The Quad is a 2D Mesh.
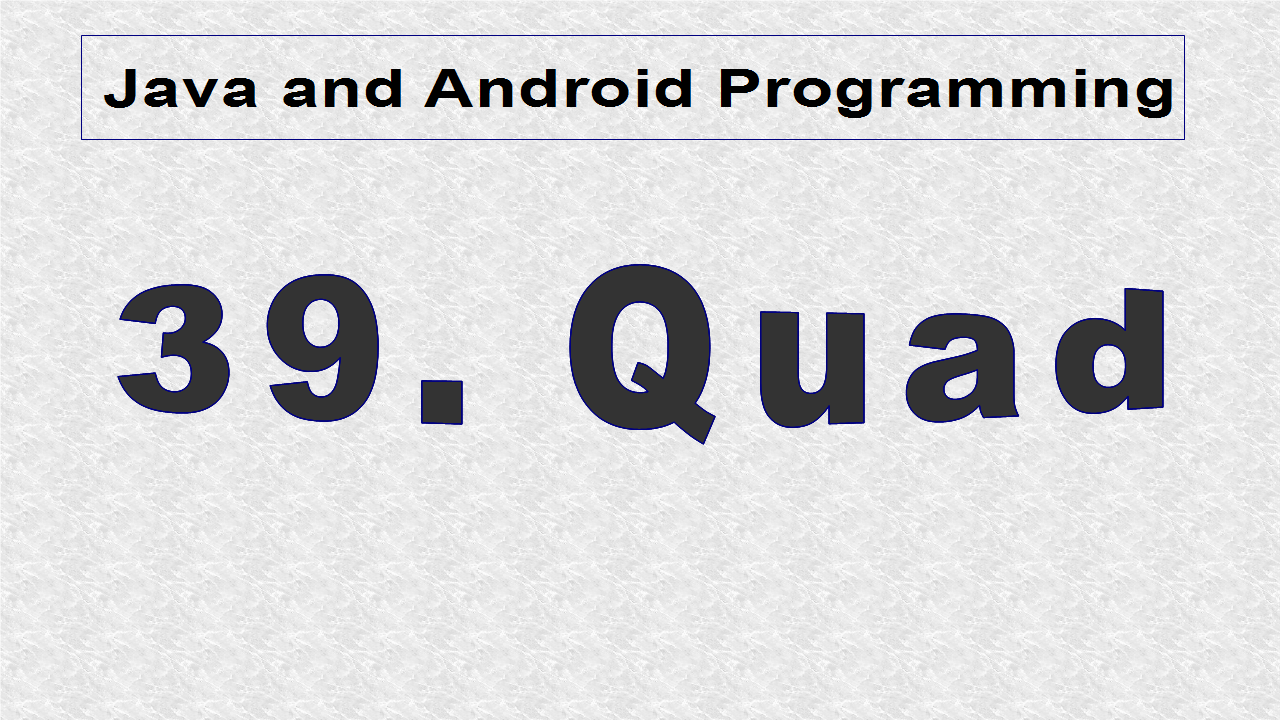
The Mesh and Geometry are created.
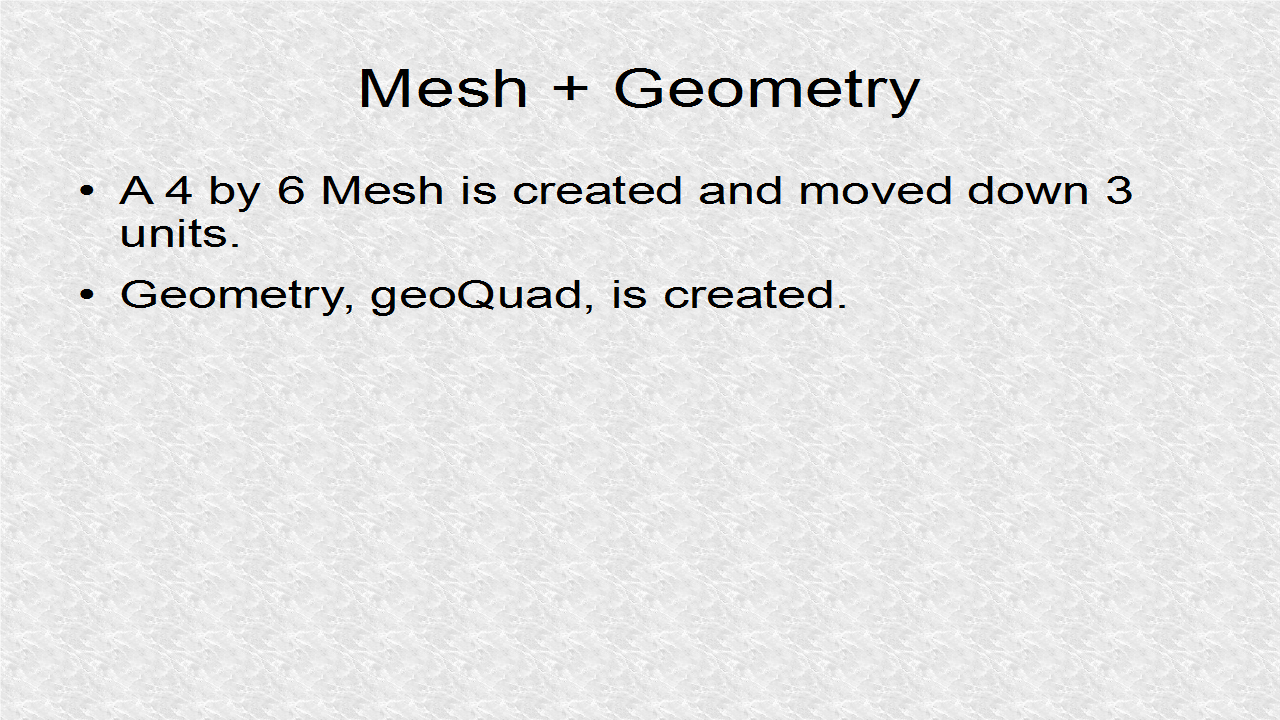
// *** 1. Start (Quad Mesh + Geometry) Quad quad = new Quad(4,6); // 4 by 6 geoQuad = new Geometry("quad", quad); geoQuad.setLocalTranslation(0, -3, 0); // *** 1. End
Some mesh information is printed.
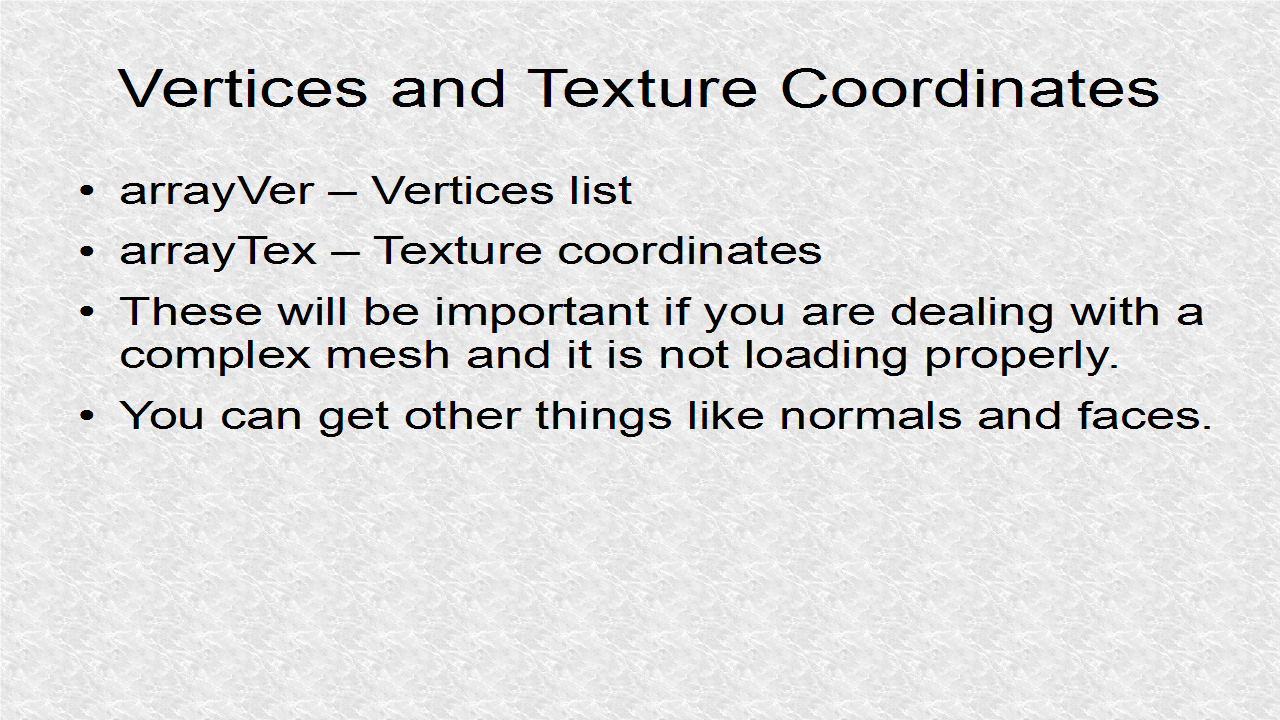
// *** 2. Start (Vertices and Texture coordinates) VertexBuffer position = quad. getBuffer(VertexBuffer.Type.Position); Vector3f[] arrayVer = BufferUtils. getVector3Array((FloatBuffer)position. getData()); VertexBuffer texCoord = quad. getBuffer(VertexBuffer.Type.TexCoord); Vector2f[] arrayTex = BufferUtils. getVector2Array((FloatBuffer)texCoord. getData()); System.out.println("quad has " + arrayVer.length + " vertices. Coordinates for " + " Vertices (x,y,z) and Texture (u,v) are: "); for (int i = 0; i<arrayVer.length; i++) { System.out.printf("%d\t%s\t%s\n",i, arrayVer[i],arrayTex[i]); } // *** 2. End
The material shows the back shadow and we also use the transparency to blend with the background color.
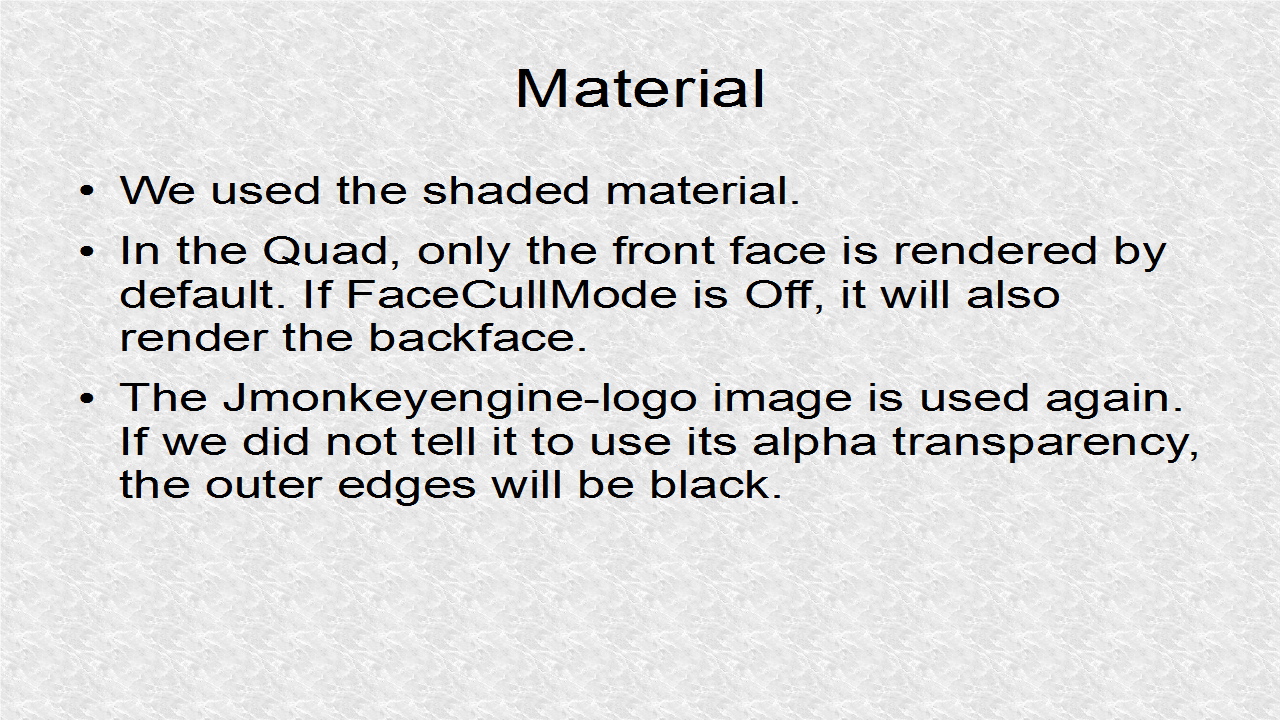
// *** 3. Start (Material) Material mat = new Material(assetManager, "Common/MatDefs/Light/Lighting.j3md"); mat.getAdditionalRenderState(). setFaceCullMode(FaceCullMode.Off); Texture quadTex = assetManager.loadTexture( "Interface/monkey/Jmonkeyengine-logo.png"); mat.setTexture("DiffuseMap", quadTex); mat.getAdditionalRenderState(). setBlendMode(BlendMode.Alpha); geoQuad.setMaterial(mat); // *** 3. End
// JMonkey39.java package mygame; import com.jme3.app.SimpleApplication; import com.jme3.light.DirectionalLight; import com.jme3.light.SpotLight; import com.jme3.material.Material; import com.jme3.material.RenderState.BlendMode; import com.jme3.material.RenderState.FaceCullMode; import com.jme3.math.ColorRGBA; import com.jme3.math.FastMath; import com.jme3.math.Vector2f; import com.jme3.math.Vector3f; import com.jme3.renderer.RenderManager; import com.jme3.scene.Geometry; import com.jme3.scene.VertexBuffer; import com.jme3.scene.shape.Quad; import com.jme3.system.AppSettings; import com.jme3.texture.Texture; import com.jme3.util.BufferUtils; import java.nio.FloatBuffer; public class JMonkey39 extends SimpleApplication { public static void main(String[] args) { AppSettings settings = new AppSettings(true); settings.setFrameRate(60); settings.setTitle("Monkey on a Quad !"); JMonkey39 app = new JMonkey39(); app.setSettings(settings); app.start(); } private Geometry geoQuad; @Override public void simpleInitApp() { viewPort.setBackgroundColor( new ColorRGBA(1f,.9f,.8f,1)); setDisplayFps(false); setDisplayStatView(false); flyCam.setEnabled(false); // *** 1. Start (Quad Mesh + Geometry) Quad quad = new Quad(4,6); // 4 by 6 geoQuad = new Geometry("quad", quad); geoQuad.setLocalTranslation(0, -3, 0); // *** 1. End // *** 2. Start (Vertices and Texture coordinates) VertexBuffer position = quad. getBuffer(VertexBuffer.Type.Position); Vector3f[] arrayVer = BufferUtils. getVector3Array((FloatBuffer)position. getData()); VertexBuffer texCoord = quad. getBuffer(VertexBuffer.Type.TexCoord); Vector2f[] arrayTex = BufferUtils. getVector2Array((FloatBuffer)texCoord. getData()); System.out.println("quad has " + arrayVer.length + " vertices. Coordinates for " + " Vertices (x,y,z) and Texture (u,v) are: "); for (int i = 0; i<arrayVer.length; i++) { System.out.printf("%d\t%s\t%s\n",i, arrayVer[i],arrayTex[i]); } // *** 2. End // *** 3. Start (Material) Material mat = new Material(assetManager, "Common/MatDefs/Light/Lighting.j3md"); mat.getAdditionalRenderState(). setFaceCullMode(FaceCullMode.Off); Texture quadTex = assetManager.loadTexture( "Interface/monkey/Jmonkeyengine-logo.png"); mat.setTexture("DiffuseMap", quadTex); mat.getAdditionalRenderState(). setBlendMode(BlendMode.Alpha); geoQuad.setMaterial(mat); // *** 3. End /** A white, directional light source */ DirectionalLight sun = new DirectionalLight(); sun.setDirection((new Vector3f(-0.5f, -0.5f, -0.5f)).normalizeLocal()); sun.setColor(ColorRGBA.White); rootNode.addLight(sun); /** A cone-shaped spotlight with location, direction, range */ SpotLight spot = new SpotLight(); spot.setSpotRange(100); spot.setSpotOuterAngle(20 * FastMath.DEG_TO_RAD); spot.setSpotInnerAngle(15 * FastMath.DEG_TO_RAD); spot.setDirection(cam.getDirection()); spot.setPosition(cam.getLocation()); rootNode.addLight(spot); rootNode.attachChild(geoQuad); } @Override public void simpleUpdate(float tpf) { geoQuad.rotate(0,FastMath.HALF_PI*tpf,0); } @Override public void simpleRender(RenderManager rm) { } }
Output:
No comments:
Post a Comment