We can apply texture images to materials.
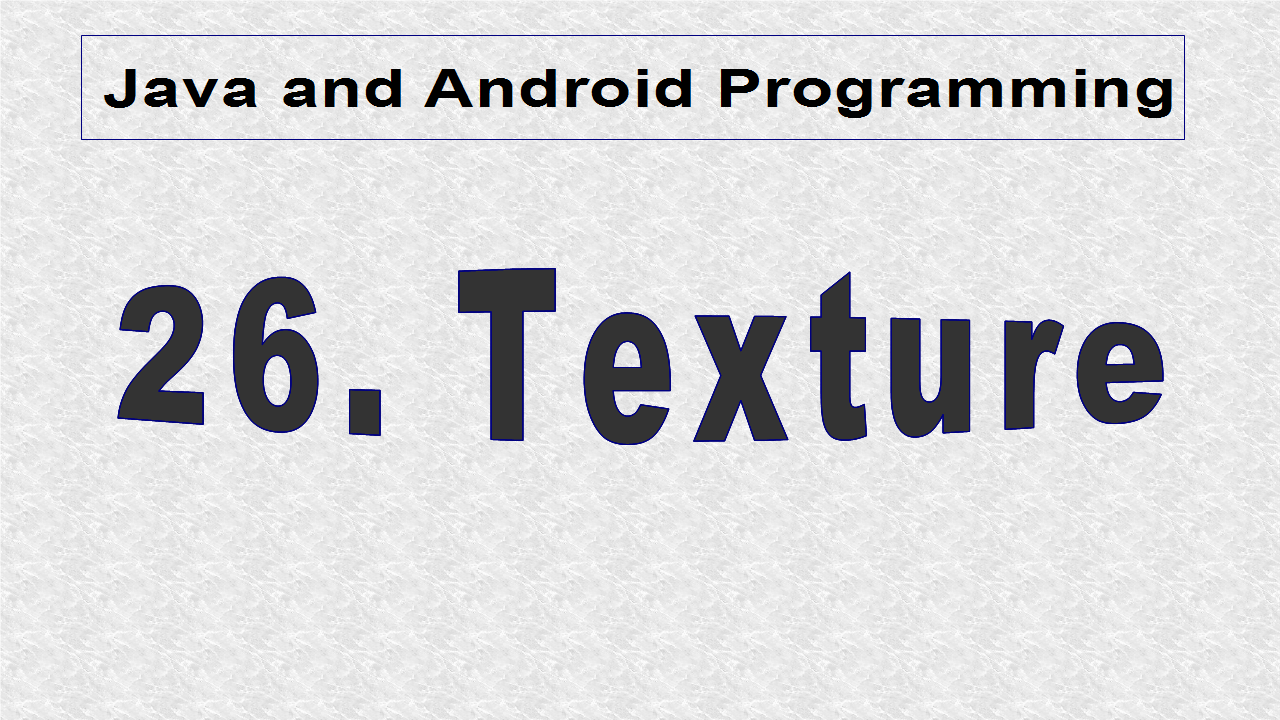
First some application settings are changed, such as the title. We also disable the display of stats and fps. We had earlier used a different method.
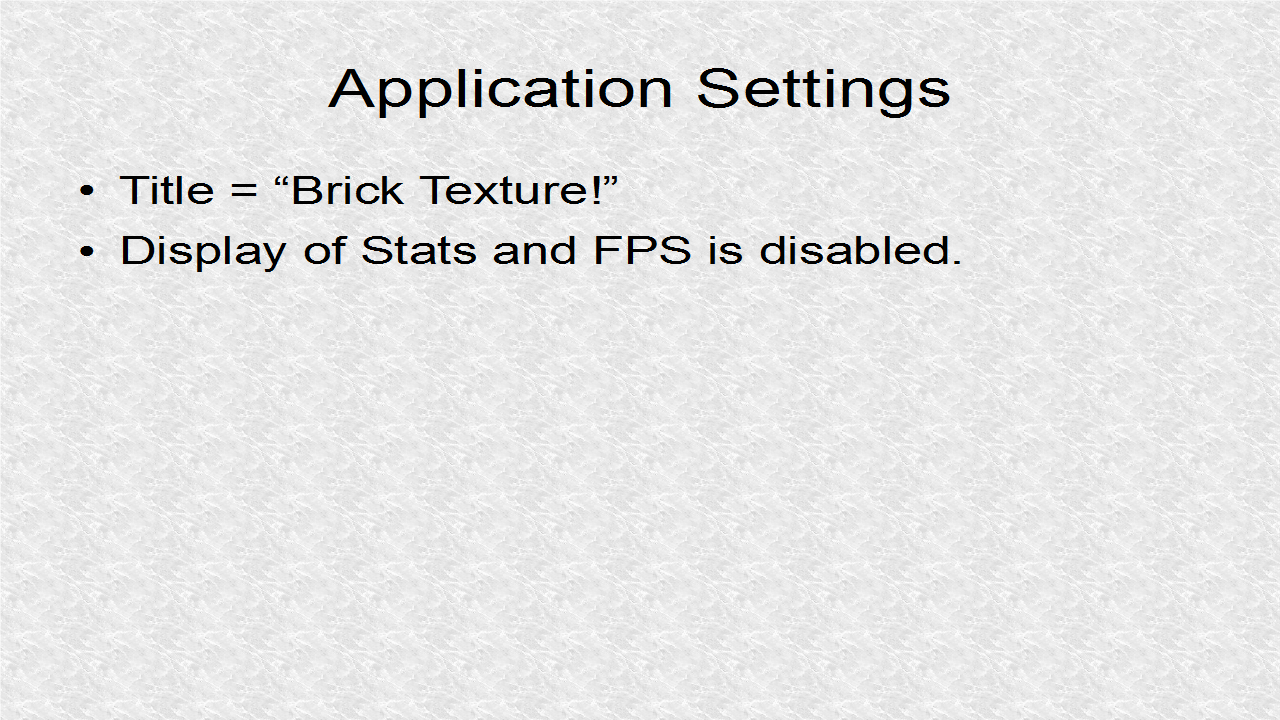
// *** 1. Start (Application settings, start) AppSettings setting= new AppSettings(true); setting.setTitle("Brick Texture!"); JMonkey26 app = new JMonkey26(); app.setDisplayFps(false); app.setDisplayStatView(false); app.setSettings(setting); app.start(); // *** 1. End
The background is set as white. A box of dimension 2 by 2 by 2 is created. The material is unshaded. It next obtains a texture.
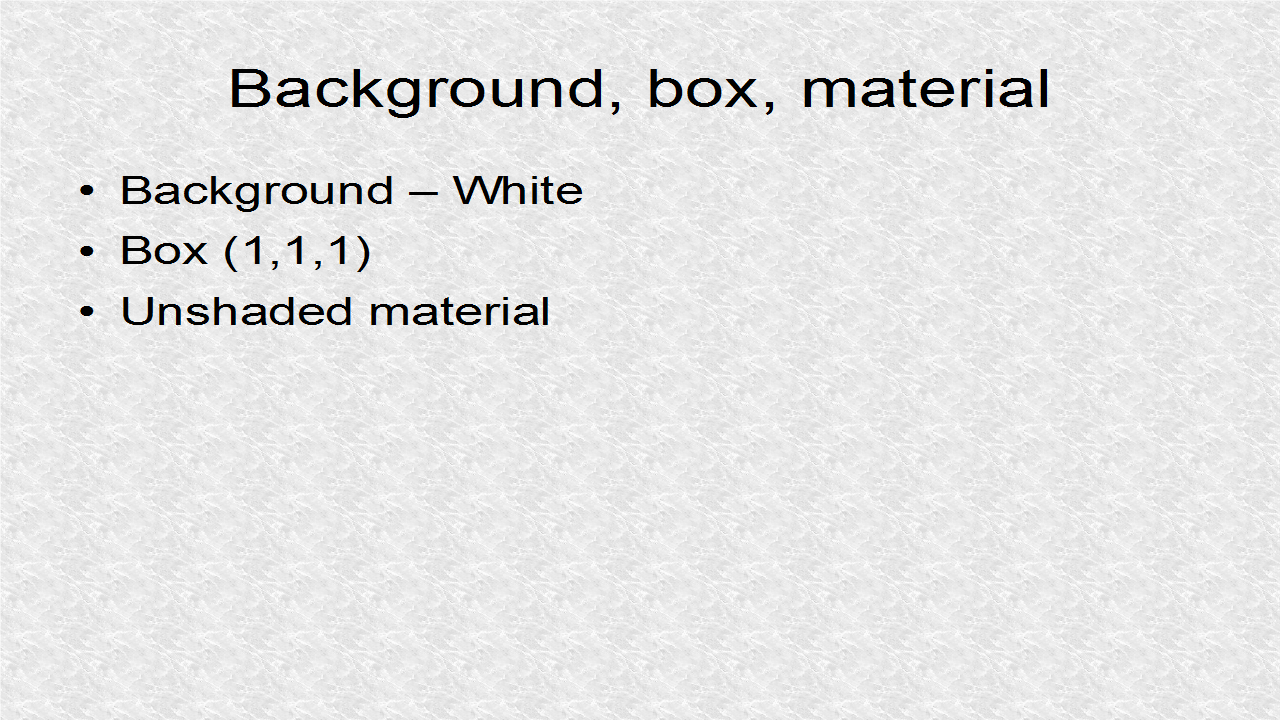
// *** 2. Start (Background, box, material) viewPort.setBackgroundColor(ColorRGBA.White); Box b = new Box(1, 1, 1); Geometry geom = new Geometry("Box", b); Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); // *** 2. End
The texture provides a color map so it is not a single color. The file 'brick.jpg' is copied to the Interface folder in the project.
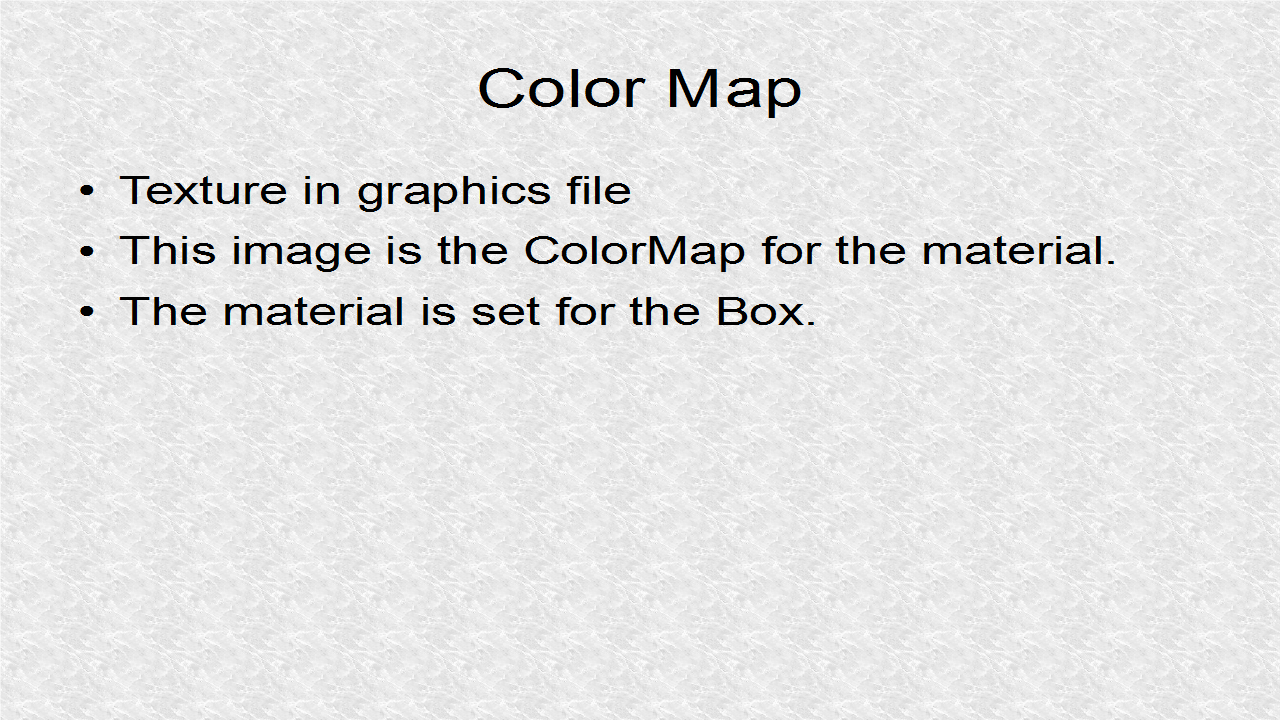
// *** 3. Start (Texture) Texture tex = assetManager.loadTexture("Interface/brick.jpg"); mat.selectTechnique("Default", renderManager); mat.setColor("Color", ColorRGBA.White); mat.setTexture("ColorMap", tex); geom.setMaterial(mat); // *** 3. End
In the simpleUpdate, which is run for every frame, the rotation angle is slightly changed, randomly in either x, y, or z direction.
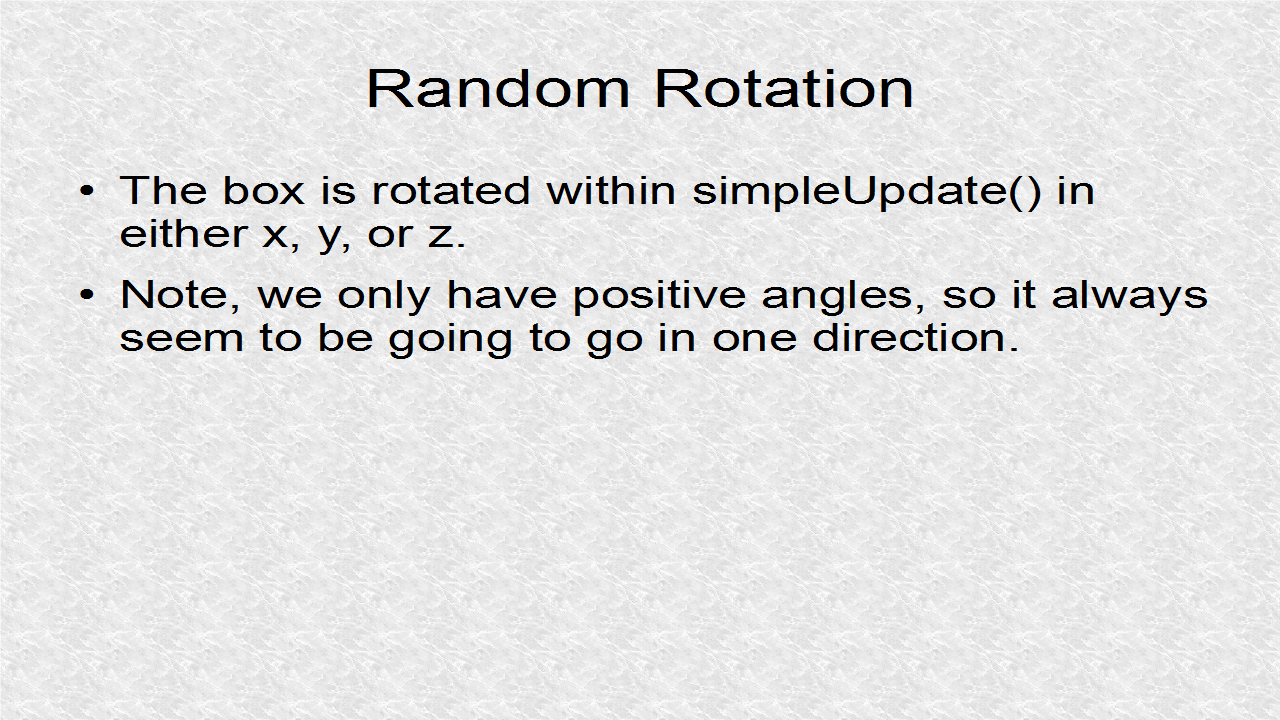
// *** 4. Start (Random Rotate) switch (FastMath.nextRandomInt(0, 2)) { case 0: rootNode.rotate(30*tpf*FastMath.DEG_TO_RAD,0,0); break; case 1: rootNode.rotate(0, 30*tpf*FastMath.DEG_TO_RAD, 0); break; case 2: rootNode.rotate(0,0,30*tpf*FastMath.DEG_TO_RAD); break; } // *** 4. End
// JMonkey26.java package mygame; import com.jme3.app.SimpleApplication; import com.jme3.material.Material; import com.jme3.math.ColorRGBA; import com.jme3.math.FastMath; import com.jme3.renderer.RenderManager; import com.jme3.scene.Geometry; import com.jme3.scene.shape.Box; import com.jme3.system.AppSettings; import com.jme3.texture.Texture; public class JMonkey26 extends SimpleApplication { public static void main(String[] args) { // *** 1. Start (Application settings, start) AppSettings setting= new AppSettings(true); setting.setTitle("Brick Texture!"); JMonkey26 app = new JMonkey26(); app.setDisplayFps(false); app.setDisplayStatView(false); app.setSettings(setting); app.start(); // *** 1. End } @Override public void simpleInitApp() { flyCam.setEnabled(false); // *** 2. Start (Background, box, material) viewPort.setBackgroundColor(ColorRGBA.White); Box b = new Box(1, 1, 1); Geometry geom = new Geometry("Box", b); Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); // *** 2. End // *** 3. Start (Texture) Texture tex = assetManager.loadTexture("Interface/brick.jpg"); mat.selectTechnique("Default", renderManager); mat.setColor("Color", ColorRGBA.White); mat.setTexture("ColorMap", tex); geom.setMaterial(mat); // *** 3. End rootNode.attachChild(geom); } @Override public void simpleUpdate(float tpf) { // *** 4. Start (Random Rotate) switch (FastMath.nextRandomInt(0, 2)) { case 0: rootNode.rotate(30*tpf*FastMath.DEG_TO_RAD,0,0); break; case 1: rootNode.rotate(0, 30*tpf*FastMath.DEG_TO_RAD, 0); break; case 2: rootNode.rotate(0,0,30*tpf*FastMath.DEG_TO_RAD); break; } // *** 4. End } @Override public void simpleRender(RenderManager rm) { //TODO: add render code } }
Output:
No comments:
Post a Comment