Line is one of the built-in mesh shapes.
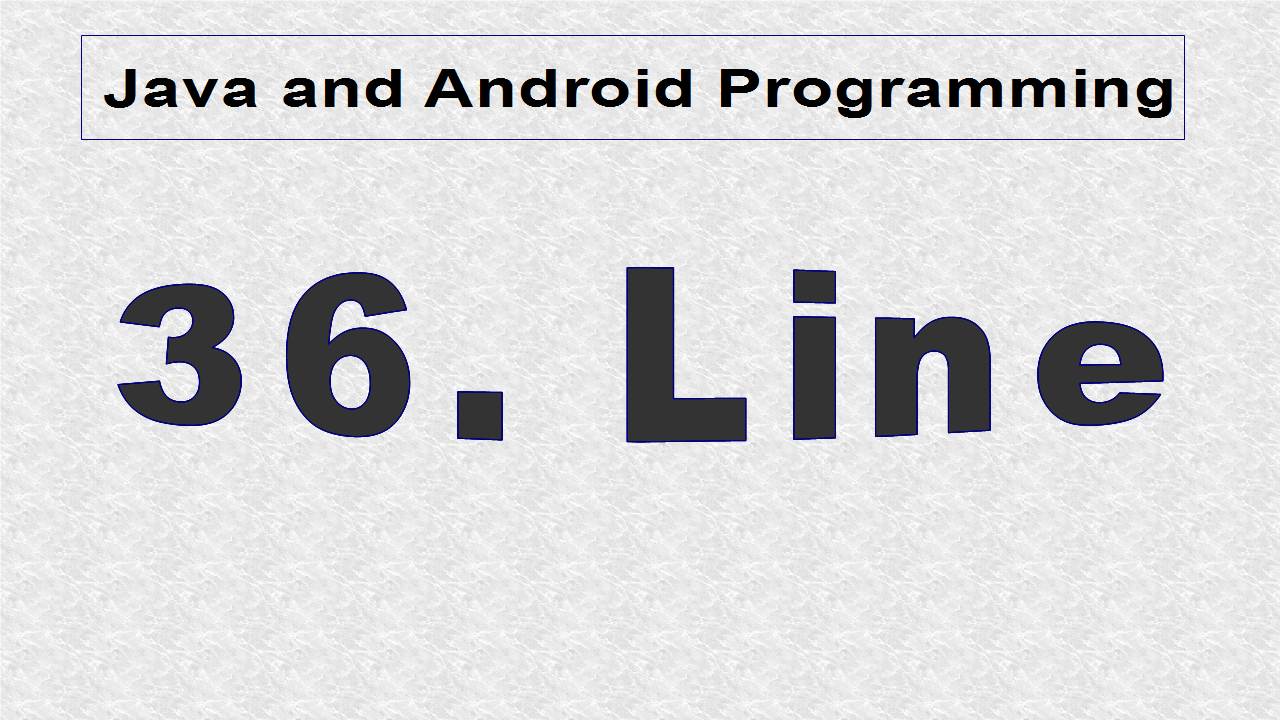
Line requires the starting point and ending point, both being Vector3f objects. We can also set Line properties such as the line width.
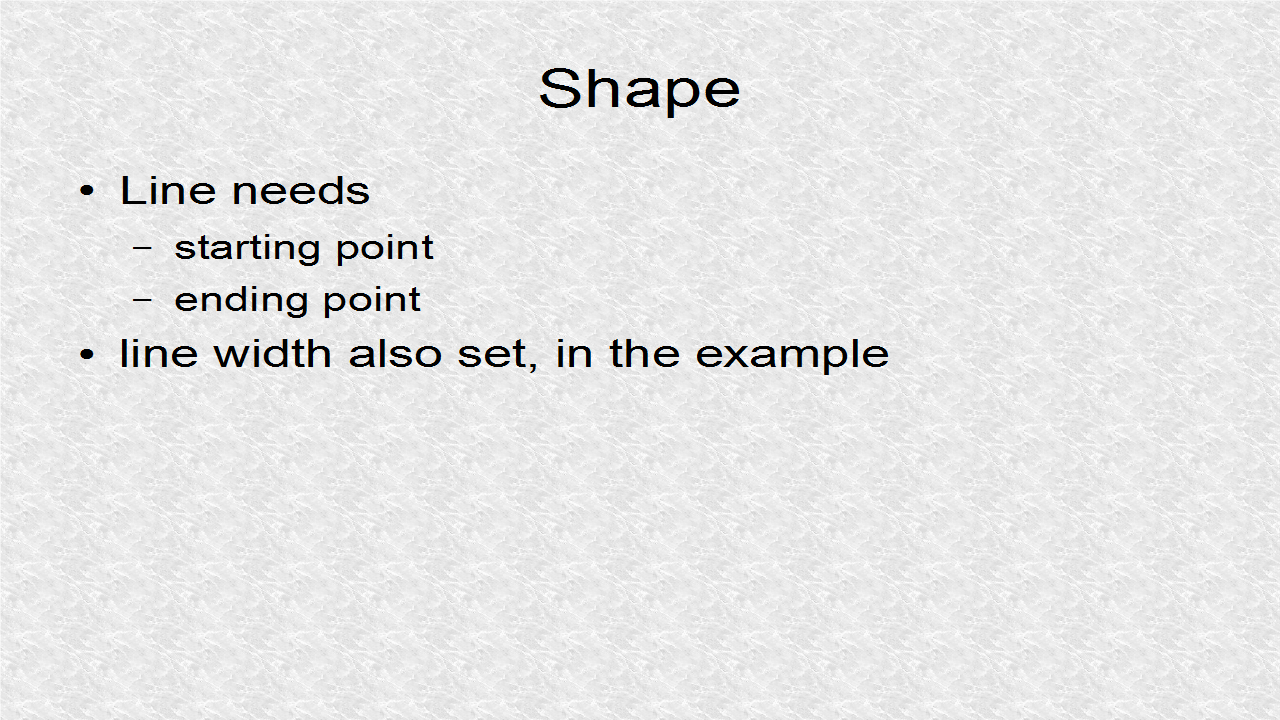
// *** 1. Start (Line objects) Line line = new Line(Vector3f.ZERO,new Vector3f(1,1,0)); line.setLineWidth(5); Line line1 = new Line(Vector3f.ZERO,new Vector3f(1,-1f,0)); line1.setLineWidth(5); Line line2 = new Line(Vector3f.ZERO,new Vector3f(1,0,3)); line2.setLineWidth(3); // *** 1. End
Three Geometries are created from the line shapes. We have to use a String identifier and mesh object.
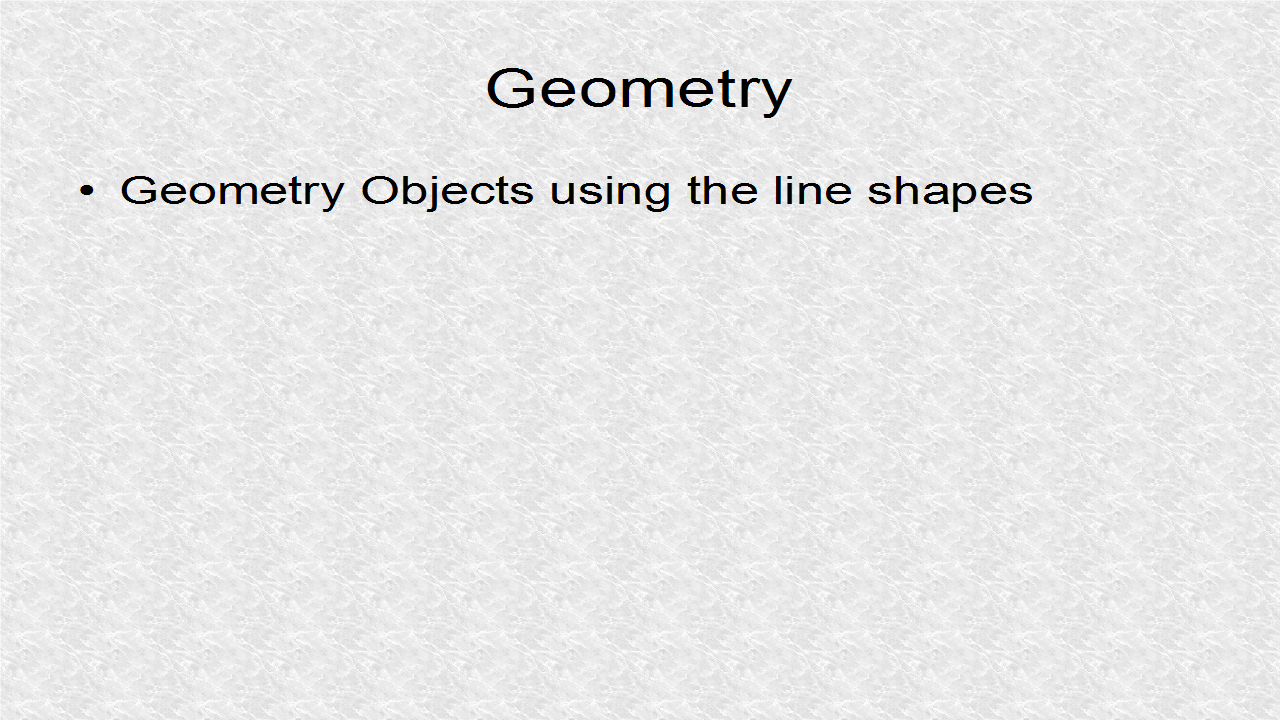
// *** 2. Start (Geometry objects) Geometry gline = new Geometry("upLine",line); Geometry gline1 = new Geometry("downLine",line1); Geometry gline2 = new Geometry("horizLine",line2); // *** 2. End
The three lines are set to different colors.
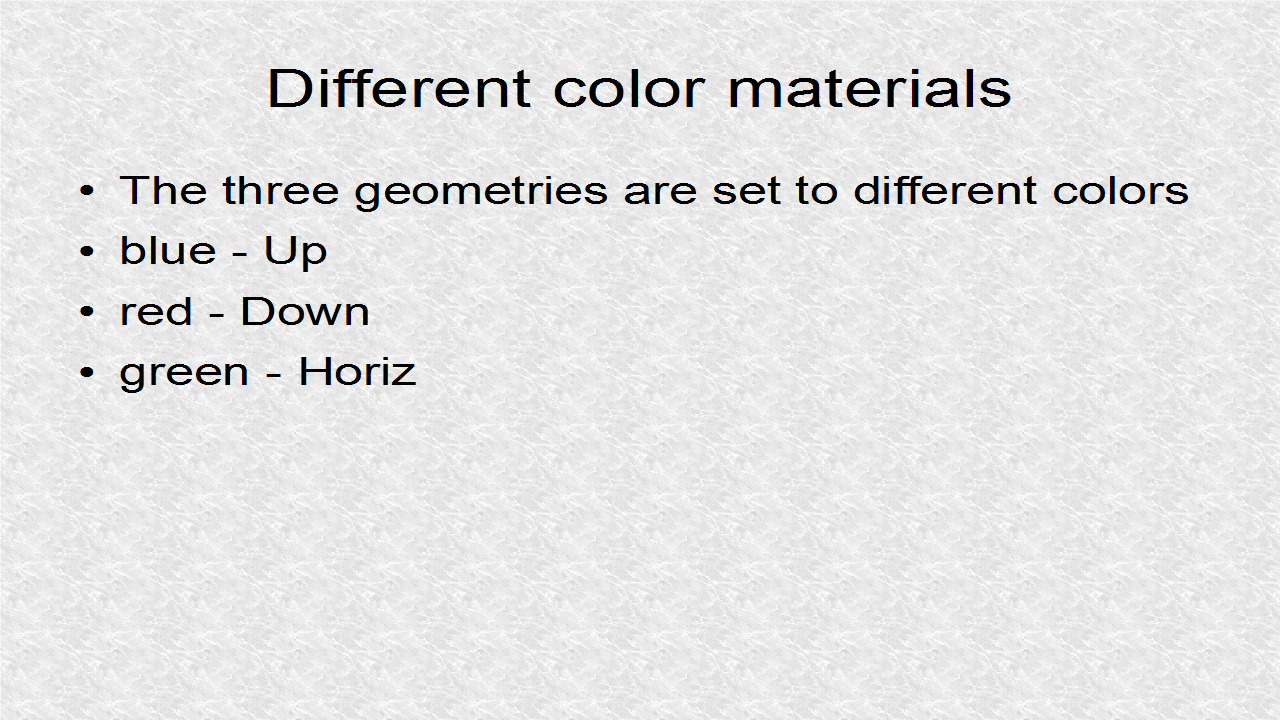
// *** 3. Start (3 Materials, Different Colors) Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat.setColor("Color", ColorRGBA.Blue); Material mat1 = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat1.setColor("Color", ColorRGBA.Red); Material mat2 = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat2.setColor("Color", ColorRGBA.Green); gline.setMaterial(mat); gline1.setMaterial(mat1); gline2.setMaterial(mat2); // *** 3. End
We clone the last 3 Geometries, and Scale and Translate them. If you did not scale them, it would be hard to see them.
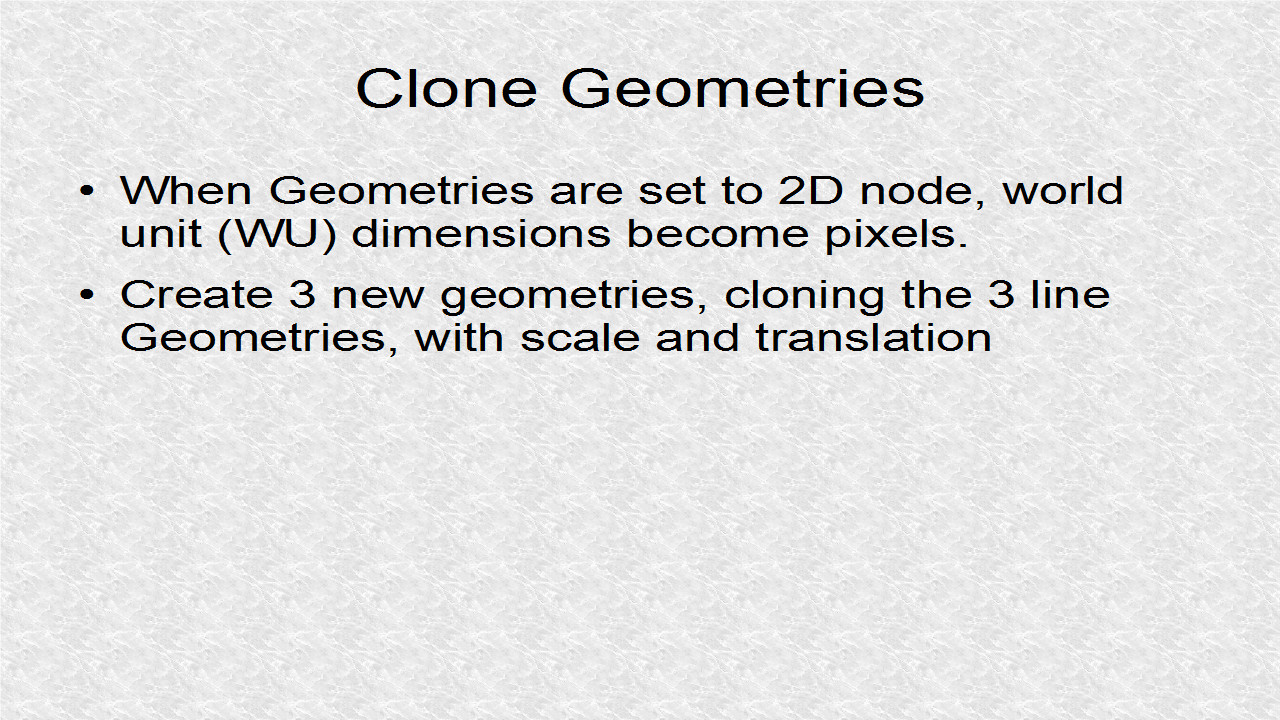
// *** 4. Start (clones with scale and translations) Geometry gline3 = new Geometry("line3"); Geometry gline4 = new Geometry("line4"); Geometry gline5 = new Geometry("line5"); gline3 = gline.clone(); gline3.setLocalScale(20); gline3.setLocalTranslation(x, y, 0); gline4 = gline1.clone(); gline4.setLocalScale(20); gline4.setLocalTranslation(x, y, 0); gline5 = gline2.clone(); gline5.setLocalScale(20); gline5.setLocalTranslation(x, y, 0); // *** 4. End
package mygame; import com.jme3.app.SimpleApplication; import com.jme3.material.Material; import com.jme3.math.ColorRGBA; import com.jme3.math.Vector3f; import com.jme3.renderer.RenderManager; import com.jme3.scene.Geometry; import com.jme3.scene.shape.Line; public class JMonkey36 extends SimpleApplication { public static void main(String[] args) { JMonkey36 app = new JMonkey36(); app.start(); } @Override public void simpleInitApp() { setDisplayFps(false); setDisplayStatView(false); viewPort.setBackgroundColor(ColorRGBA.White); // *** 1. Start (Line objects) Line line = new Line(Vector3f.ZERO,new Vector3f(1,1,0)); line.setLineWidth(5); Line line1 = new Line(Vector3f.ZERO,new Vector3f(1,-1f,0)); line1.setLineWidth(5); Line line2 = new Line(Vector3f.ZERO,new Vector3f(1,0,3)); line2.setLineWidth(3); // *** 1. End // *** 2. Start (Geometry objects) Geometry gline = new Geometry("upLine",line); Geometry gline1 = new Geometry("downLine",line1); Geometry gline2 = new Geometry("horizLine",line2); // *** 2. End // *** 3. Start (3 Materials, Different Colors) Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat.setColor("Color", ColorRGBA.Blue); Material mat1 = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat1.setColor("Color", ColorRGBA.Red); Material mat2 = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat2.setColor("Color", ColorRGBA.Green); gline.setMaterial(mat); gline1.setMaterial(mat1); gline2.setMaterial(mat2); // *** 3. End int x = settings.getWidth()/4; // 2dgui traslated int y = settings.getHeight()/4; // to (x,y) // *** 4. Start (clones with scale and translations) Geometry gline3 = new Geometry("line3"); Geometry gline4 = new Geometry("line4"); Geometry gline5 = new Geometry("line5"); gline3 = gline.clone(); gline3.setLocalScale(20); gline3.setLocalTranslation(x, y, 0); gline4 = gline1.clone(); gline4.setLocalScale(20); gline4.setLocalTranslation(x, y, 0); gline5 = gline2.clone(); gline5.setLocalScale(20); gline5.setLocalTranslation(x, y, 0); // *** 4. End rootNode.attachChild(gline); rootNode.attachChild(gline1); rootNode.attachChild(gline2); guiNode.attachChild(gline3); guiNode.attachChild(gline4); guiNode.attachChild(gline5); } @Override public void simpleUpdate(float tpf) { } @Override public void simpleRender(RenderManager rm) { } }
No comments:
Post a Comment