Sphere and Dome are two built-in mesh shapes.
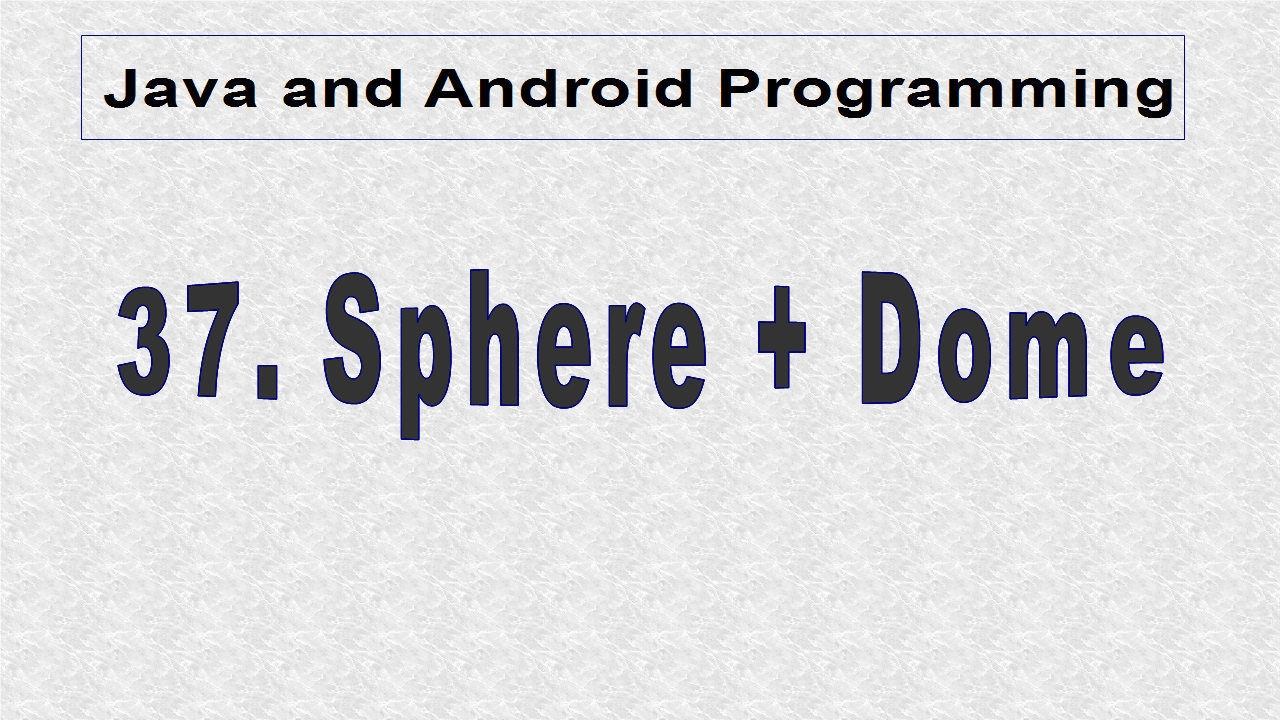
One Sphere and two Dome objects are created with constructors setting number of mesh divisions and radius. The Geometry has to be created.
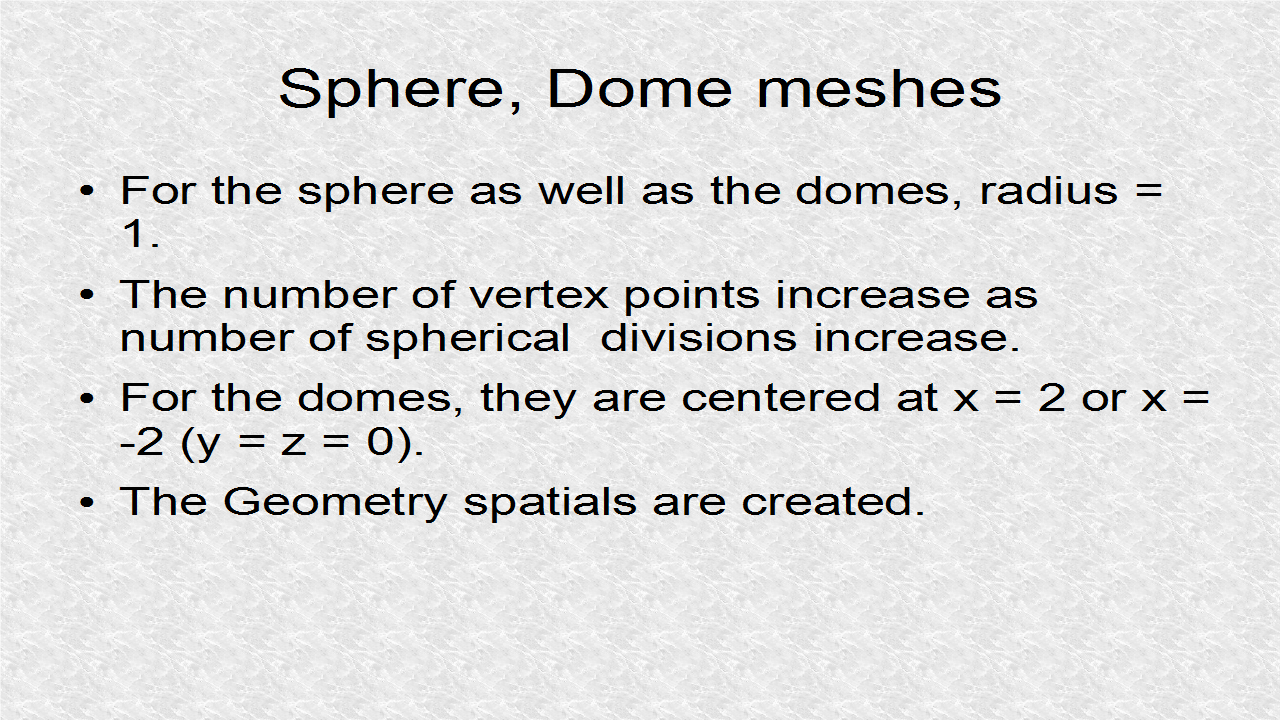
// *** 1. Start (Sphere, Dome1, Dome2) Sphere sphere = new Sphere(16,16,1); Geometry geoSphere = new Geometry("sphere", sphere); Dome dome1 = new Dome(new Vector3f(2,0,0),16,16,1); Geometry geoDome1 = new Geometry("dome1", dome1); Dome dome2 = new Dome(new Vector3f(-2,0,0),16,16,1); Geometry geoDome2 = new Geometry("dome2", dome2); geoDome2.rotate(FastMath.PI,0,0); // *** 1. End
We print the number of vertex points in each structure.
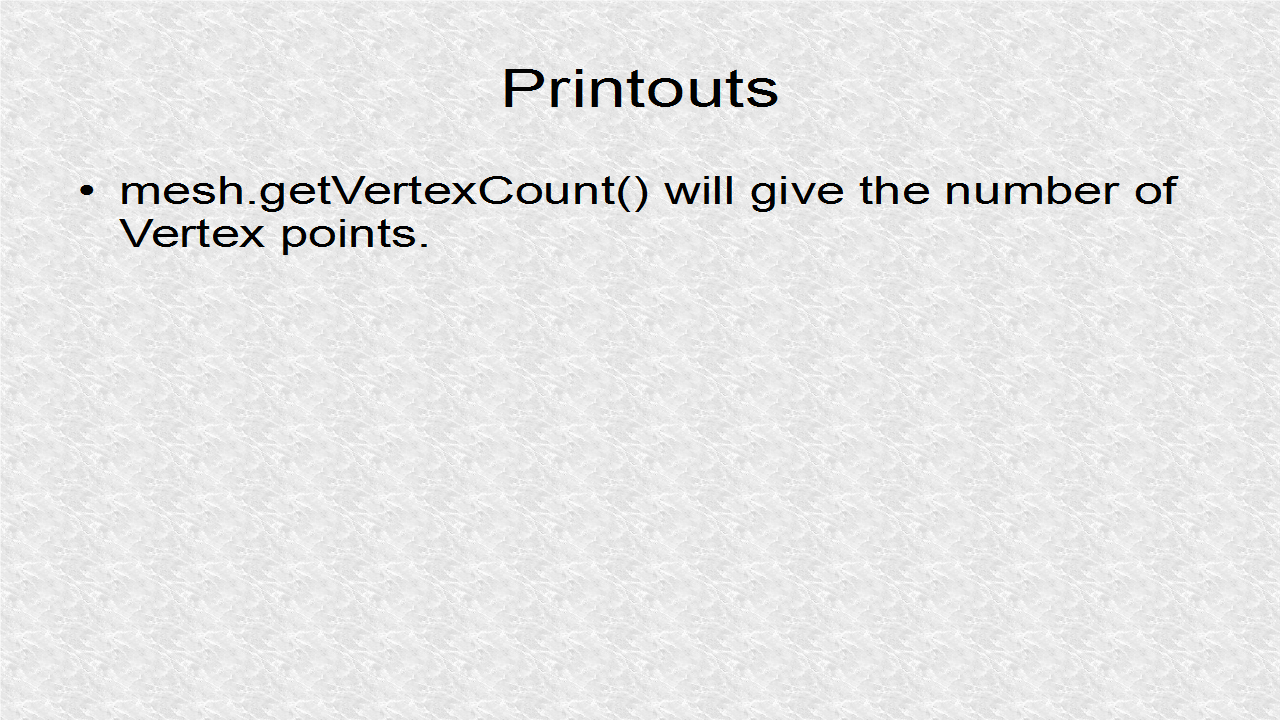
// *** 2. Start (Number of Vertex Points) System.out.println("The sphere has " + sphere.getVertexCount() + " vertices."); System.out.println("The dome1 has " + dome1.getVertexCount() + " vertices."); System.out.println("The dome2 has " + dome2.getVertexCount() + " vertices."); // *** 2. End
The three Geometries are set as blue, red or green.
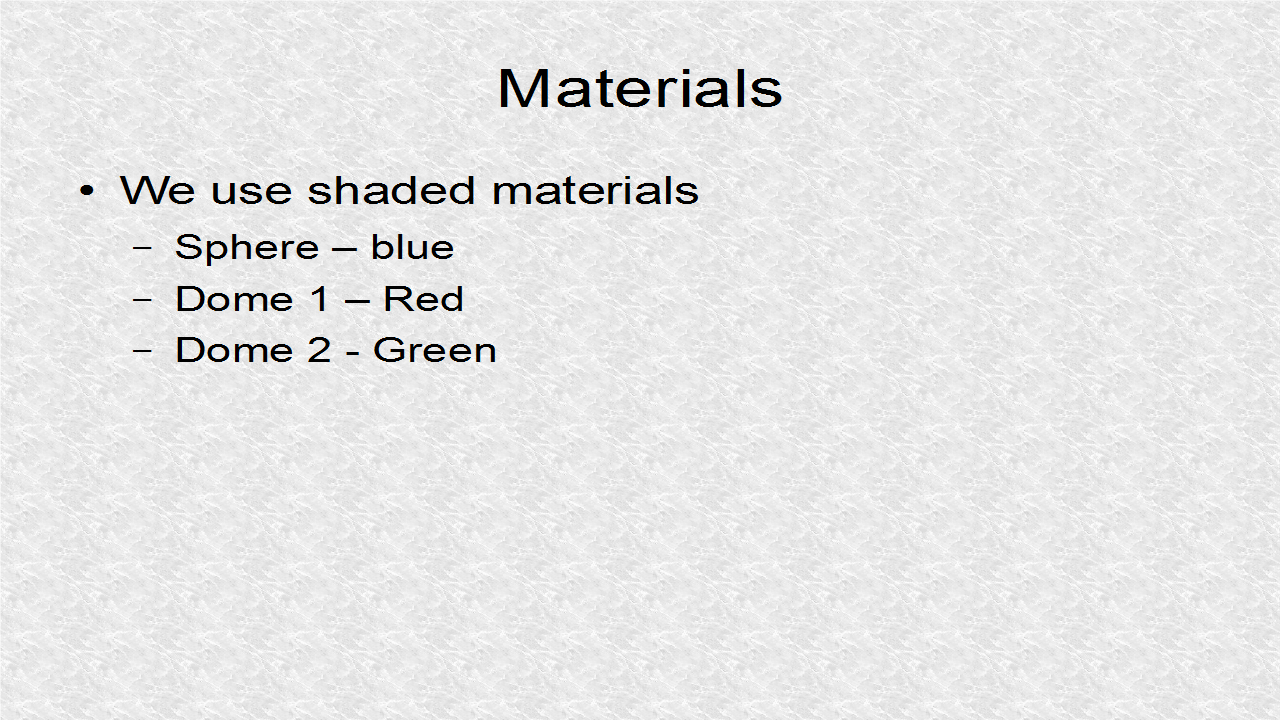
// *** 3. Start (Materials - Blue, Red, Green) Material mat = new Material(assetManager, "Common/MatDefs/Light/Lighting.j3md"); mat.setBoolean("UseMaterialColors",true); mat.setColor("Diffuse",ColorRGBA.Blue); mat.setColor("Specular",ColorRGBA.White); geoSphere.setMaterial(mat); Material mat1 = new Material(assetManager, "Common/MatDefs/Light/Lighting.j3md"); mat1.setBoolean("UseMaterialColors",true); mat1.setColor("Diffuse",ColorRGBA.Red); mat1.setColor("Specular",ColorRGBA.White); geoDome1.setMaterial(mat1); Material mat2 = new Material(assetManager, "Common/MatDefs/Light/Lighting.j3md"); mat2.setBoolean("UseMaterialColors",true); mat2.setColor("Diffuse",ColorRGBA.Green); mat2.setColor("Specular",ColorRGBA.White); geoDome2.setMaterial(mat2); // *** 3. End
// JMonkey37.java package mygame; import com.jme3.app.SimpleApplication; import com.jme3.light.SpotLight; import com.jme3.material.Material; import com.jme3.math.ColorRGBA; import com.jme3.math.FastMath; import com.jme3.math.Vector3f; import com.jme3.renderer.RenderManager; import com.jme3.scene.Geometry; import com.jme3.scene.shape.Dome; import com.jme3.scene.shape.Sphere; public class JMonkey37 extends SimpleApplication { public static void main(String[] args) { JMonkey37 app = new JMonkey37(); app.start(); } @Override public void simpleInitApp() { flyCam.setEnabled(false); viewPort.setBackgroundColor(ColorRGBA.White); setDisplayFps(false); setDisplayStatView(false); // *** 1. Start (Sphere, Dome1, Dome2) Sphere sphere = new Sphere(16,16,1); Geometry geoSphere = new Geometry("sphere", sphere); Dome dome1 = new Dome(new Vector3f(2,0,0),16,16,1); Geometry geoDome1 = new Geometry("dome1", dome1); Dome dome2 = new Dome(new Vector3f(-2,0,0),16,16,1); Geometry geoDome2 = new Geometry("dome2", dome2); geoDome2.rotate(FastMath.PI,0,0); // *** 1. End // *** 2. Start (Number of Vertex Points) System.out.println("The sphere has " + sphere.getVertexCount() + " vertices."); System.out.println("The dome1 has " + dome1.getVertexCount() + " vertices."); System.out.println("The dome2 has " + dome2.getVertexCount() + " vertices."); // *** 2. End // *** 3. Start (Materials - Blue, Red, Green) Material mat = new Material(assetManager, "Common/MatDefs/Light/Lighting.j3md"); mat.setBoolean("UseMaterialColors",true); mat.setColor("Diffuse",ColorRGBA.Blue); mat.setColor("Specular",ColorRGBA.White); geoSphere.setMaterial(mat); Material mat1 = new Material(assetManager, "Common/MatDefs/Light/Lighting.j3md"); mat1.setBoolean("UseMaterialColors",true); mat1.setColor("Diffuse",ColorRGBA.Red); mat1.setColor("Specular",ColorRGBA.White); geoDome1.setMaterial(mat1); Material mat2 = new Material(assetManager, "Common/MatDefs/Light/Lighting.j3md"); mat2.setBoolean("UseMaterialColors",true); mat2.setColor("Diffuse",ColorRGBA.Green); mat2.setColor("Specular",ColorRGBA.White); geoDome2.setMaterial(mat2); // *** 3. End /** A cone-shaped spotlight with location, direction, range */ SpotLight spot = new SpotLight(); spot = new SpotLight(); spot.setSpotRange(100); spot.setSpotOuterAngle(20 * FastMath.DEG_TO_RAD); spot.setSpotInnerAngle(15 * FastMath.DEG_TO_RAD); spot.setDirection(cam.getDirection()); spot.setPosition(cam.getLocation()); rootNode.addLight(spot); rootNode.attachChild(geoSphere); rootNode.attachChild(geoDome1); rootNode.attachChild(geoDome2); } @Override public void simpleUpdate(float tpf) { rootNode.rotate(2*tpf,tpf,2*tpf); } @Override public void simpleRender(RenderManager rm) { } }
Output:
No comments:
Post a Comment