A Cylinder is one of the built-in mesh shapes.
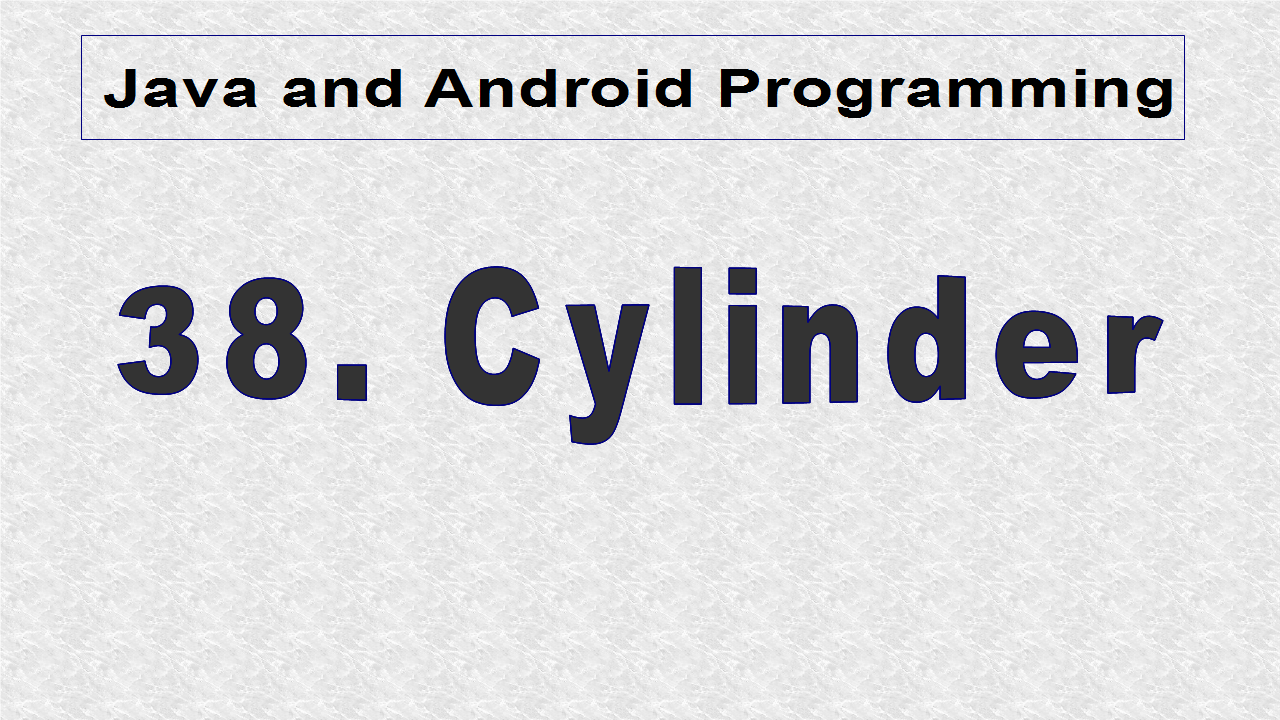
We can find number of vertices and their coordinates.
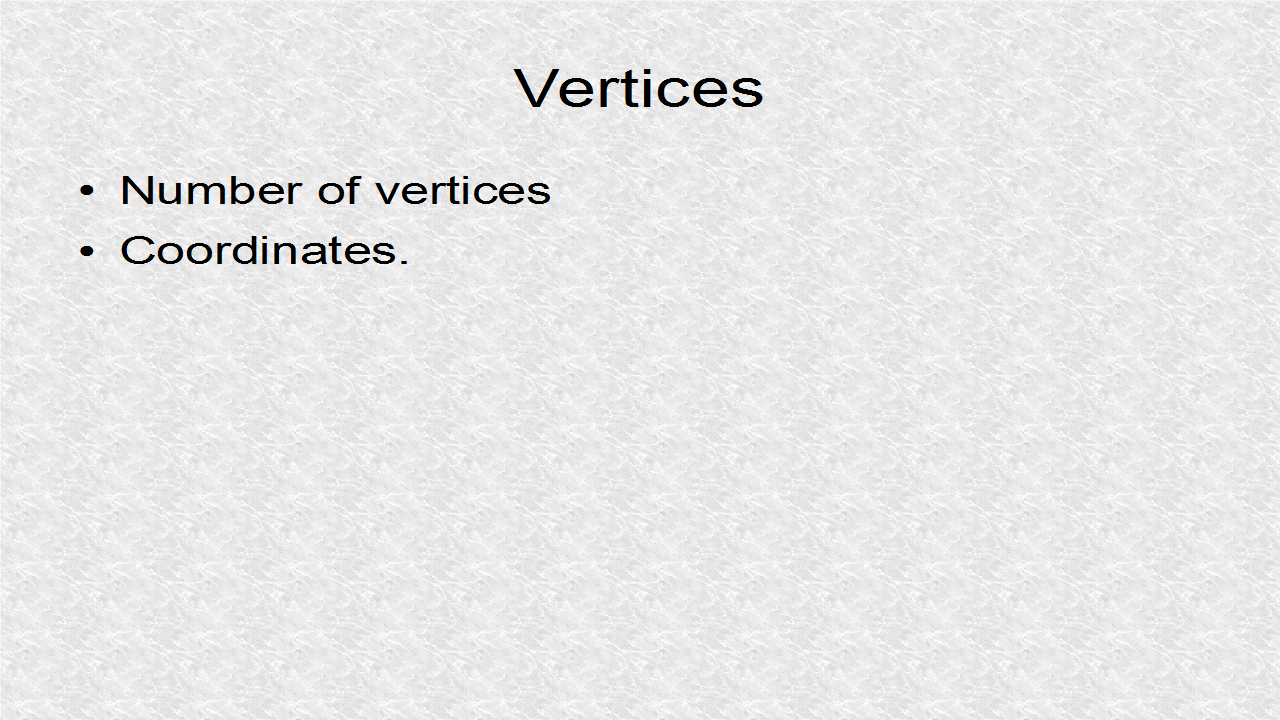
// *** 2. Start (Vertices) VertexBuffer position = cylinder. getBuffer(Type.Position); Vector3f[] array = BufferUtils. getVector3Array((FloatBuffer)position. getData()); System.out.println("cylinder has " + array.length + " vertices. Coordinates are: "); int i = 0; for (Vector3f v : array) { System.out.printf("%d\t%s\n",i++,v); } // *** 2. End
This materials tests if the mesh structure is correctly loaded.
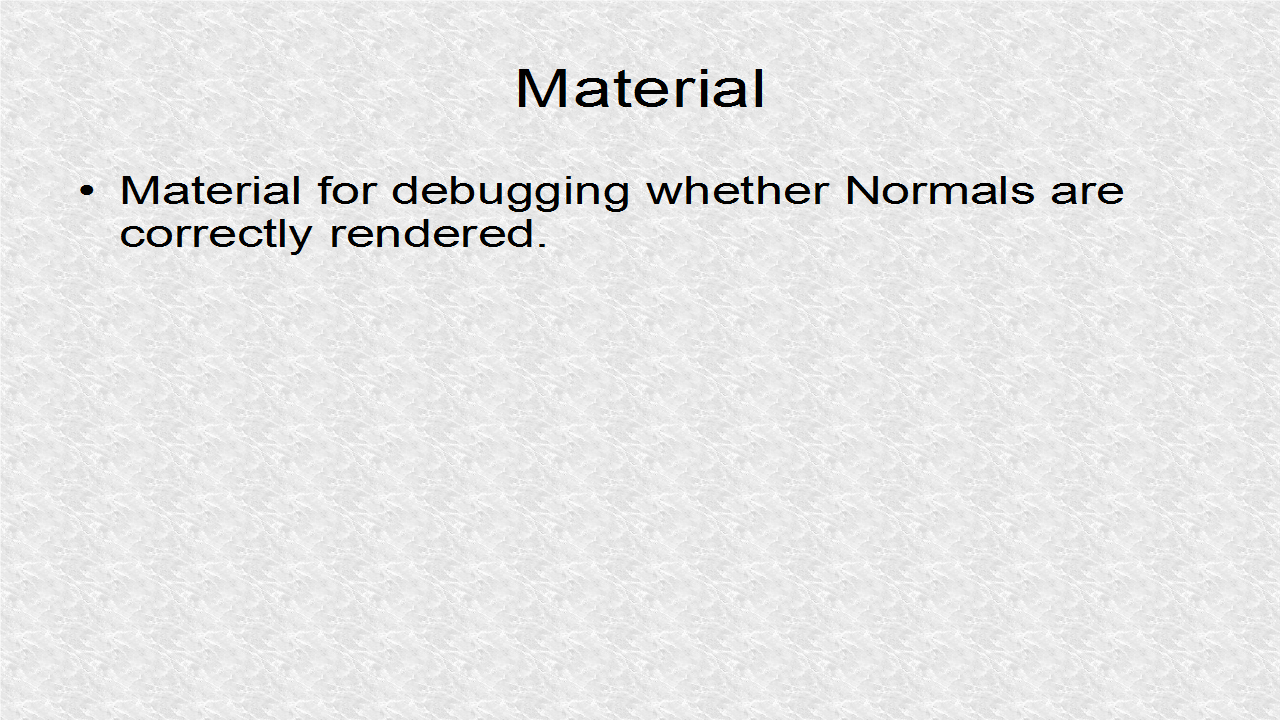
// *** 3. Start (Material) Material mat = new Material(assetManager, "Common/MatDefs/Misc/ShowNormals.j3md"); geoCylinder.setMaterial(mat); // *** 3. End
// *** 4. Start (Rotation per frame) rootNode.rotate(.5f*tpf, .1f*tpf, .2f*tpf); // *** 4. End
// JMonkey38.java package mygame; import com.jme3.app.SimpleApplication; import com.jme3.material.Material; import com.jme3.math.ColorRGBA; import com.jme3.math.Vector3f; import com.jme3.renderer.RenderManager; import com.jme3.scene.Geometry; import com.jme3.scene.VertexBuffer; import com.jme3.scene.VertexBuffer.Type; import com.jme3.scene.shape.Cylinder; import com.jme3.system.AppSettings; import com.jme3.util.BufferUtils; import java.nio.FloatBuffer; public class JMonkey38 extends SimpleApplication { public static void main(String[] args) { AppSettings settings = new AppSettings(true); settings.setFrameRate(57); settings.setTitle("Cylinder !"); JMonkey38 app = new JMonkey38(); app.setSettings(settings); app.start(); } @Override public void simpleInitApp() { viewPort.setBackgroundColor( new ColorRGBA(1f,.9f,.8f,1)); setDisplayFps(false); setDisplayStatView(false); flyCam.setEnabled(false); // *** 1. Start (cylinder mesh) Cylinder cylinder = new Cylinder(8,8,1.5f,2.5f,true); Geometry geoCylinder = new Geometry("cylinder", cylinder); // *** 2. Start (Vertices) VertexBuffer position = cylinder. getBuffer(Type.Position); Vector3f[] array = BufferUtils. getVector3Array((FloatBuffer)position. getData()); System.out.println("cylinder has " + array.length + " vertices. Coordinates are: "); int i = 0; for (Vector3f v : array) { System.out.printf("%d\t%s\n",i++,v); } // *** 2. End // *** 3. Start (Material) Material mat = new Material(assetManager, "Common/MatDefs/Misc/ShowNormals.j3md"); geoCylinder.setMaterial(mat); // *** 3. End rootNode.attachChild(geoCylinder); } @Override public void simpleUpdate(float tpf) { // *** 4. Start (Rotation per frame) rootNode.rotate(.5f*tpf, .1f*tpf, .2f*tpf); // *** 4. End } @Override public void simpleRender(RenderManager rm) { } }
Output:
No comments:
Post a Comment