Here 2 random integers are created. We have to use the Random library. To use Random in a scrapbook, we have to right click on the jpage, select Set Imports... and add the java.util package. This is same as writing import java.util.*; in a Java application.
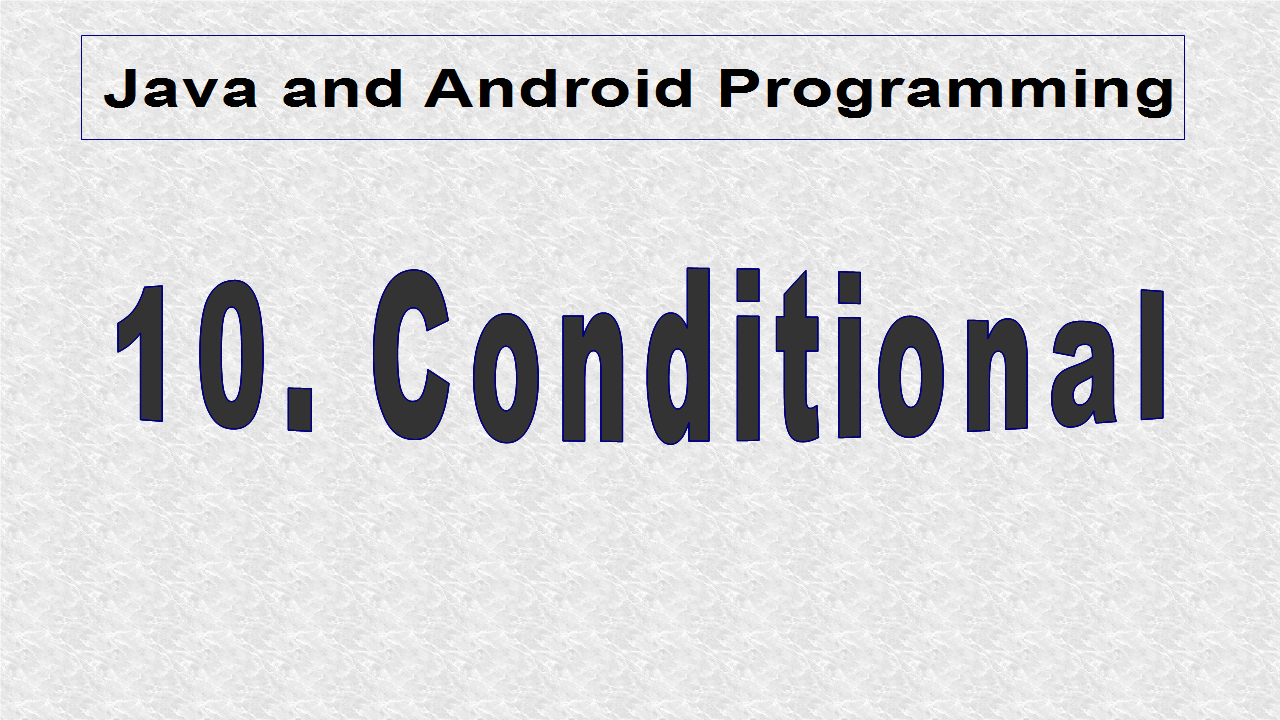
if tests a boolean, and if true, evaluates a block. Since the block in the example is one statement, the brackets are optional. It is a good idea to have them in case in the future you plan to write more statements inside the block.
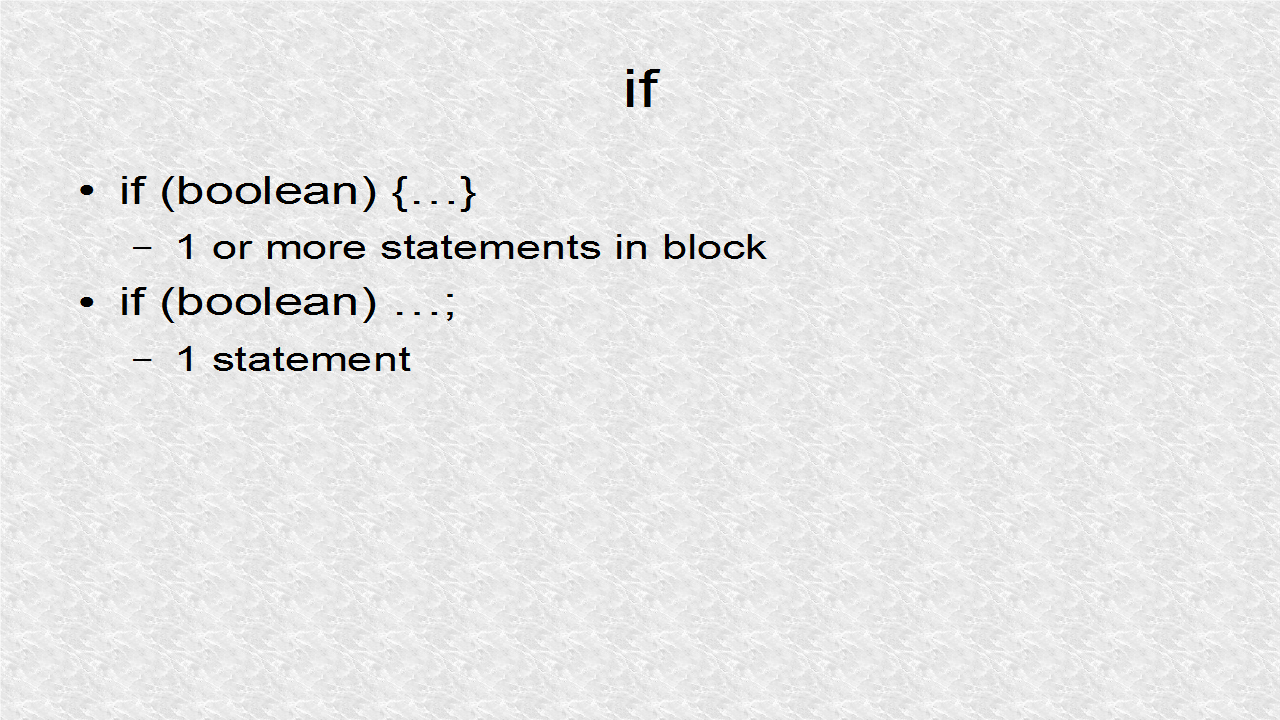
// *** 1. Start (if) Random r = new Random(); int i1 = r.nextInt(5); int i2 = r.nextInt(5); System.out.printf("i1 = %d,\ti2 = %d\n", i1, i2); if (i1<i2) System.out.println("i1 < i2"); if (i1<=i2) System.out.println("i1 <= i2"); if (i1==i2) System.out.println("i1 == i2"); if (i1>i2) System.out.println("i1 > i2"); if (i1>=i2) System.out.println("i1 >= i2"); // *** 1. End
if and if else can be used to test for multiple conditions.
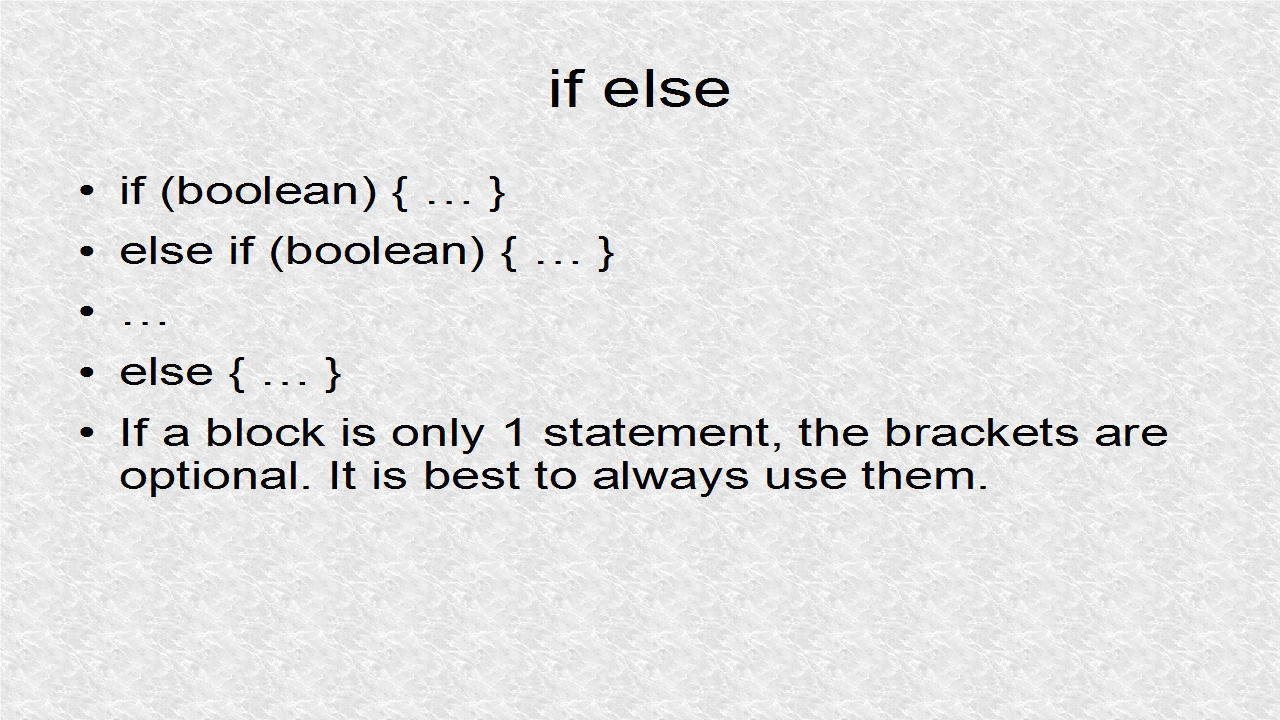
// *** 2. Start (if else) System.out.println("Starting 3..."); if (i1 == 0) System.out.println("i1 == 0"); else if (i1 == 1) System.out.println("i1 == 1"); else if (i1 == 2) System.out.println("i1 == 2"); else if (i1 == 3) System.out.println("i1 == 3"); else if (i1 == 4) System.out.println("i1 == 4"); else if (i1 == 5) System.out.println("i1 == 5"); // *** 2. End
In case you only test one variable for different values, switch often used. The cases have to be literals the variable might be equal to. The variable could be a primitive or a String. We have to use break, so multiple cases are not done.
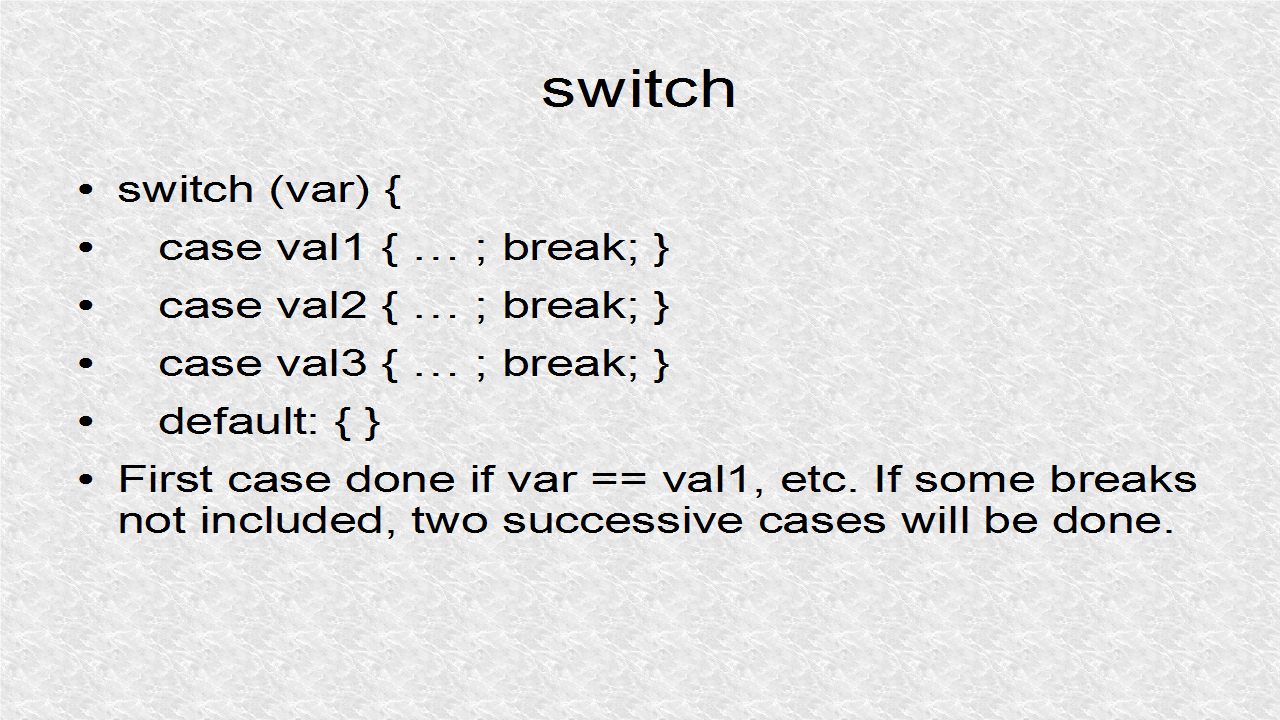
// *** 3. Start (switch) System.out.println("Starting 4..."); switch (i1) { case 0: { System.out.println("i1 == 0"); break; } case 1: { System.out.println("i1 == 1"); break; } case 2: { System.out.println("i1 == 2"); break; } case 3: { System.out.println("i1 == 3"); break; } case 4: { System.out.println("i1 == 4"); break; } default: System.out.println("ERROR"); } // *** 3. End
The ternary operator allows for conditionals to be written in a compact manner. However, if statements can also do the same thing, and might more sense in some cases, for more readable code.
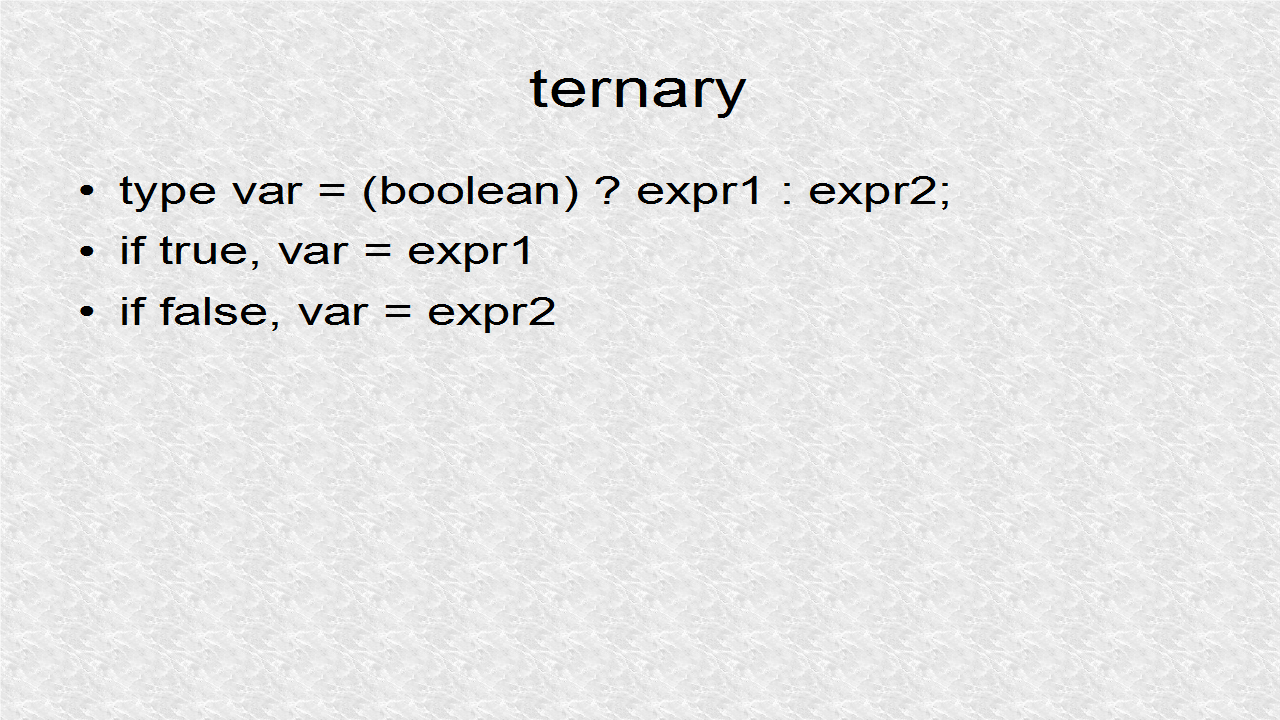
// *** 4. Start (Ternary) System.out.println("Starting 5..."); int i3 = (i2>i1)?i2:i1; String s1 = (i1==i2)?"They are equal":"They are not equal"; System.out.println("i3 = " + i3 + ",\ts1 = " + s1); // *** 4. End
//ex10.jpage // *** 1. Start (if) Random r = new Random(); int i1 = r.nextInt(5); int i2 = r.nextInt(5); System.out.printf("i1 = %d,\ti2 = %d\n", i1, i2); if (i1<i2) System.out.println("i1 < i2"); if (i1<=i2) System.out.println("i1 <= i2"); if (i1==i2) System.out.println("i1 == i2"); if (i1>i2) System.out.println("i1 > i2"); if (i1>=i2) System.out.println("i1 >= i2"); // *** 1. End // *** 2. Start (if else) System.out.println("Starting 3..."); if (i1 == 0) System.out.println("i1 == 0"); else if (i1 == 1) System.out.println("i1 == 1"); else if (i1 == 2) System.out.println("i1 == 2"); else if (i1 == 3) System.out.println("i1 == 3"); else if (i1 == 4) System.out.println("i1 == 4"); else if (i1 == 5) System.out.println("i1 == 5"); // *** 2. End // *** 3. Start (switch) System.out.println("Starting 4..."); switch (i1) { case 0: { System.out.println("i1 == 0"); break; } case 1: { System.out.println("i1 == 1"); break; } case 2: { System.out.println("i1 == 2"); break; } case 3: { System.out.println("i1 == 3"); break; } case 4: { System.out.println("i1 == 4"); break; } default: System.out.println("ERROR"); } // *** 3. End // *** 4. Start (Ternary) System.out.println("Starting 5..."); int i3 = (i2>i1)?i2:i1; String s1 = (i1==i2)?"They are equal":"They are not equal"; System.out.println("i3 = " + i3 + ",\ts1 = " + s1); // *** 4. End
No comments:
Post a Comment