The use of keys is important in testing an application. They also can be important in desktop applications.
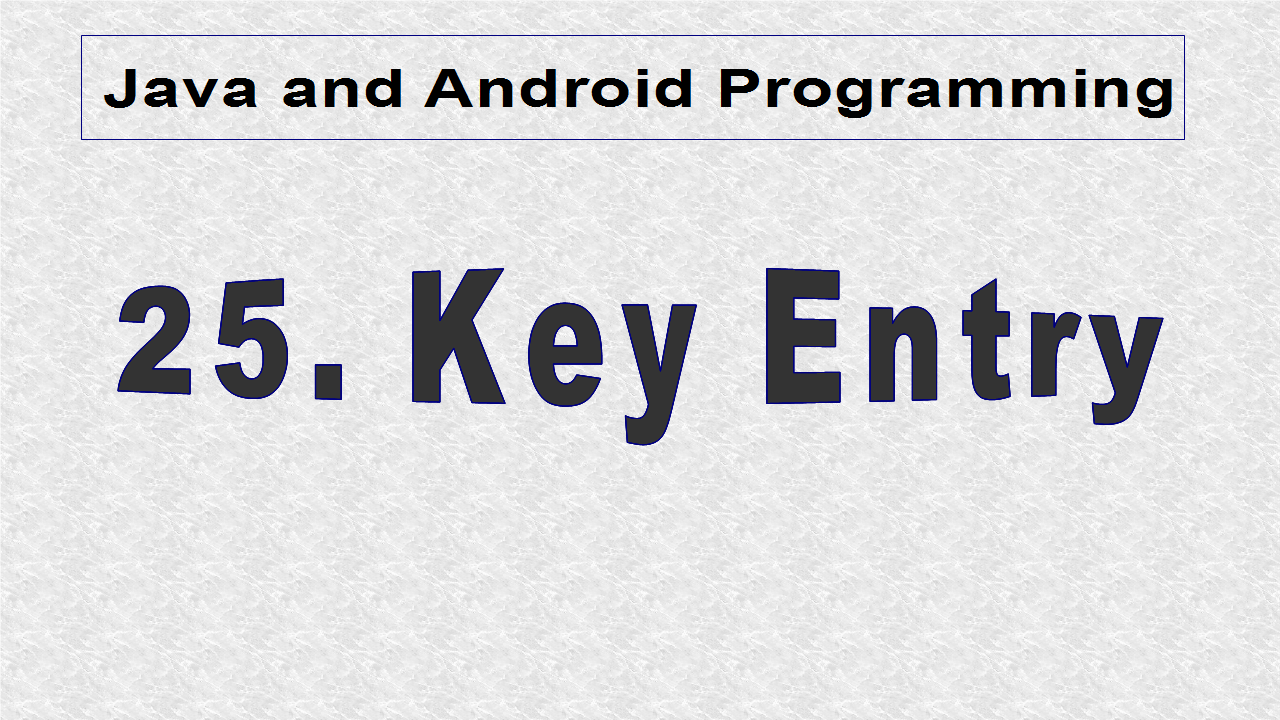
Here 12 keys are mapped. In addition some keys have default meanings such as those for the default camera. 6 keys are for position and 6 keys are for angles. The keys were selected because they are near each other in the physical keyboard.
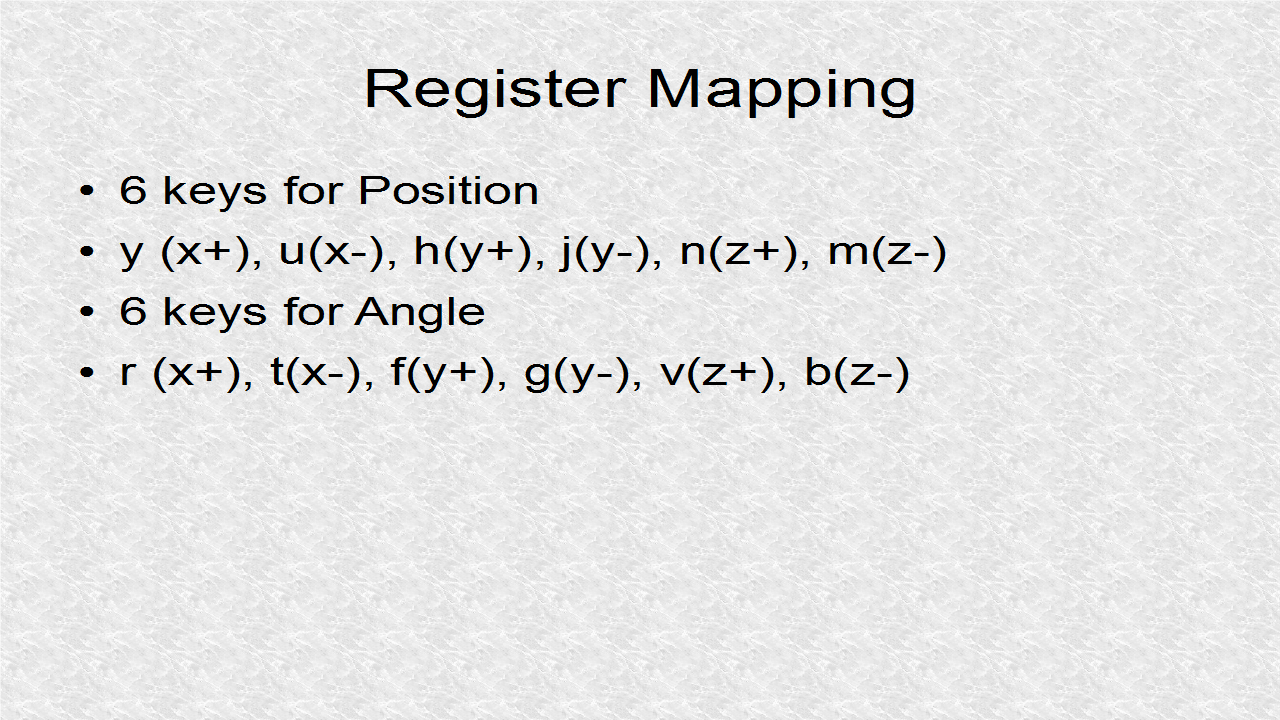
// *** 1. Start (Register Mappings) inputManager.addMapping("XP", new KeyTrigger(KeyInput.KEY_Y)); // x+ inputManager.addMapping("XM", new KeyTrigger(KeyInput.KEY_U)); // x- inputManager.addMapping("YP", new KeyTrigger(KeyInput.KEY_H)); // y+ inputManager.addMapping("YM", new KeyTrigger(KeyInput.KEY_J)); // y- inputManager.addMapping("ZP", new KeyTrigger(KeyInput.KEY_N)); // z+ inputManager.addMapping("ZM", new KeyTrigger(KeyInput.KEY_M)); // z- inputManager.addMapping("AXP", new KeyTrigger(KeyInput.KEY_R)); // ang x+ inputManager.addMapping("AXM", new KeyTrigger(KeyInput.KEY_T)); // ang x- inputManager.addMapping("AYP", new KeyTrigger(KeyInput.KEY_F)); // ang y+ inputManager.addMapping("AYM", new KeyTrigger(KeyInput.KEY_G)); // ang y- inputManager.addMapping("AZP", new KeyTrigger(KeyInput.KEY_V)); // ang z+ inputManager.addMapping("AZM", new KeyTrigger(KeyInput.KEY_B)); // ang z- // *** 1. End
We have to add listeners for the 12 keys.
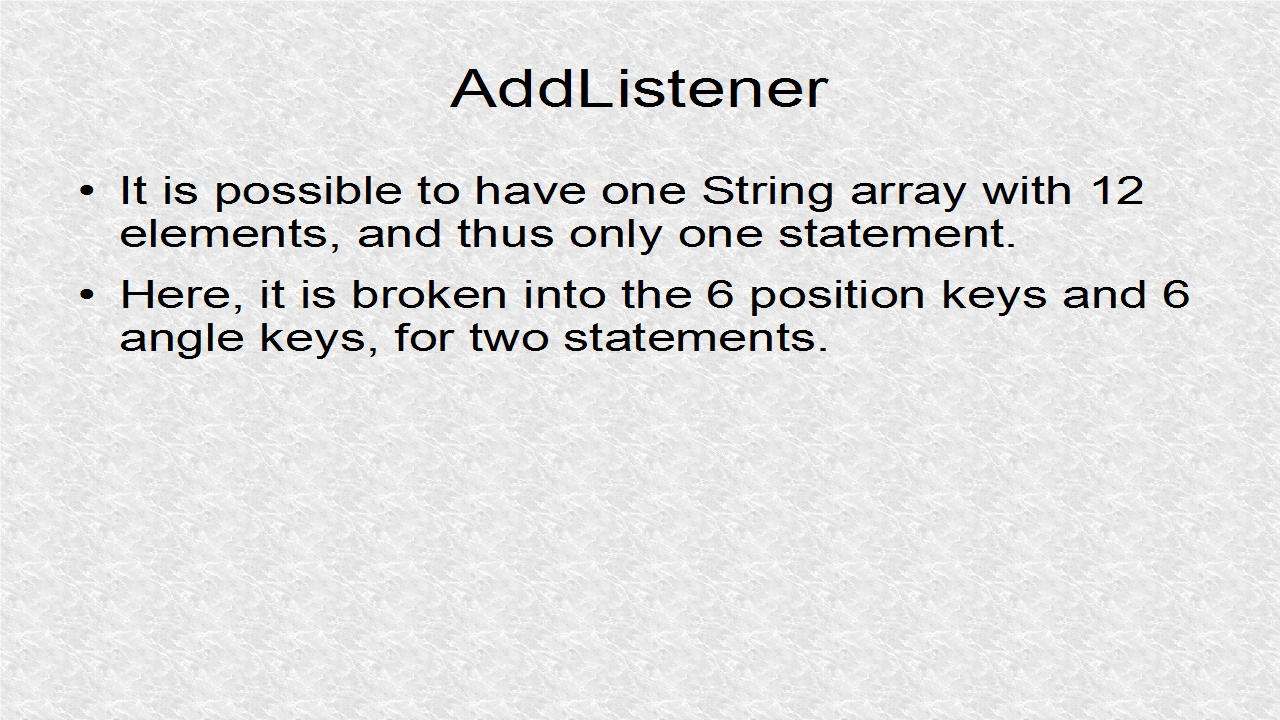
// *** 2. Start (Add Listeners) inputManager.addListener(analogListener, new String[]{"XP","XM","YP","YM","ZP","ZM"}); // pos inputManager.addListener(analogListener, new String[]{"AXP","AXM","AYP","AYM","AZP","AZM"}); // ang // *** 2. End
A monkey mesh, of Suzanne, is exported from Blender.
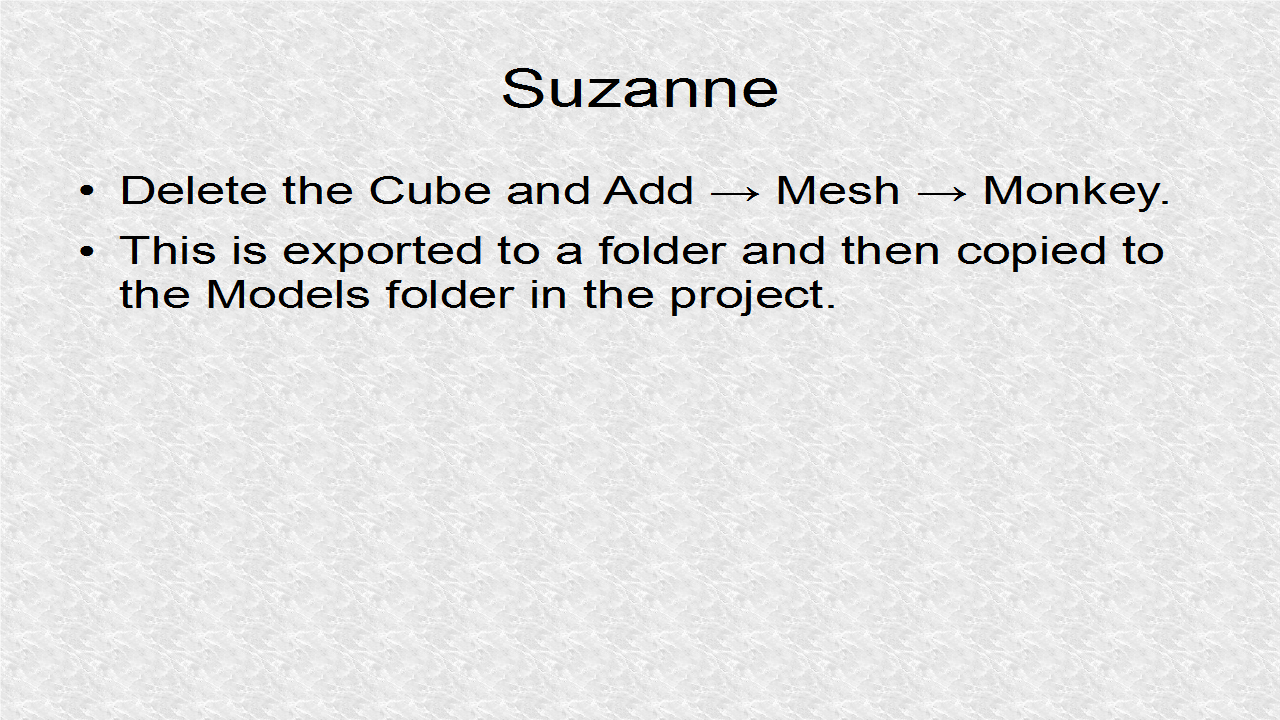
// *** 3. Start (Suzanne from Blender) geom = assetManager.loadModel("Models/Suzanne.mesh.xml"); // *** 3. End
The material is color blue.
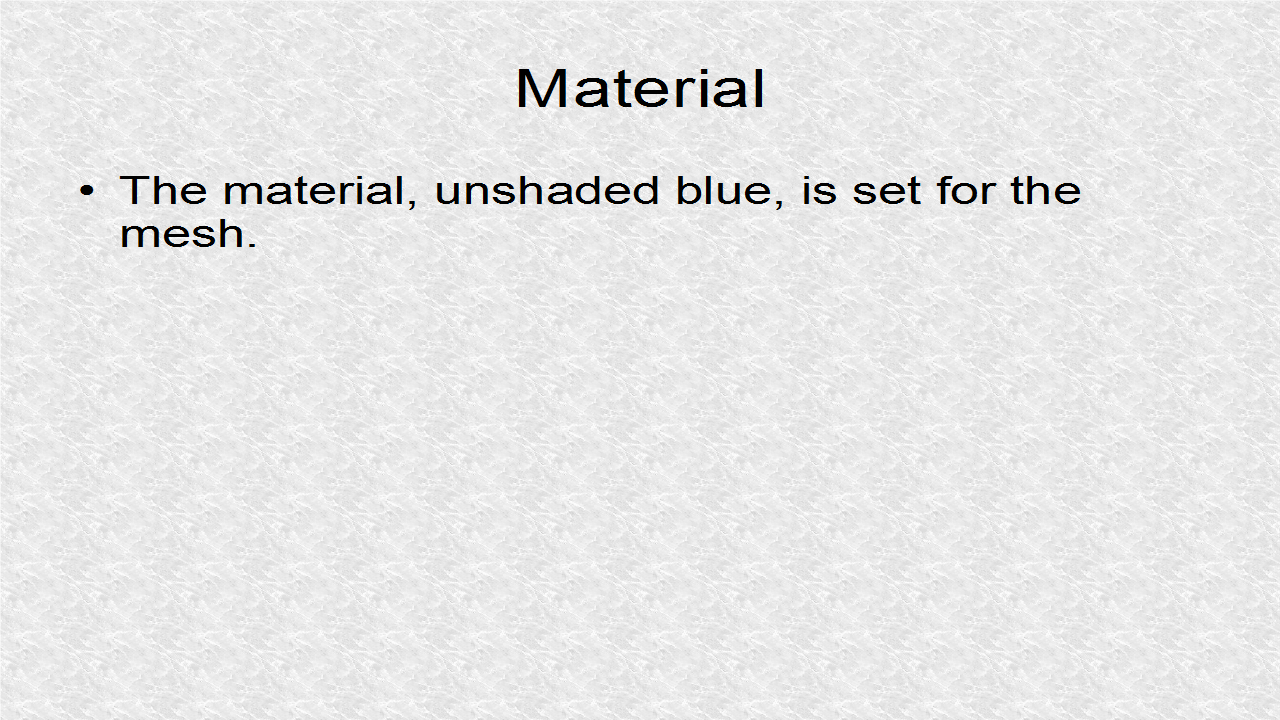
// *** 4. Start (Unshaded Blue) Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat.setColor("Color", ColorRGBA.Blue); geom.setMaterial(mat); // *** 4. End
An if and eleven else if's are used to test for the 12 triggers.
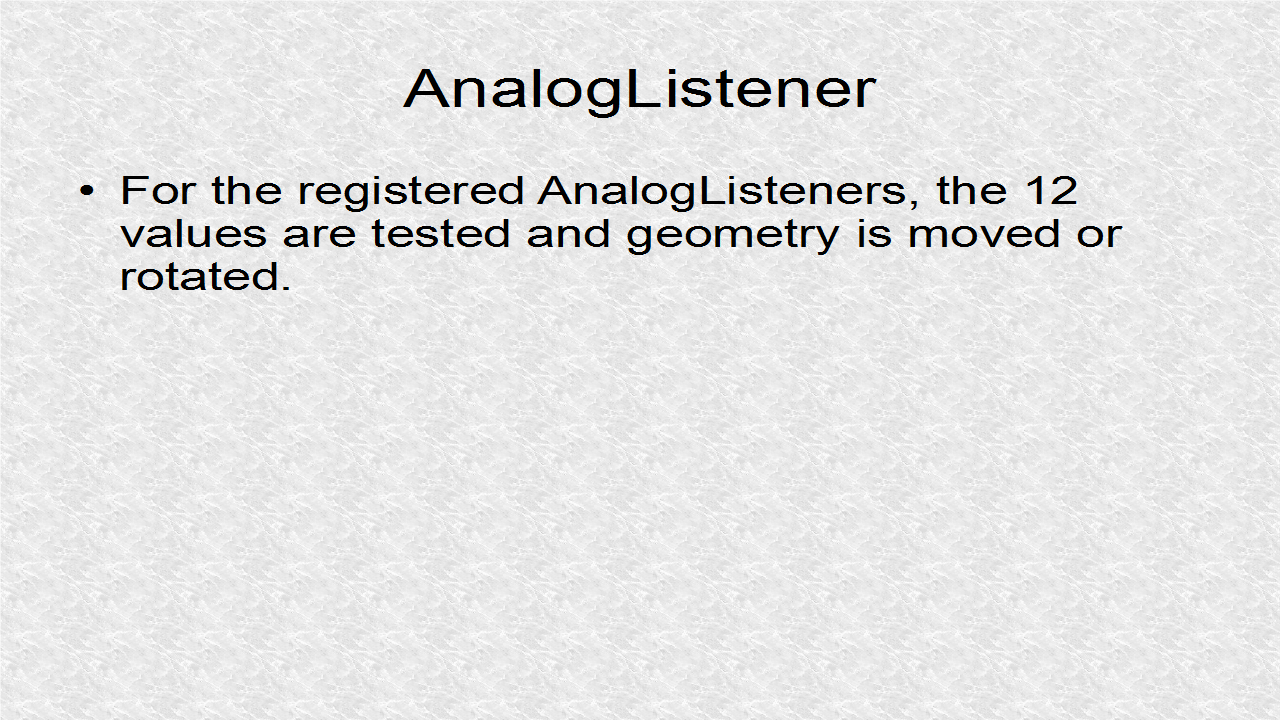
// *** 5. Start (AnalogListener) private AnalogListener analogListener = new AnalogListener() { public void onAnalog(String name, float intensity, float tpf) { if (name.equals("XP")) { geom.move(.01f, 0, 0); } else if (name.equals("XM")) { geom.move(-.01f, 0, 0); } else if (name.equals("YM")) { geom.move(0, -.01f, 0); } else if (name.equals("YP")) { geom.move(0, .01f, 0); } else if (name.equals("ZP")) { geom.move(0, 0, .01f); } else if (name.equals("ZM")) { geom.move(0, 0, -.01f); } else if (name.equals("AXP")) { geom.rotate(ANG,0,0); } else if (name.equals("AXM")) { geom.rotate(-ANG,0,0); } else if (name.equals("AYP")) { geom.rotate(0,ANG,0); } else if (name.equals("AYM")) { geom.rotate(0,-ANG,0); } else if (name.equals("AZP")) { geom.rotate(0,0,ANG); } else if (name.equals("AZM")) { geom.rotate(0,0,-ANG); } } }; // *** 5. End
package mygame; import com.jme3.app.SimpleApplication; import com.jme3.input.KeyInput; import com.jme3.input.controls.AnalogListener; import com.jme3.input.controls.KeyTrigger; import com.jme3.input.controls.Trigger; import com.jme3.material.Material; import com.jme3.math.ColorRGBA; import com.jme3.math.FastMath; import com.jme3.renderer.RenderManager; import com.jme3.scene.Spatial; public class JMonkey4 extends SimpleApplication { Spatial geom; final float ANG = 0.05f*FastMath.DEG_TO_RAD; public static void main(String[] args) { JMonkey4 app = new JMonkey4(); app.start(); } @Override public void simpleInitApp() { // *** 1. Start (Register Mappings) inputManager.addMapping("XP", new KeyTrigger(KeyInput.KEY_Y)); // x+ inputManager.addMapping("XM", new KeyTrigger(KeyInput.KEY_U)); // x- inputManager.addMapping("YP", new KeyTrigger(KeyInput.KEY_H)); // y+ inputManager.addMapping("YM", new KeyTrigger(KeyInput.KEY_J)); // y- inputManager.addMapping("ZP", new KeyTrigger(KeyInput.KEY_N)); // z+ inputManager.addMapping("ZM", new KeyTrigger(KeyInput.KEY_M)); // z- inputManager.addMapping("AXP", new KeyTrigger(KeyInput.KEY_R)); // ang x+ inputManager.addMapping("AXM", new KeyTrigger(KeyInput.KEY_T)); // ang x- inputManager.addMapping("AYP", new KeyTrigger(KeyInput.KEY_F)); // ang y+ inputManager.addMapping("AYM", new KeyTrigger(KeyInput.KEY_G)); // ang y- inputManager.addMapping("AZP", new KeyTrigger(KeyInput.KEY_V)); // ang z+ inputManager.addMapping("AZM", new KeyTrigger(KeyInput.KEY_B)); // ang z- // *** 1. End // *** 2. Start (Add Listeners) inputManager.addListener(analogListener, new String[]{"XP","XM","YP","YM","ZP","ZM"}); // pos inputManager.addListener(analogListener, new String[]{"AXP","AXM","AYP","AYM","AZP","AZM"}); // ang // *** 2. End // *** 3. Start (Suzanne from Blender) geom = assetManager.loadModel("Models/Suzanne.mesh.xml"); // *** 3. End // *** 4. Start (Unshaded Blue) Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat.setColor("Color", ColorRGBA.Blue); geom.setMaterial(mat); // *** 4. End rootNode.attachChild(geom); } // *** 5. Start (AnalogListener) private AnalogListener analogListener = new AnalogListener() { public void onAnalog(String name, float intensity, float tpf) { if (name.equals("XP")) { geom.move(.01f, 0, 0); } else if (name.equals("XM")) { geom.move(-.01f, 0, 0); } else if (name.equals("YM")) { geom.move(0, -.01f, 0); } else if (name.equals("YP")) { geom.move(0, .01f, 0); } else if (name.equals("ZP")) { geom.move(0, 0, .01f); } else if (name.equals("ZM")) { geom.move(0, 0, -.01f); } else if (name.equals("AXP")) { geom.rotate(ANG,0,0); } else if (name.equals("AXM")) { geom.rotate(-ANG,0,0); } else if (name.equals("AYP")) { geom.rotate(0,ANG,0); } else if (name.equals("AYM")) { geom.rotate(0,-ANG,0); } else if (name.equals("AZP")) { geom.rotate(0,0,ANG); } else if (name.equals("AZM")) { geom.rotate(0,0,-ANG); } } }; // *** 5. End @Override public void simpleUpdate(float tpf) { //TODO: add update code } @Override public void simpleRender(RenderManager rm) { //TODO: add render code } }
Output:
As x position change, moves left and right
As y position changes, move bottom to top.
As z position changes, moves towards and away. (Seems bigger or smaller)
Then the 6 angle keys are tested. First x, then y, and finally in z.
No comments:
Post a Comment