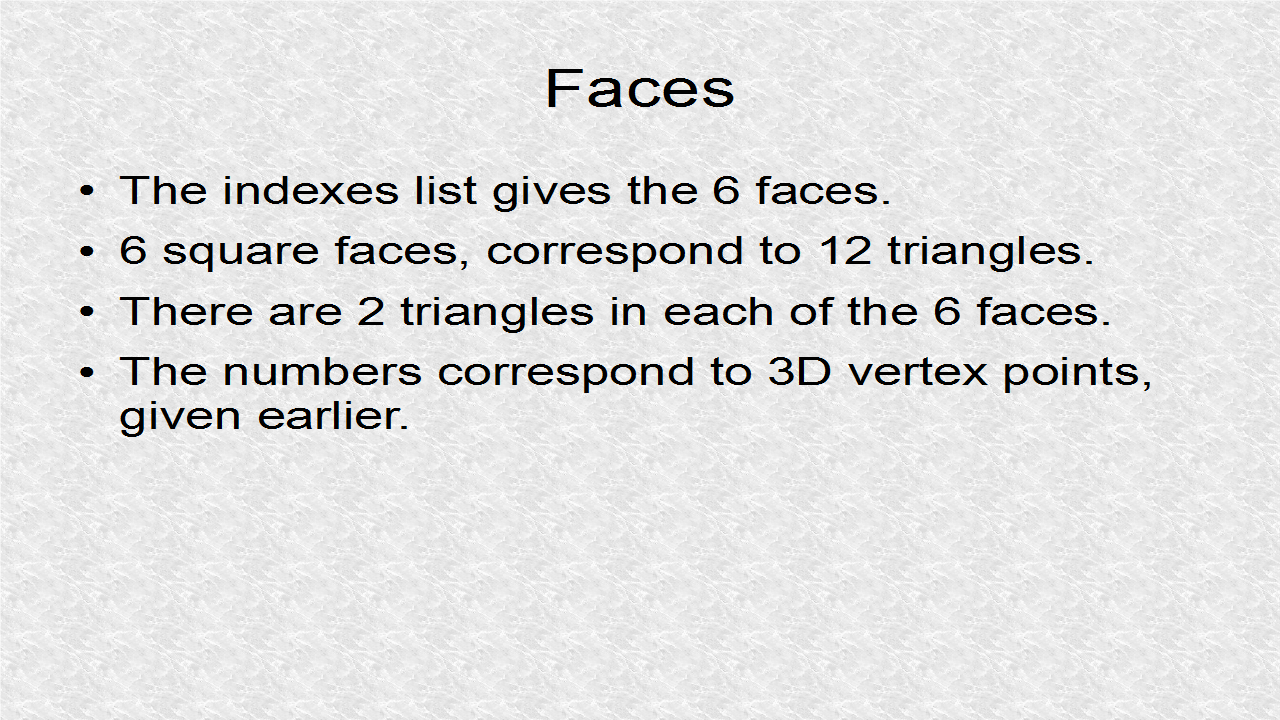
// *** 4. Start (// Indexes. 12 CCW Triangles) int [] indexes = { 3,2,1, 1,0,3, // -z 7,6,5, 5,4,7, // +x 11,10,9, 9,8,11, // +z 15,14,13, 13,12,15, // -x 19,18,17, 17,16,19, // +y 23,22,21, 21,20,23 // -y }; // *** 4. End
Now that we have the lists, we can create our mesh.
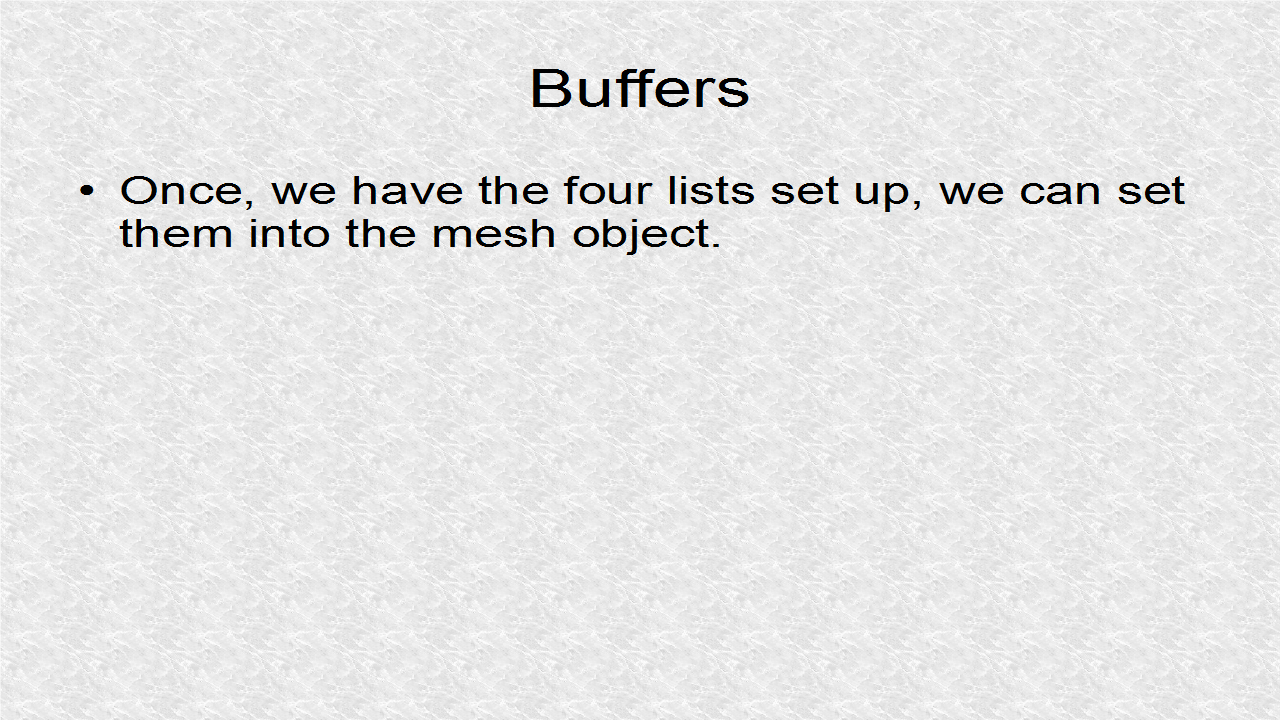
// *** 5. Start (Setting buffers) m.setBuffer(Type.Position, 3, BufferUtils.createFloatBuffer(vert)); m.setBuffer(Type.Normal, 3, BufferUtils.createFloatBuffer(norm)); m.setBuffer(Type.TexCoord, 2, BufferUtils.createFloatBuffer(texCoord)); m.setBuffer(Type.Index, 1, BufferUtils.createIntBuffer(indexes)); m.updateBound(); // *** 5. End
We have to set the texture from external image file.
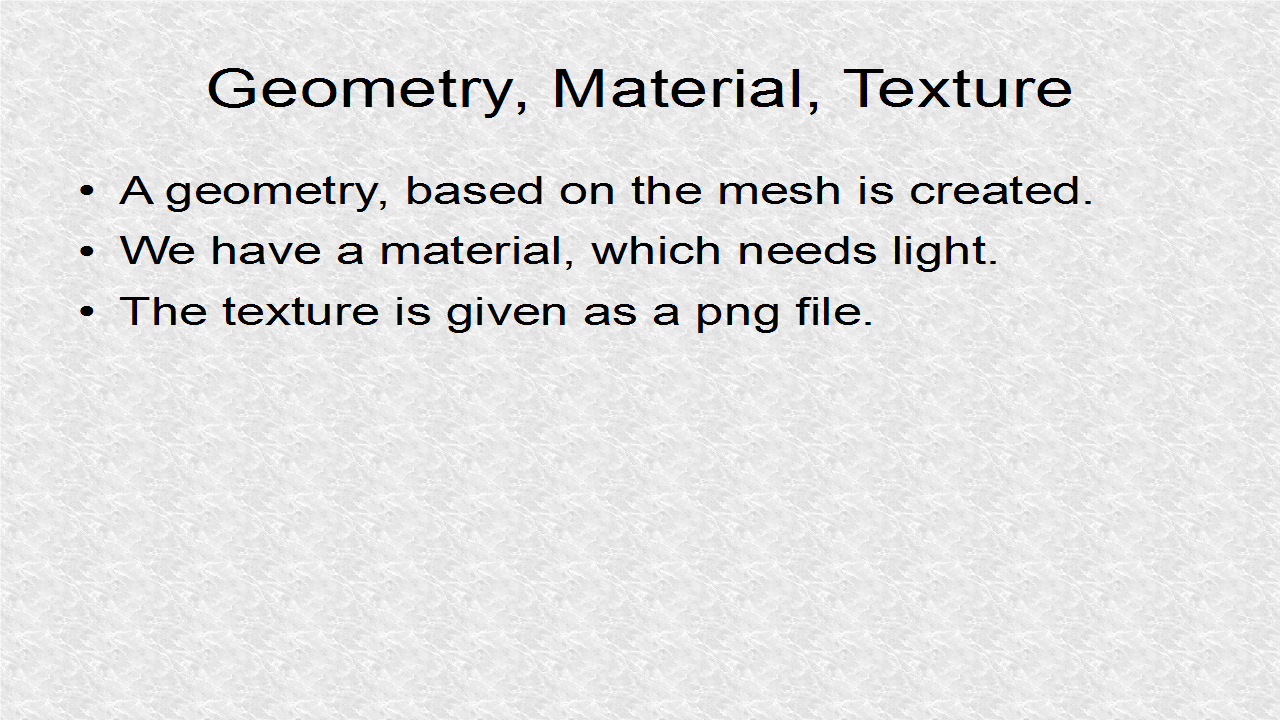
// *** 6. Start (Geometry, Material, Texture) Geometry geom = new Geometry("DiceMesh", m); Material mat = new Material(assetManager, "Common/MatDefs/Light/Lighting.j3md"); Texture matTex = assetManager.loadTexture( "Textures/dice.png"); mat.setTexture("DiffuseMap", matTex); mat.setColor("Diffuse",ColorRGBA.Red); mat.setColor("Specular",ColorRGBA.White); mat.setFloat("Shininess", 32f); // [0,128] geom.setMaterial(mat); rootNode.attachChild(geom); // *** 6. End
The lights have to illuminate the scene.
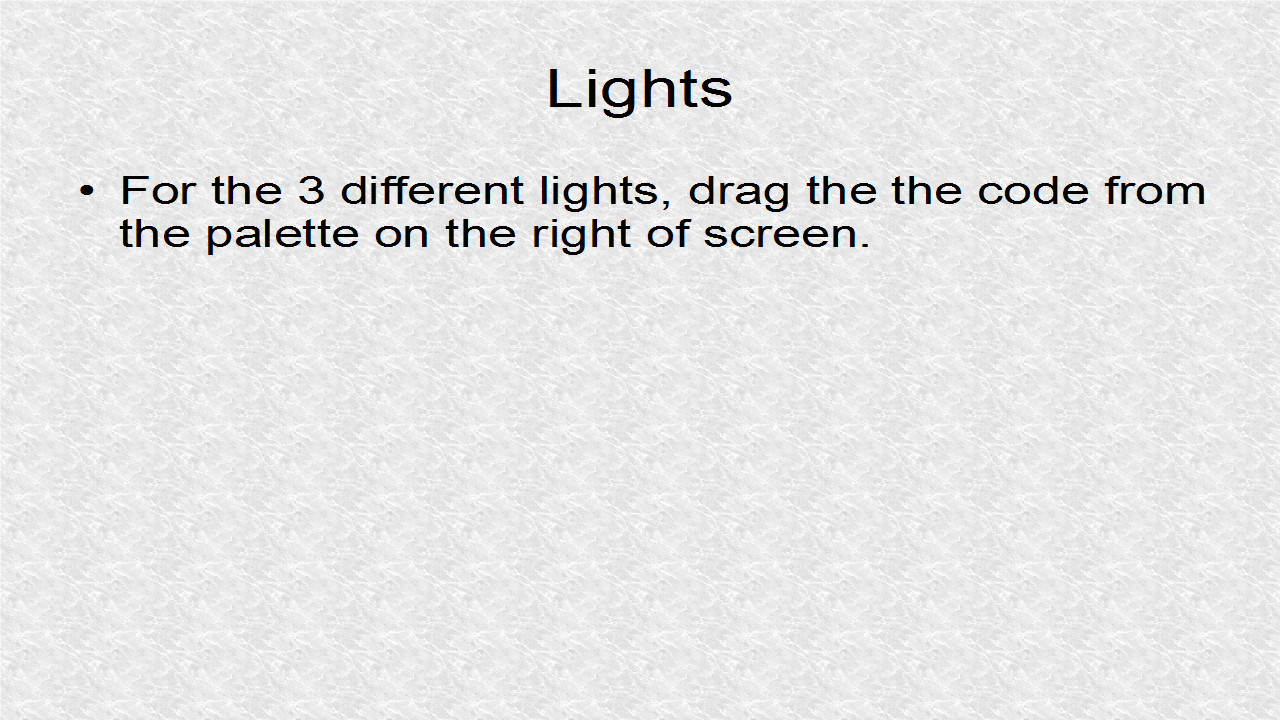
// *** 7. Start (Lights, Drag from Palette) /** A white, directional light source */ DirectionalLight sun = new DirectionalLight(); sun.setDirection((new Vector3f(-0.5f, -0.5f, -0.5f)).normalizeLocal()); sun.setColor(ColorRGBA.White); rootNode.addLight(sun); /** A white ambient light source. */ /** A cone-shaped spotlight with location, direction, range */ SpotLight spot = new SpotLight(); spot = new SpotLight(); spot.setSpotRange(100); spot.setSpotOuterAngle(20 * FastMath.DEG_TO_RAD); spot.setSpotInnerAngle(15 * FastMath.DEG_TO_RAD); spot.setDirection(cam.getDirection()); spot.setPosition(cam.getLocation()); rootNode.addLight(spot); AmbientLight ambient = new AmbientLight(); ambient.setColor(ColorRGBA.Red); rootNode.addLight(ambient); // *** 7. End
package mygame; import com.jme3.app.SimpleApplication; import com.jme3.light.AmbientLight; import com.jme3.light.DirectionalLight; import com.jme3.light.SpotLight; import com.jme3.material.Material; import com.jme3.math.ColorRGBA; import com.jme3.math.FastMath; import com.jme3.math.Vector2f; import com.jme3.math.Vector3f; import com.jme3.scene.Geometry; import com.jme3.scene.Mesh; import com.jme3.scene.VertexBuffer.Type; import com.jme3.system.AppSettings; import com.jme3.texture.Texture; import com.jme3.util.BufferUtils; public class JMonkey32 extends SimpleApplication { public static void main(String[] args){ AppSettings cfg = new AppSettings(true); cfg.setFrameRate(60); JMonkey32 app = new JMonkey32(); app.setSettings(cfg); app.setDisplayFps(false); app.setDisplayStatView(false); app.start(); } @Override public void simpleInitApp() { final float THIRD = 1f/3f; Mesh m = new Mesh(); viewPort.setBackgroundColor(ColorRGBA.White); flyCam.setEnabled(false); // *** 1. Start (24 pts in cube with normal) Vector3f [] vert = new Vector3f[24]; vert[0] = new Vector3f(-1, -1, -1); // -z vert[1] = new Vector3f(1, -1, -1); // -z vert[2] = new Vector3f(1, 1, -1); // -z vert[3] = new Vector3f(-1, 1, -1); // -z vert[4] = new Vector3f(1, -1, -1); // +x vert[5] = new Vector3f(1, -1, 1); // +x vert[6] = new Vector3f(1, 1, 1); // +x vert[7] = new Vector3f(1, 1, -1); // +x vert[8] = new Vector3f(1, -1, 1); // +z vert[9] = new Vector3f(-1, -1, 1); // +z vert[10] = new Vector3f(-1, 1, 1); // +z vert[11] = new Vector3f(1, 1, 1); // +z vert[12] = new Vector3f(-1, -1, 1); // -x vert[13] = new Vector3f(-1, -1, -1); // -x vert[14] = new Vector3f(-1, 1, -1); // -x vert[15] = new Vector3f(-1, 1, 1); // -x vert[16] = new Vector3f(1, 1, -1); // +y vert[17] = new Vector3f(1, 1, 1); // +y vert[18] = new Vector3f(-1, 1, 1); // +y vert[19] = new Vector3f(-1, 1, -1); // +y vert[20] = new Vector3f(-1, -1, -1); // -y vert[21] = new Vector3f(-1, -1, 1); // -y vert[22] = new Vector3f(1, -1, 1); // -y vert[23] = new Vector3f(1, -1, -1); // -y // *** 1. End // *** 2. Start (Normals for the Verts) Vector3f[] norm = new Vector3f[24]; norm[0] = new Vector3f(0, 0, -1); // -z norm[1] = new Vector3f(0, 0, -1); // -z norm[2] = new Vector3f(0, 0, -1); // -z norm[3] = new Vector3f(0, 0, -1); // -z norm[4] = new Vector3f(1, 0, 0); // +x norm[5] = new Vector3f(1, 0, 0); // +x norm[6] = new Vector3f(1, 0, 0); // +x norm[7] = new Vector3f(1, 0, 0); // +x norm[8] = new Vector3f(0, 0, 1); // +z norm[9] = new Vector3f(0, 0, 1); // +z norm[10] = new Vector3f(0, 0, 1); // +z norm[11] = new Vector3f(0, 0, 1); // +z norm[12] = new Vector3f(-1, 0, 0); // -x norm[13] = new Vector3f(-1, 0, 0); // -x norm[14] = new Vector3f(-1, 0, 0); // -x norm[15] = new Vector3f(-1, 0, 0); // -x norm[16] = new Vector3f(0, 1, 0); // +y norm[17] = new Vector3f(0, 1, 0); // +y norm[18] = new Vector3f(0, 1, 0); // +y norm[19] = new Vector3f(0, 1, 0); // +y norm[20] = new Vector3f(0, -1, 0); // -y norm[21] = new Vector3f(0, -1, 0); // -y norm[22] = new Vector3f(0, -1, 0); // -y norm[23] = new Vector3f(0, -1, 0); // -y // *** 2. End // *** 3. Start (Texture coordinates for the Verts) Vector2f [] texCoord = new Vector2f[24]; texCoord[0] = new Vector2f(0,0); texCoord[1] = new Vector2f(THIRD,0); texCoord[2] = new Vector2f(THIRD,0.5f); texCoord[3] = new Vector2f(0,0.5f); texCoord[4] = new Vector2f(THIRD,0); texCoord[5] = new Vector2f(2*THIRD,0); texCoord[6] = new Vector2f(2*THIRD,0.5f); texCoord[7] = new Vector2f(THIRD,0.5f); texCoord[8] = new Vector2f(2*THIRD,0); texCoord[9] = new Vector2f(1,0); texCoord[10] = new Vector2f(1,0.5f); texCoord[11] = new Vector2f(2*THIRD,0.5f); texCoord[12] = new Vector2f(0,0.5f); texCoord[13] = new Vector2f(THIRD,0.5f); texCoord[14] = new Vector2f(THIRD,1); texCoord[15] = new Vector2f(0,1); texCoord[16] = new Vector2f(THIRD,0.5f); texCoord[17] = new Vector2f(2*THIRD,0.5f); texCoord[18] = new Vector2f(2*THIRD,1); texCoord[19] = new Vector2f(THIRD,1); texCoord[20] = new Vector2f(2*THIRD,0.5f); texCoord[21] = new Vector2f(1,0.5f); texCoord[22] = new Vector2f(1,1); texCoord[23] = new Vector2f(2*THIRD,1); // *** 3. End // *** 4. Start (// Indexes. 12 CCW Triangles) int [] indexes = { 3,2,1, 1,0,3, // -z 7,6,5, 5,4,7, // +x 11,10,9, 9,8,11, // +z 15,14,13, 13,12,15, // -x 19,18,17, 17,16,19, // +y 23,22,21, 21,20,23 // -y }; // *** 4. End // *** 5. Start (Setting buffers) m.setBuffer(Type.Position, 3, BufferUtils.createFloatBuffer(vert)); m.setBuffer(Type.Normal, 3, BufferUtils.createFloatBuffer(norm)); m.setBuffer(Type.TexCoord, 2, BufferUtils.createFloatBuffer(texCoord)); m.setBuffer(Type.Index, 1, BufferUtils.createIntBuffer(indexes)); m.updateBound(); // *** 5. End // *** 6. Start (Geometry, Material, Texture) Geometry geom = new Geometry("DiceMesh", m); Material mat = new Material(assetManager, "Common/MatDefs/Light/Lighting.j3md"); Texture matTex = assetManager.loadTexture( "Textures/dice.png"); mat.setTexture("DiffuseMap", matTex); mat.setColor("Diffuse",ColorRGBA.Red); mat.setColor("Specular",ColorRGBA.White); mat.setFloat("Shininess", 32f); // [0,128] geom.setMaterial(mat); rootNode.attachChild(geom); // *** 6. End // *** 7. Start (Lights, Drag from Palette) /** A white, directional light source */ DirectionalLight sun = new DirectionalLight(); sun.setDirection((new Vector3f(-0.5f, -0.5f, -0.5f)).normalizeLocal()); sun.setColor(ColorRGBA.White); rootNode.addLight(sun); /** A white ambient light source. */ /** A cone-shaped spotlight with location, direction, range */ SpotLight spot = new SpotLight(); spot = new SpotLight(); spot.setSpotRange(100); spot.setSpotOuterAngle(20 * FastMath.DEG_TO_RAD); spot.setSpotInnerAngle(15 * FastMath.DEG_TO_RAD); spot.setDirection(cam.getDirection()); spot.setPosition(cam.getLocation()); rootNode.addLight(spot); AmbientLight ambient = new AmbientLight(); ambient.setColor(ColorRGBA.Red); rootNode.addLight(ambient); // *** 7. End } @Override public void simpleUpdate(float tpf) { // Rotation per frame rootNode.rotate(.005f,.01f,.008f); } }
Output:
No comments:
Post a Comment