Most real application involve using light to illuminate the mesh.
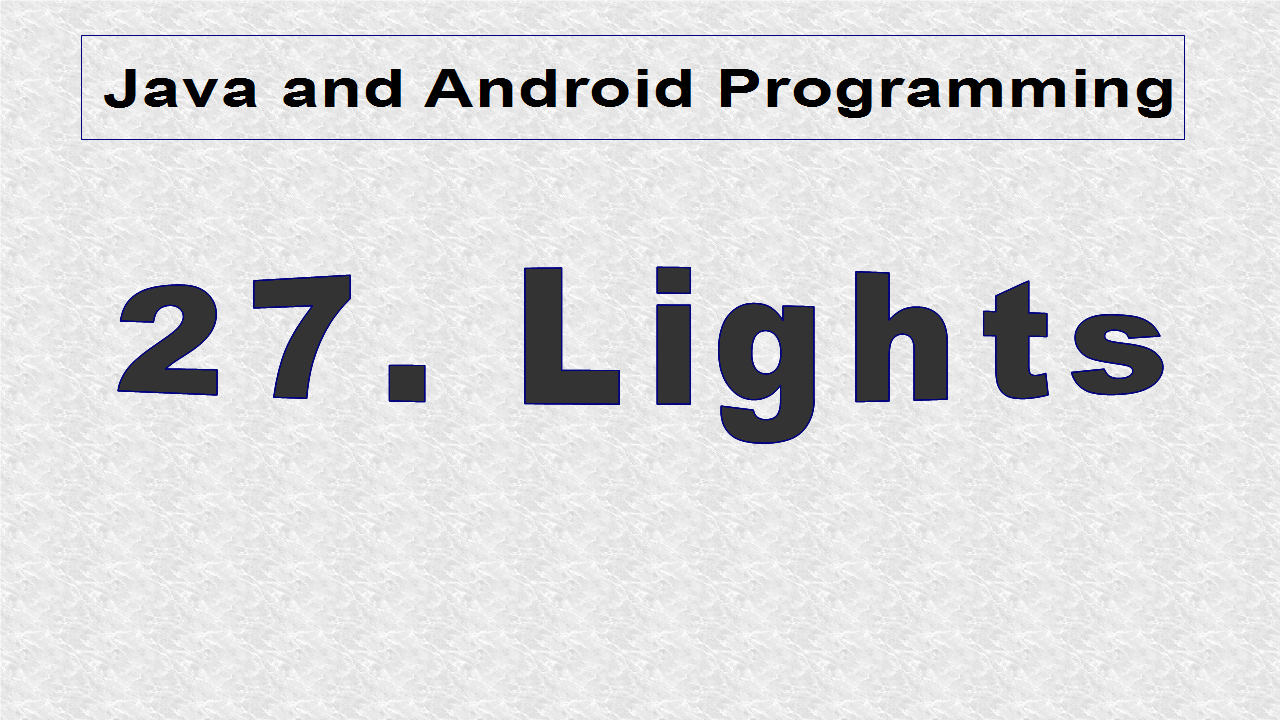
The App Settings are changed as in the previous tutorial.
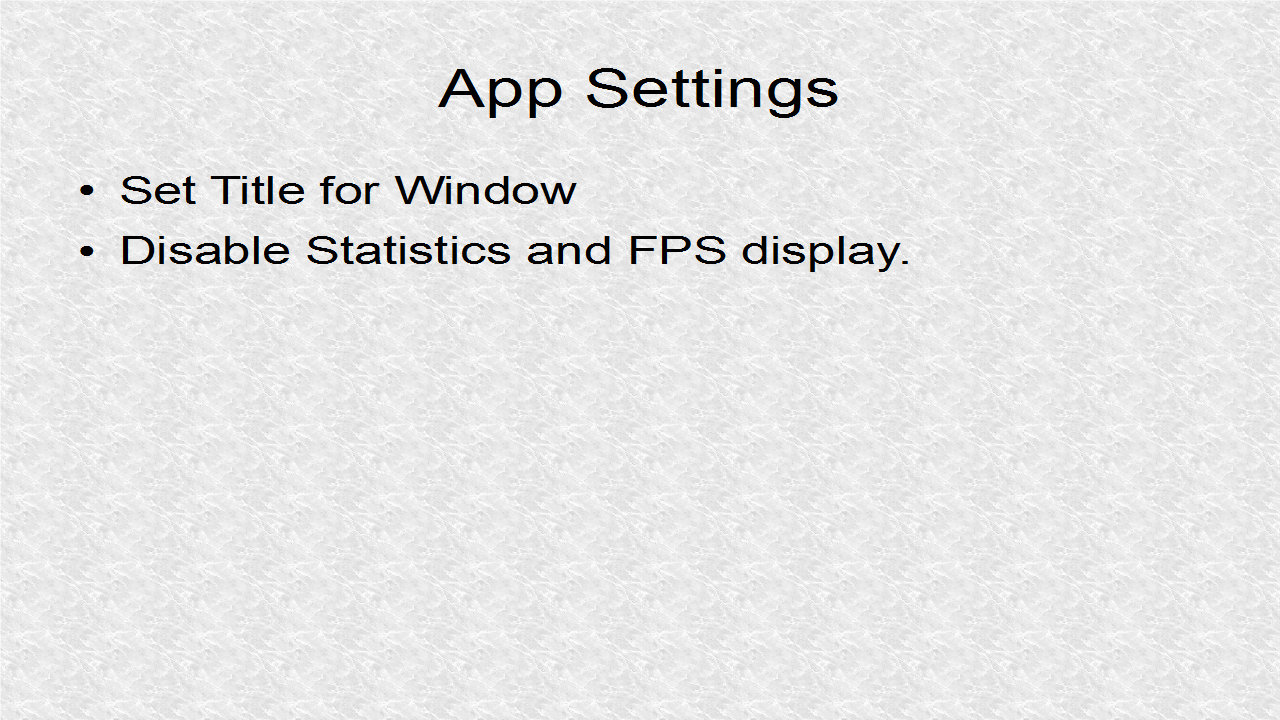
// *** 1. Start (App Settings, App start) AppSettings setting= new AppSettings(true); setting.setTitle("Green Monkey!"); JMonkey27 app = new JMonkey27(); app.setDisplayFps(false); app.setDisplayStatView(false); app.setSettings(setting); app.start(); // *** 1. End
We disable the default camera (flyCam) key mapping. The background is set white. In Blender, delete the default cube, and add a Monkey mesh. Add a material by selecting Material icon and then clicking on New. The Diffuse Color is set to a shade of green. Export the structure using OGRE. Move both mesh and material file to the Models folder in the project. We also have to rename the materials file so it has the same name. Thus, the two files in Models folder are Suzanne.mesh.xml and Suzanne.material.
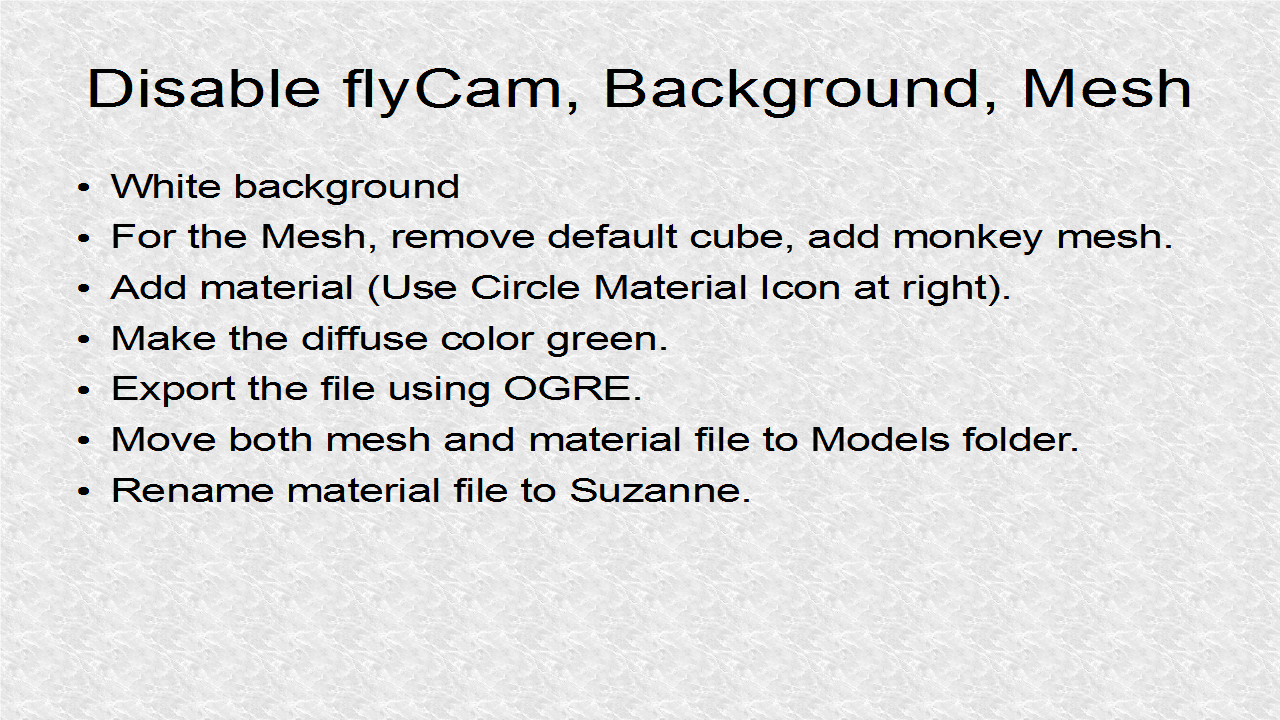
// *** 2. Start (Disable flyCam, White Background, mesh) flyCam.setEnabled(false); viewPort.setBackgroundColor(ColorRGBA.White); geom = assetManager.loadModel("Models/Suzanne.mesh.xml"); // *** 2. End
We can do initial moves and rotates, before adding the mesh to rootNode.
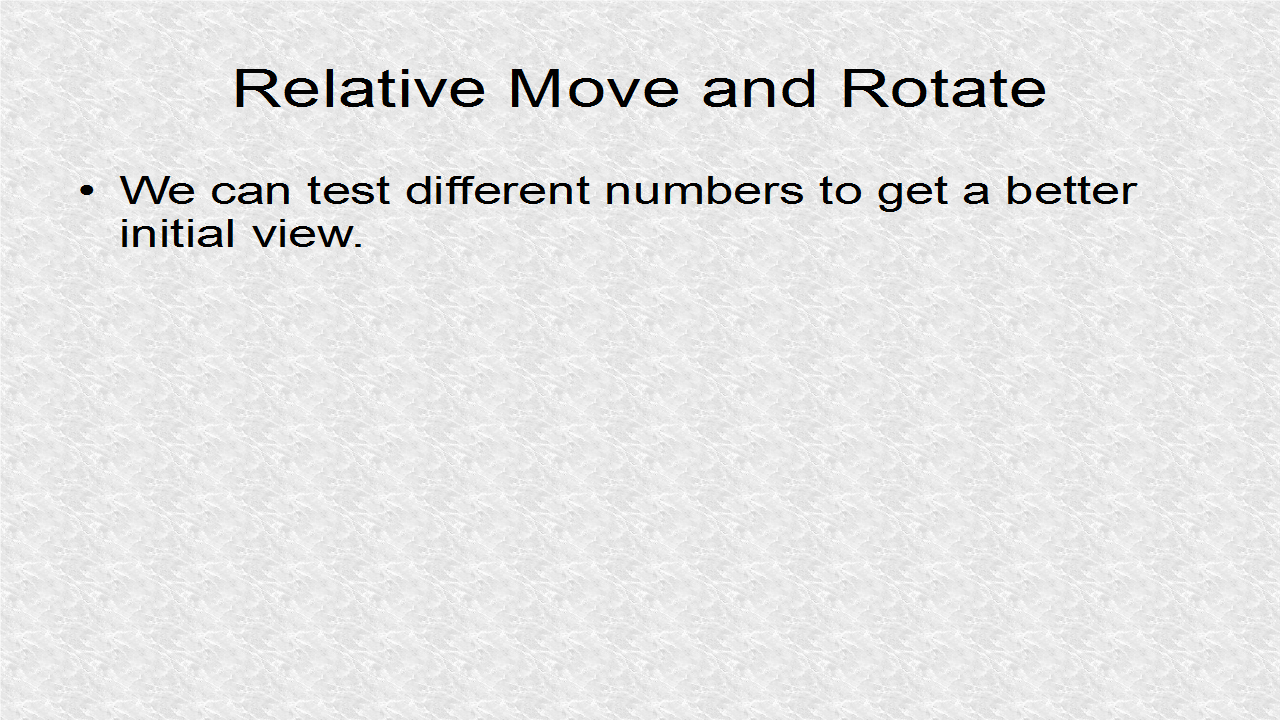
// *** 3. Start (Relative Translate/Rotate) geom.move(2, 1, 0); geom.rotate(0, 2*ANG, 0); rootNode.attachChild(geom); // *** 3. End
A light has to be added to rootNode. The only way to view an object now is if light is reflected by the surface.
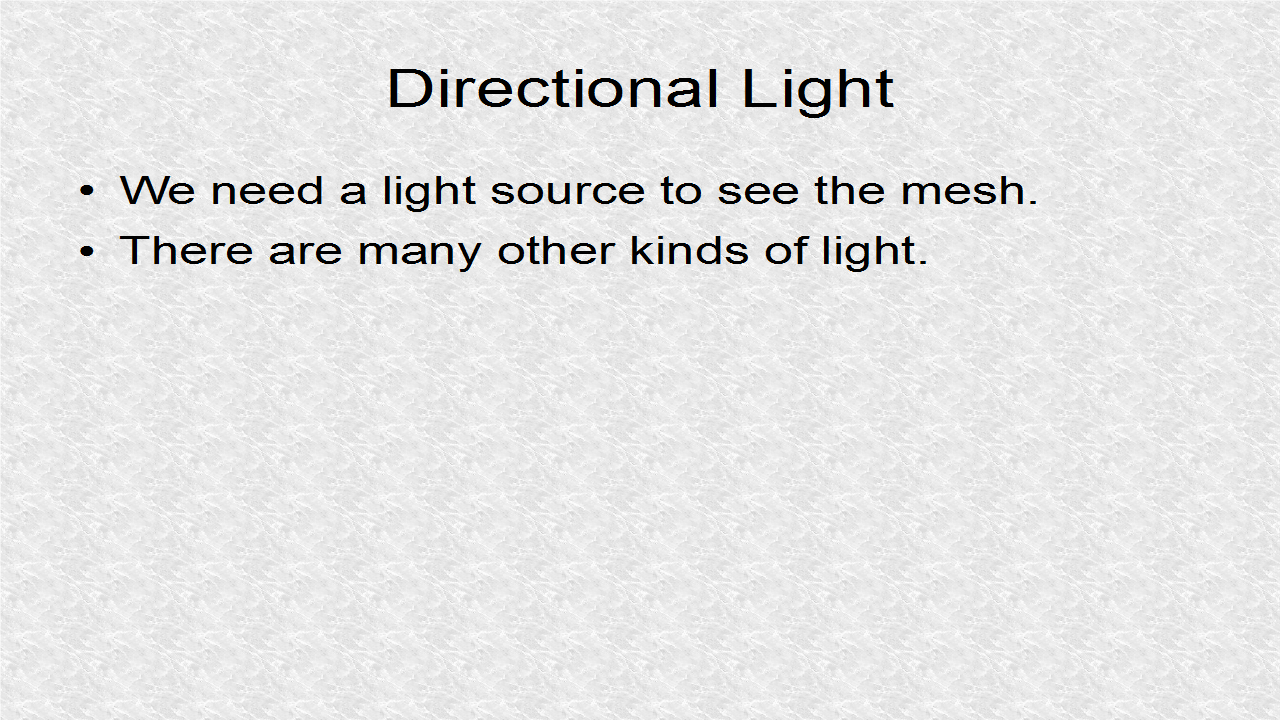
// *** 4. Start (DirectionalLight) DirectionalLight sun = new DirectionalLight(); sun.setDirection(new Vector3f(-0.1f, -0.7f, -1.0f)); rootNode.addLight(sun); // *** 4. End
There are now random rotations in x (1/6 probability), y (2/3 probability) or z (1/6 probability).
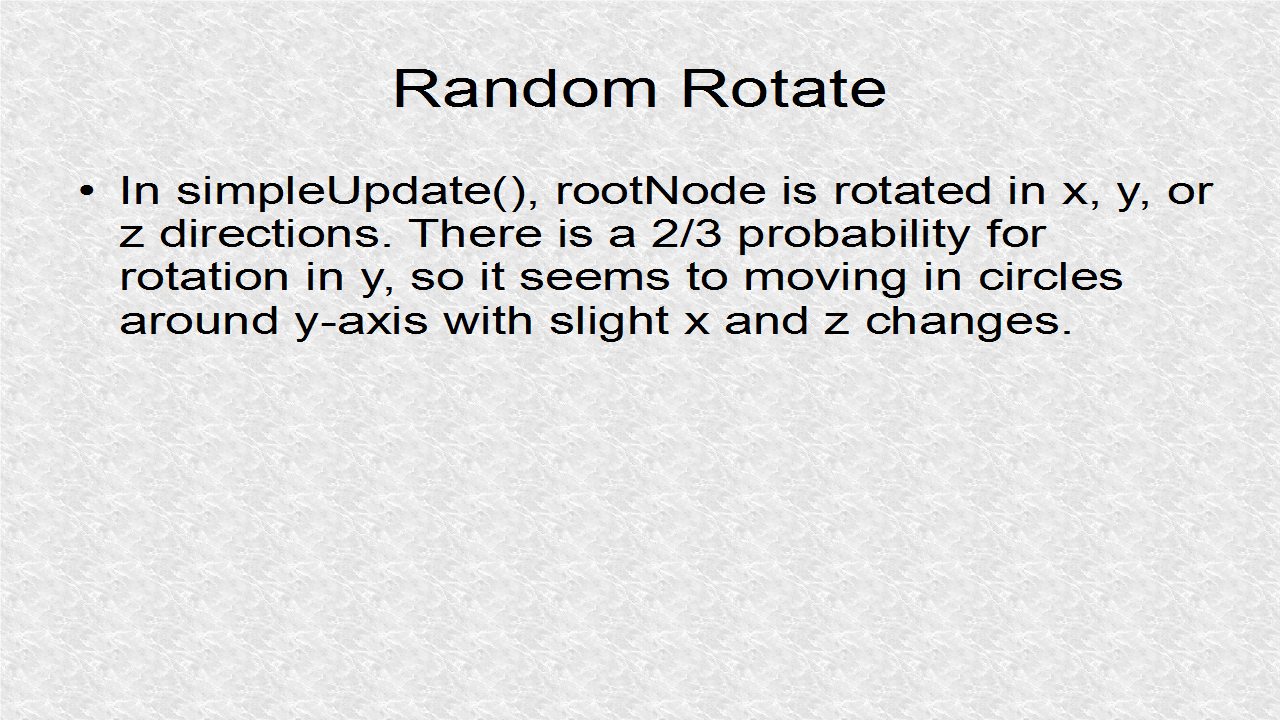
// *** 5. Start (Random Rotate, 2/3 cases for y) switch (FastMath.nextRandomInt(0, 5)) { case 0: rootNode.rotate(ANG*tpf,0,0); break; case 1: case 2: case 3: case 4: rootNode.rotate(0,ANG*tpf,0); break; case 5: rootNode.rotate(0,0,ANG*tpf); break; } // *** 5. End
// JMonkey27.java package mygame; import com.jme3.app.SimpleApplication; import com.jme3.light.DirectionalLight; import com.jme3.material.Material; import com.jme3.math.ColorRGBA; import com.jme3.math.FastMath; import com.jme3.math.Vector3f; import com.jme3.renderer.RenderManager; import com.jme3.scene.Spatial; import com.jme3.system.AppSettings; public class JMonkey27 extends SimpleApplication { Spatial geom; // used by simpleInitApp, simpleUpdate final float ANG = 125*FastMath.DEG_TO_RAD; // constant public static void main(String[] args) { // *** 1. Start (App Settings, App start) AppSettings setting= new AppSettings(true); setting.setTitle("Green Monkey!"); JMonkey27 app = new JMonkey27(); app.setDisplayFps(false); app.setDisplayStatView(false); app.setSettings(setting); app.start(); // *** 1. End } @Override public void simpleInitApp() { // *** 2. Start (Disable flyCam, White Background, mesh) flyCam.setEnabled(false); viewPort.setBackgroundColor(ColorRGBA.White); geom = assetManager.loadModel("Models/Suzanne.mesh.xml"); // *** 2. End // *** 3. Start (Relative Translate/Rotate) geom.move(2, 1, 0); geom.rotate(0, 2*ANG, 0); rootNode.attachChild(geom); // *** 3. End // *** 4. Start (DirectionalLight) DirectionalLight sun = new DirectionalLight(); sun.setDirection(new Vector3f(-0.1f, -0.7f, -1.0f)); rootNode.addLight(sun); // *** 4. End } @Override public void simpleUpdate(float tpf) { // *** 5. Start (Random Rotate, 2/3 cases for y) switch (FastMath.nextRandomInt(0, 5)) { case 0: rootNode.rotate(ANG*tpf,0,0); break; case 1: case 2: case 3: case 4: rootNode.rotate(0,ANG*tpf,0); break; case 5: rootNode.rotate(0,0,ANG*tpf); break; } // *** 5. End } @Override public void simpleRender(RenderManager rm) { //TODO: add render code } }
Output:
No comments:
Post a Comment