We will use the icosphere mesh created in Blender.
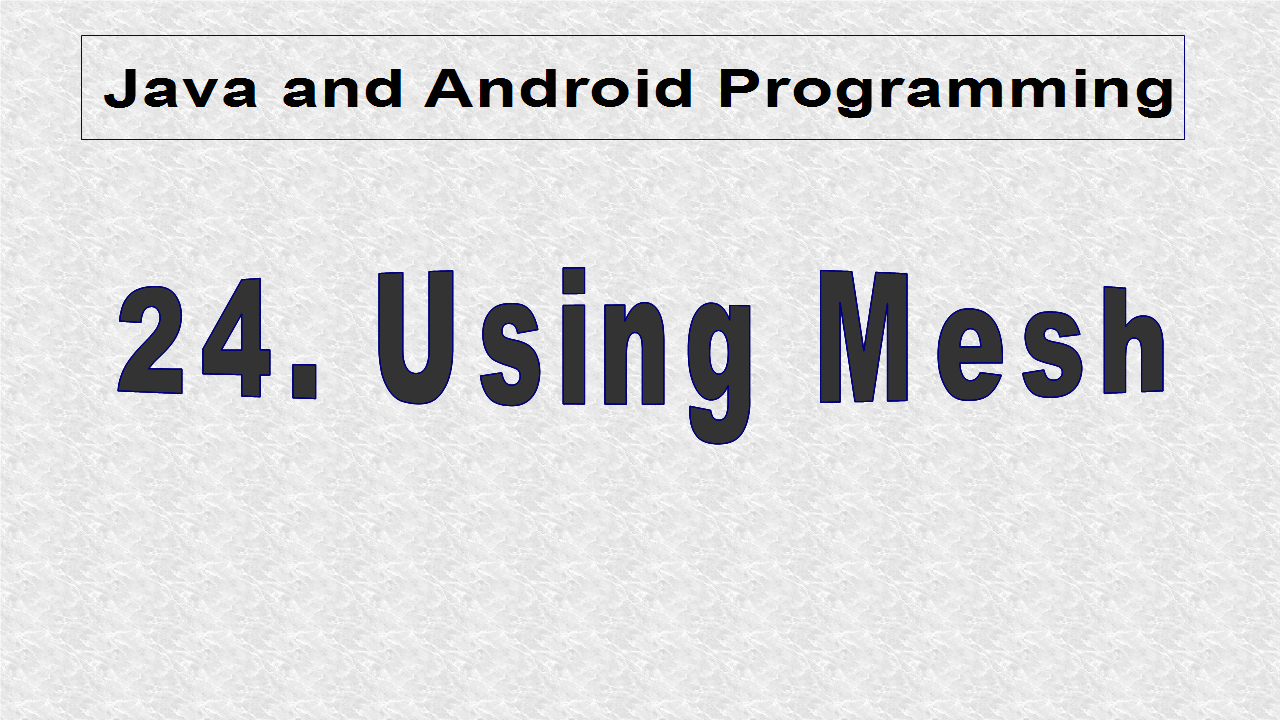
First we have to copy the file to the Models folder of our project.
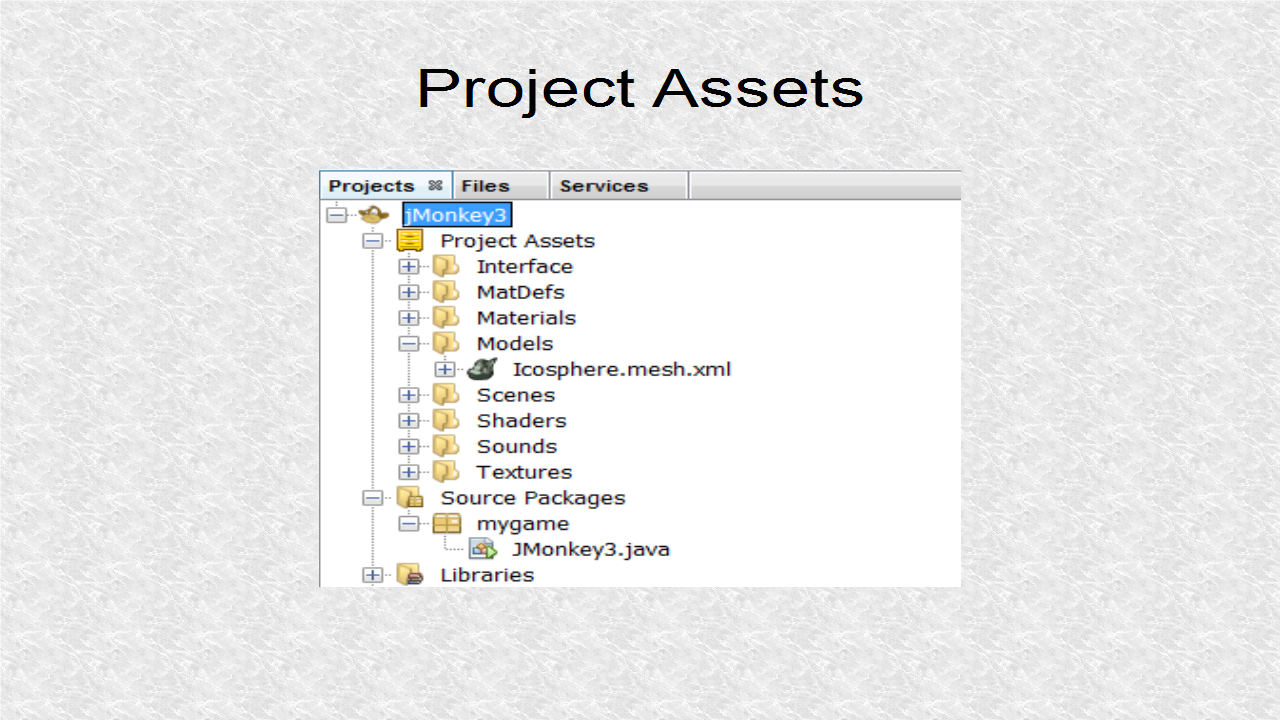
We will set a background color of yellow.
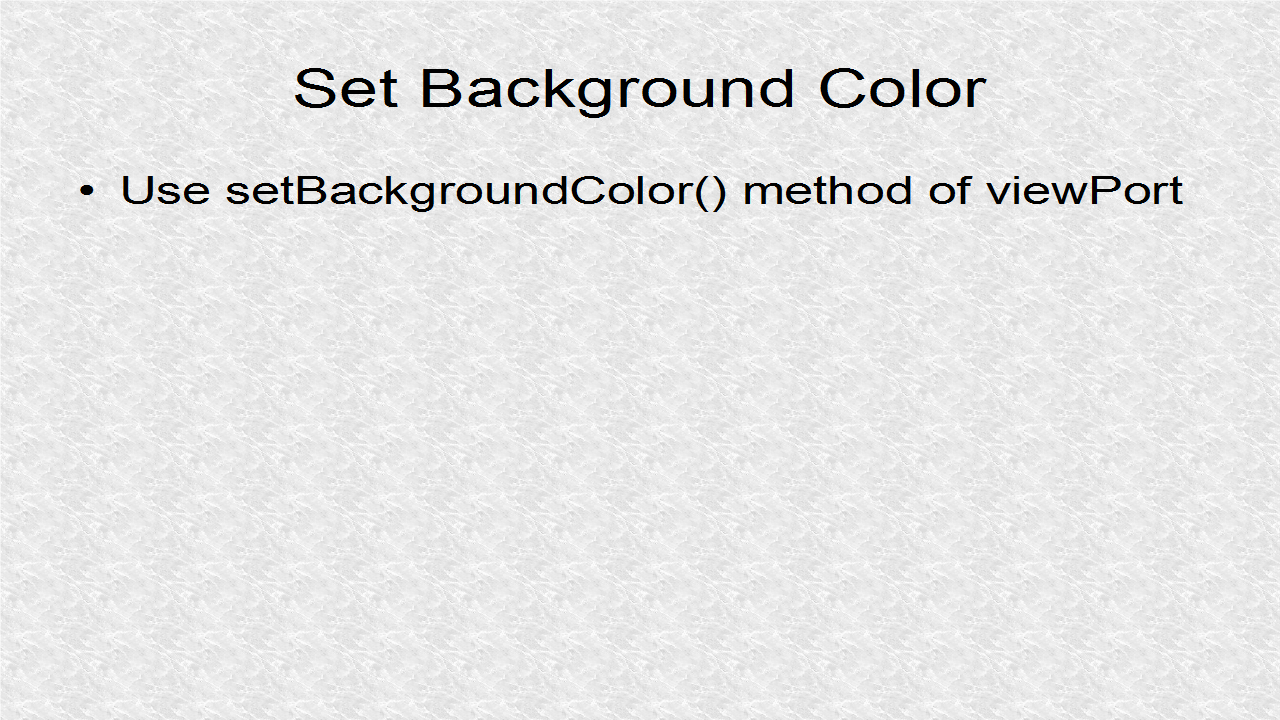
// *** 1. Start (Set background color as yellow) viewPort.setBackgroundColor(ColorRGBA.Yellow); // *** 1. End
We show the 3 axes. Note, they are at a fixed place in 3D space, and we will not move them. However, they will rotate along the icosphere since they are all attached to rootNode.
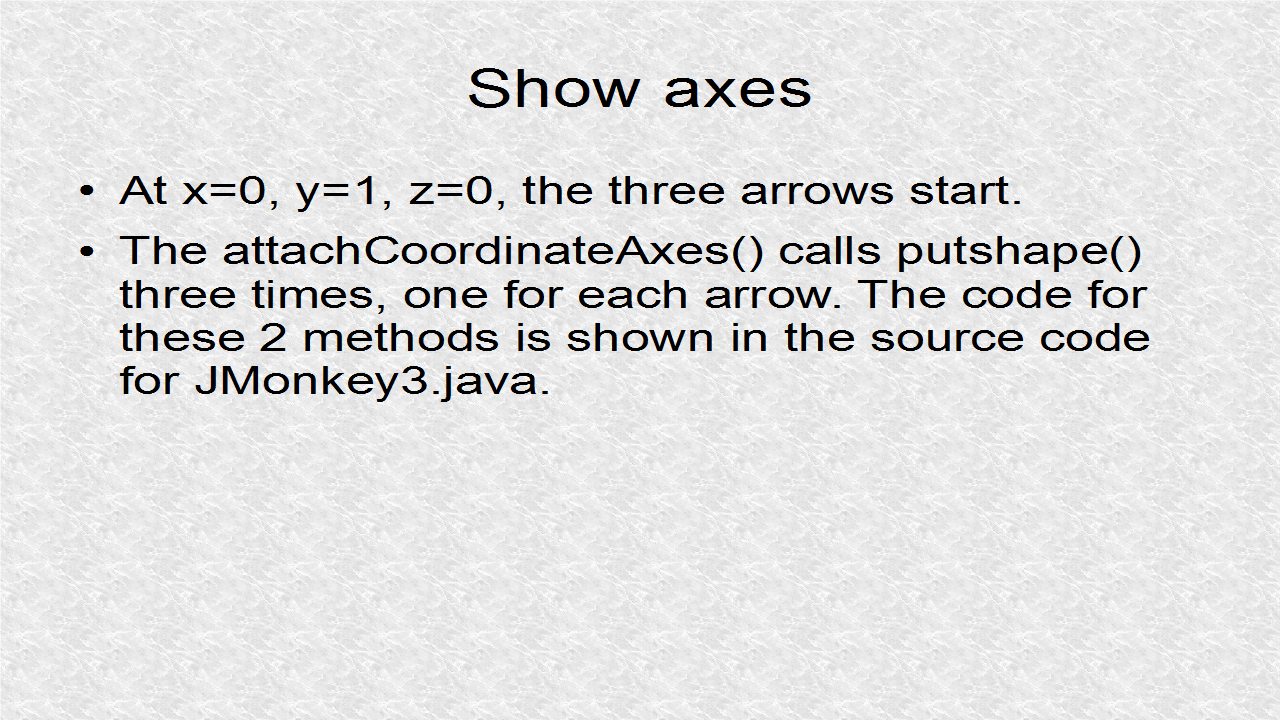
// *** 2. Start (Show coordinates, red x, green y, blue z) attachCoordinateAxes(new Vector3f(0,1,0)); // *** 2. End
We then load the icosphere mesh to a Spatial object.
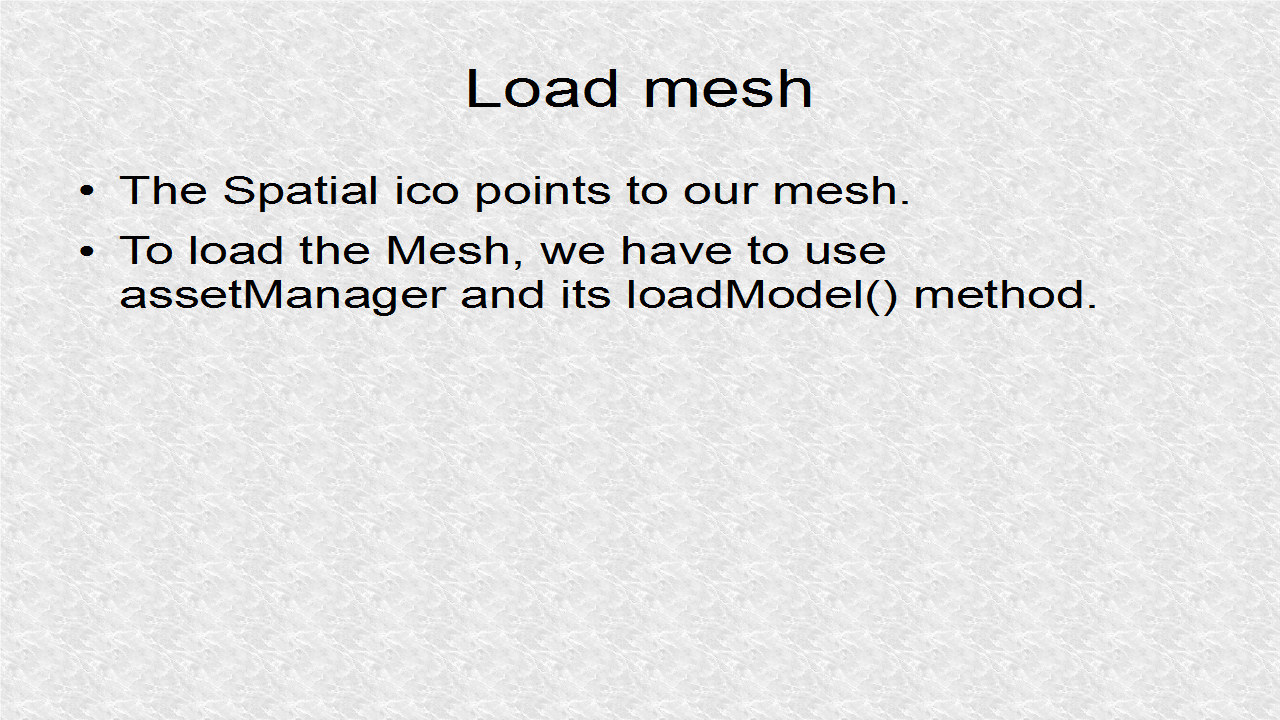
// *** 3. Start (Load Icoshpere --> ico) Spatial ico = assetManager.loadModel("Models/Icosphere.mesh.xml"); // *** 3. End
The material, applied to the Spatial object, is an unshaded Pink.
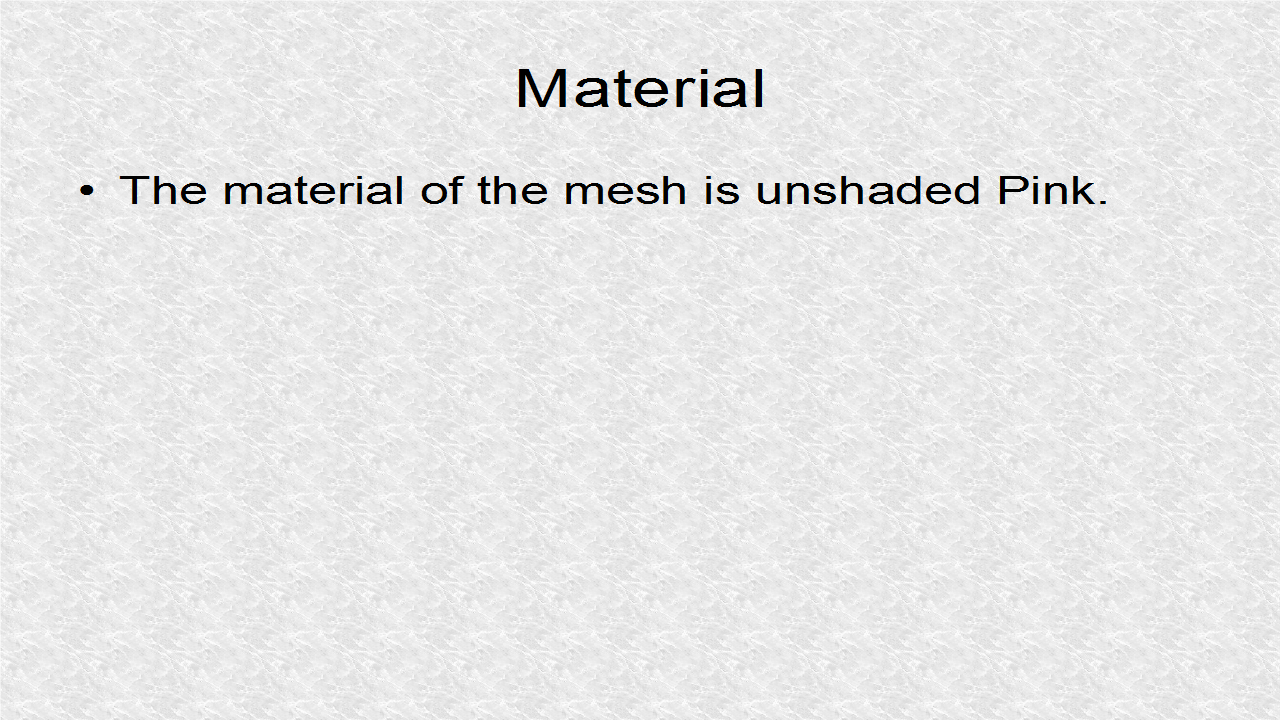
// *** 4. Start (Set ico to pink color) Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat.setColor("Color", ColorRGBA.Pink); ico.setMaterial(mat); // *** 4. End
We attach it to rootNode.
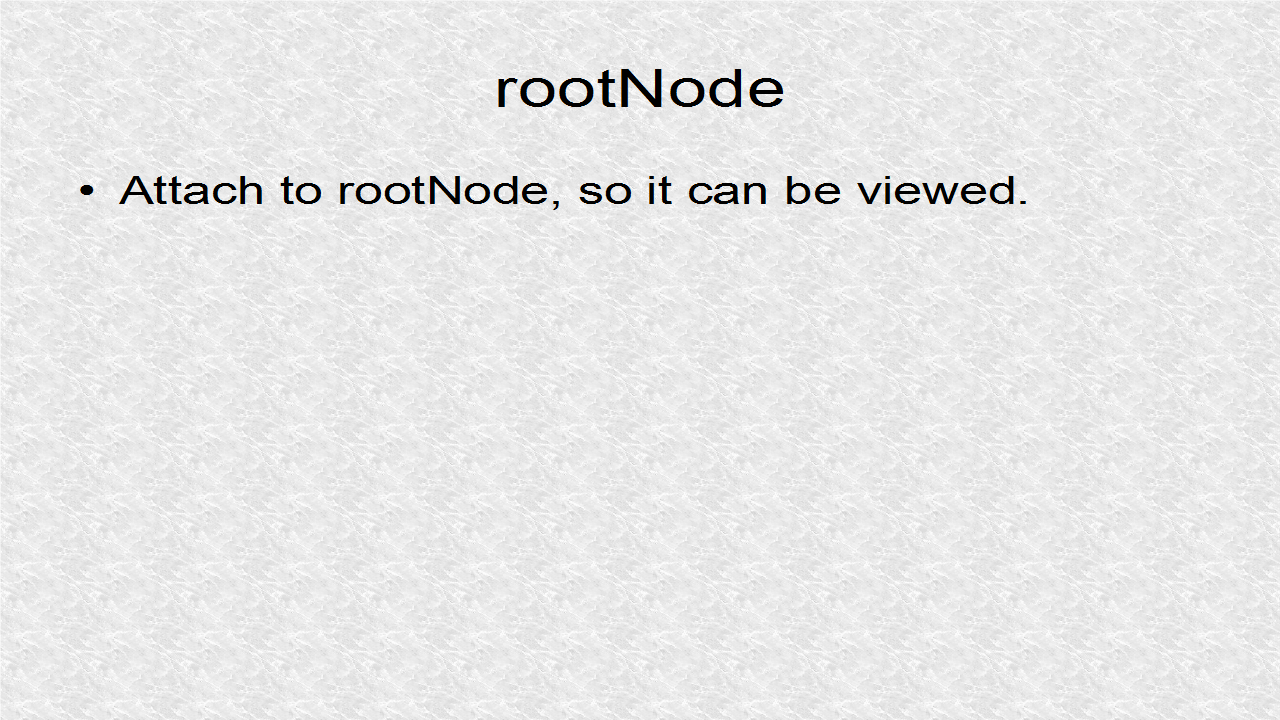
// *** 5. Start (Show it) rootNode.attachChild(ico); // *** 5. End
In the update, we rotate the icosphere in all 3 directions. Since the icosphere is almost spherical, we only note slight changes in the sphere. However, we can easily see the changes in the arrows.
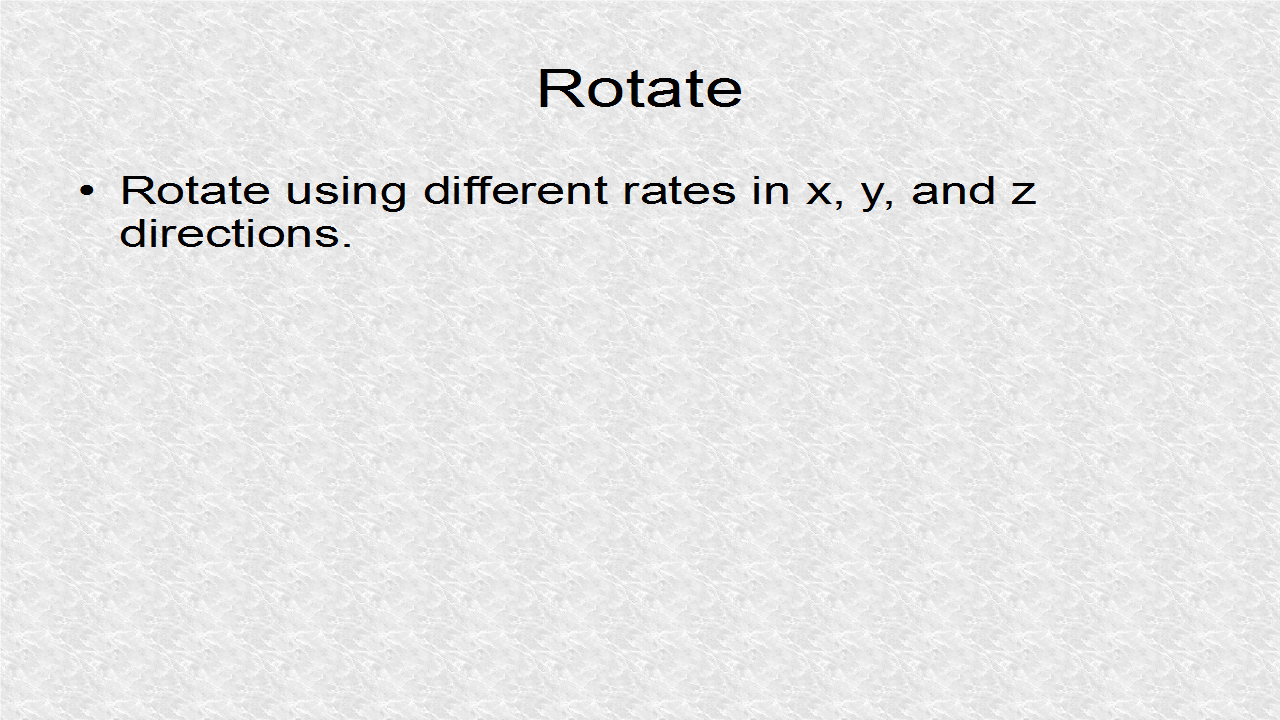
// *** 6. Start (Rotate at different rates in x,y,z) rootNode.rotate(100*FastMath.DEG_TO_RAD*tpf, 200*FastMath.DEG_TO_RAD*tpf, 300*FastMath.DEG_TO_RAD*tpf); // *** 6. End
// JMonkey3.java package mygame; import com.jme3.app.SimpleApplication; import com.jme3.material.Material; import com.jme3.math.ColorRGBA; import com.jme3.math.FastMath; import com.jme3.math.Vector3f; import com.jme3.renderer.RenderManager; import com.jme3.scene.Geometry; import com.jme3.scene.Mesh; import com.jme3.scene.Spatial; import com.jme3.scene.debug.Arrow; public class JMonkey3 extends SimpleApplication { float rot = 0f; public static void main(String[] args) { JMonkey3 app = new JMonkey3(); app.start(); } private Geometry putShape(Mesh shape, ColorRGBA color) { Geometry g = new Geometry("coordinate axis", shape); Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat.getAdditionalRenderState().setWireframe(true); mat.setColor("Color", color); g.setMaterial(mat); rootNode.attachChild(g); return g; } private void attachCoordinateAxes(Vector3f pos){ Arrow arrow = new Arrow(Vector3f.UNIT_X); arrow.setLineWidth(2); putShape(arrow, ColorRGBA.Red).setLocalTranslation(pos); arrow = new Arrow(Vector3f.UNIT_Y); arrow.setLineWidth(2); putShape(arrow, ColorRGBA.Green).setLocalTranslation(pos); arrow = new Arrow(Vector3f.UNIT_Z); arrow.setLineWidth(2); putShape(arrow, ColorRGBA.Blue).setLocalTranslation(pos); } @Override public void simpleInitApp() { // *** 1. Start (Set background color as yellow) viewPort.setBackgroundColor(ColorRGBA.Yellow); // *** 1. End // *** 2. Start (Show coordinates, red x, green y, blue z) attachCoordinateAxes(new Vector3f(0,1,0)); // *** 2. End // *** 3. Start (Load Icoshpere --> ico) Spatial ico = assetManager.loadModel("Models/Icosphere.mesh.xml"); // *** 3. End // *** 4. Start (Set ico to pink color) Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat.setColor("Color", ColorRGBA.Pink); ico.setMaterial(mat); // *** 4. End // *** 5. Start (Show it) rootNode.attachChild(ico); // *** 5. End } @Override public void simpleUpdate(float tpf) { // *** 6. Start (Rotate at different rates in x,y,z) rootNode.rotate(100*FastMath.DEG_TO_RAD*tpf, 200*FastMath.DEG_TO_RAD*tpf, 300*FastMath.DEG_TO_RAD*tpf); // *** 6. End } @Override public void simpleRender(RenderManager rm) { //TODO: add render code } }
Output:
No comments:
Post a Comment