RigidBodyControl can either be static or dynamic, depending on mass.
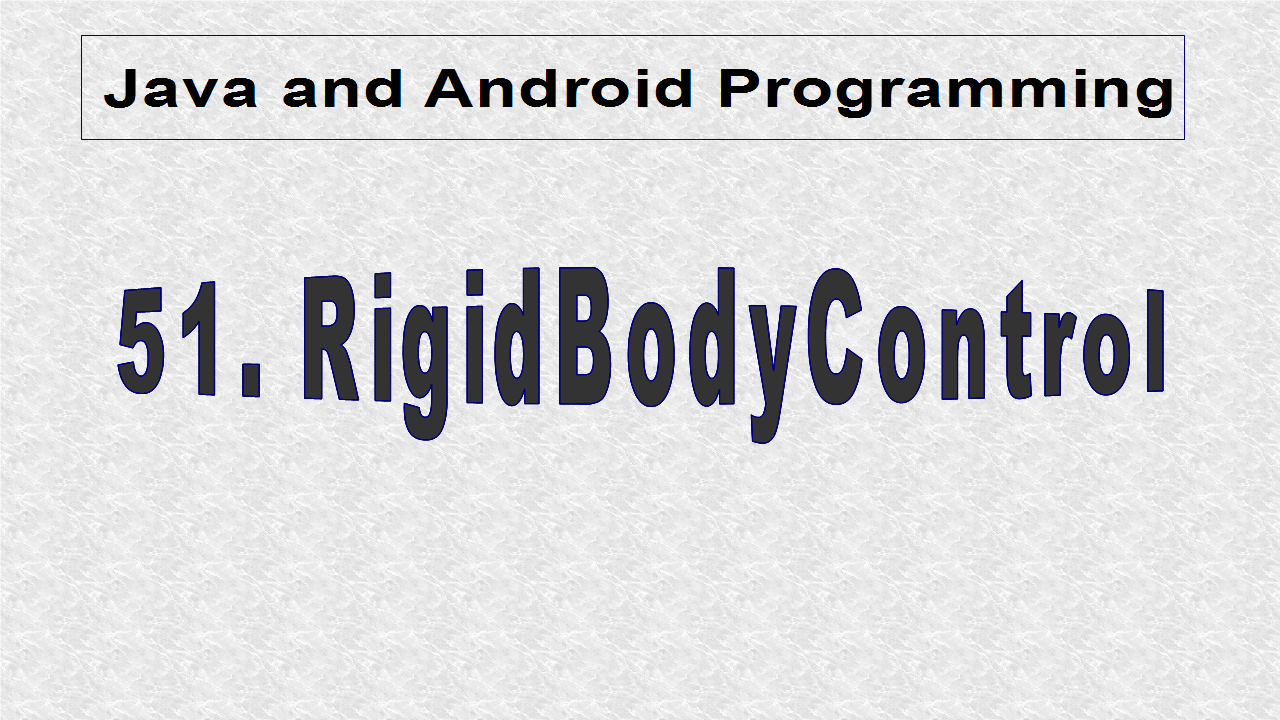
The camera is moved and rotated, so it as y = 20, pointing to origin.
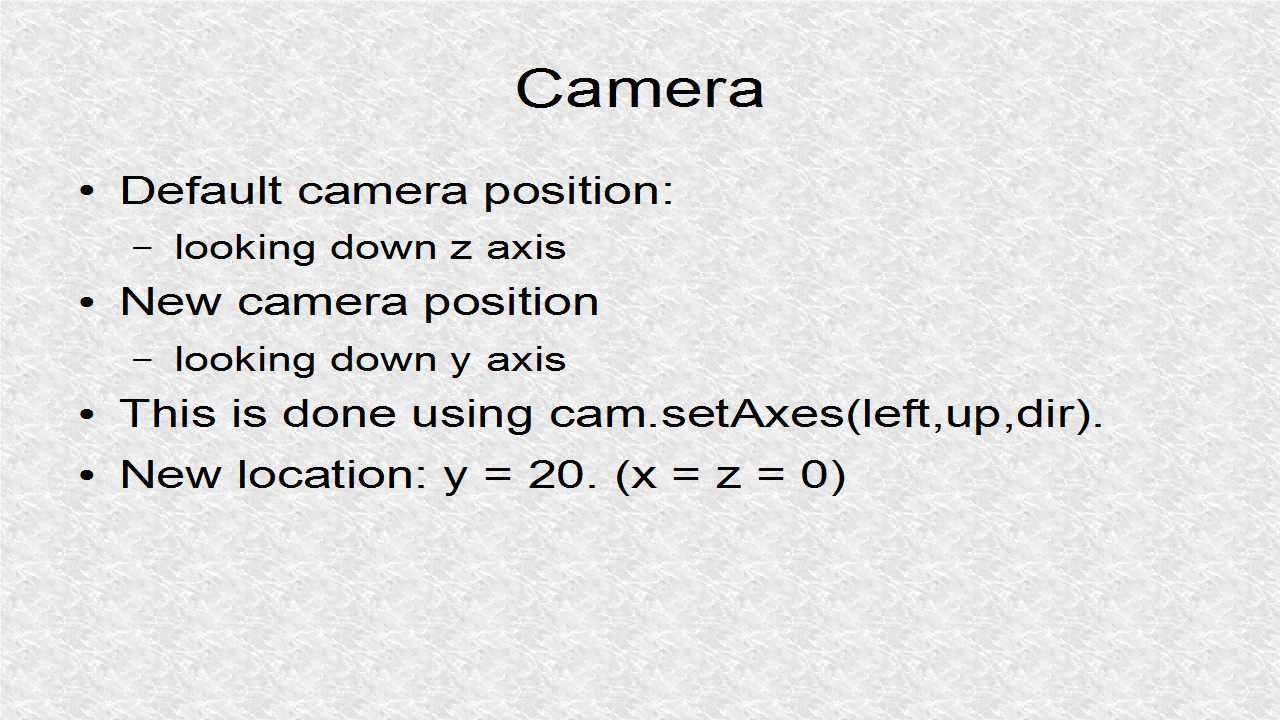
// *** 1. Start (Camera +y looking down y) cam.setAxes(new Vector3f(-1,0,0), // left new Vector3f(0,0,-1), // up new Vector3f(0,-1,0)); // dir cam.setLocation(new Vector3f(0,20,0)); // above ground cam.update(); // *** 1. End
The Application has to use BulletAppState object to enable physics.
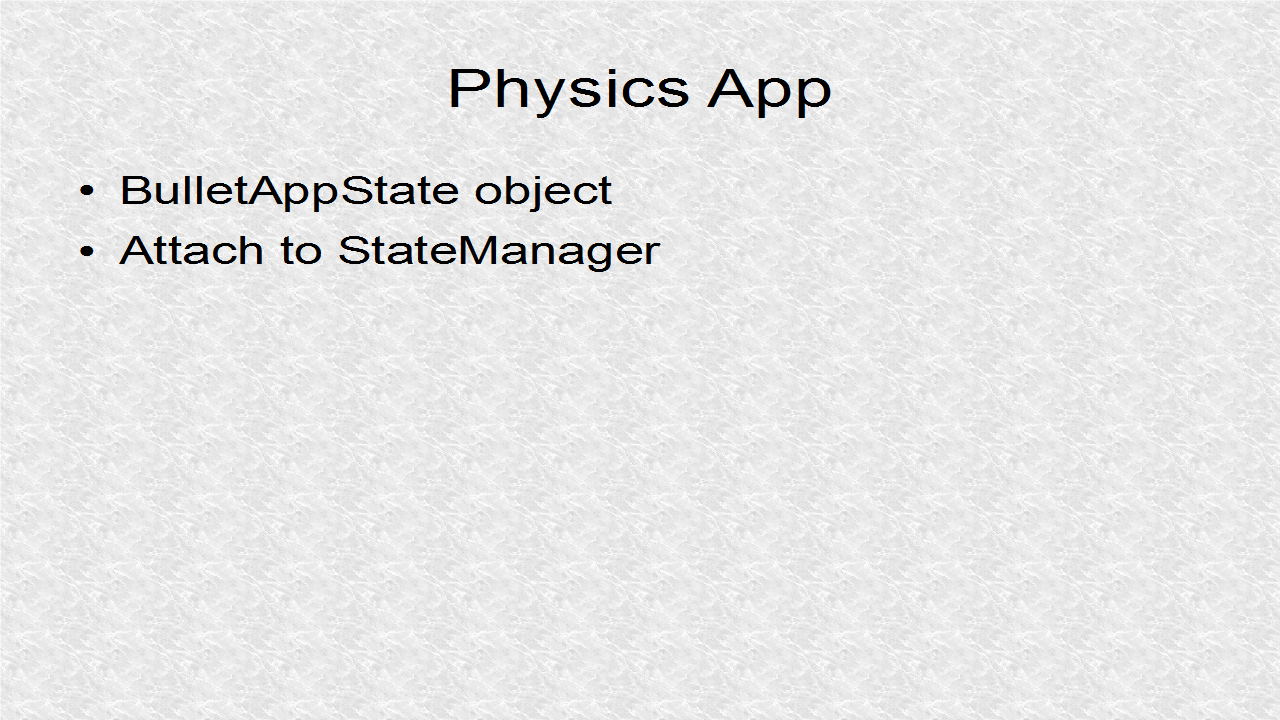
// *** 2. Start (Set Physics) bulletAppState = new BulletAppState(); stateManager.attach(bulletAppState); // *** 2. End
The floor is a static Box mesh object.
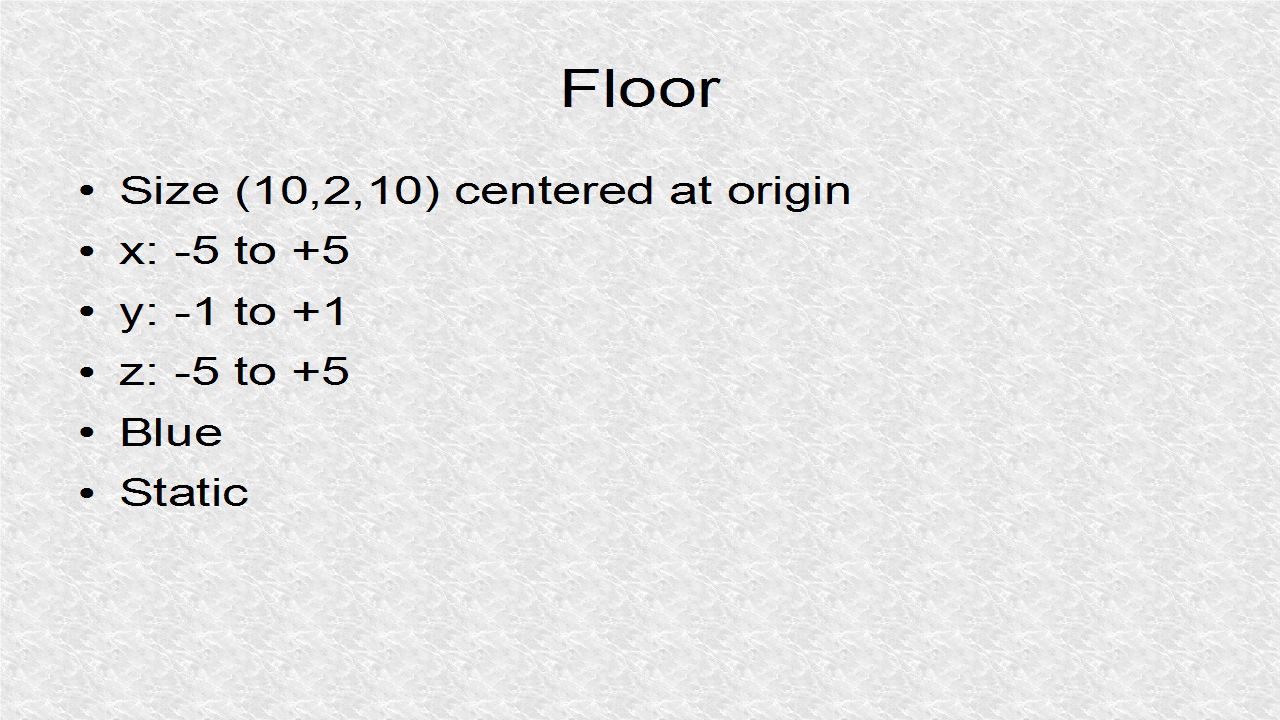
// *** 3. Start (Floor) Box bFloor = new Box(5, 1, 5); Geometry geomFloor = new Geometry("Floor", bFloor); Material matFloor = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matFloor.setColor("Color", ColorRGBA.Blue); geomFloor.setMaterial(matFloor); rootNode.attachChild(geomFloor); RigidBodyControl floorPhy = new RigidBodyControl(0.0f); geomFloor.addControl(floorPhy); bulletAppState.getPhysicsSpace().add(floorPhy); // *** 3. End
The North Wall is also a static Box mesh object.
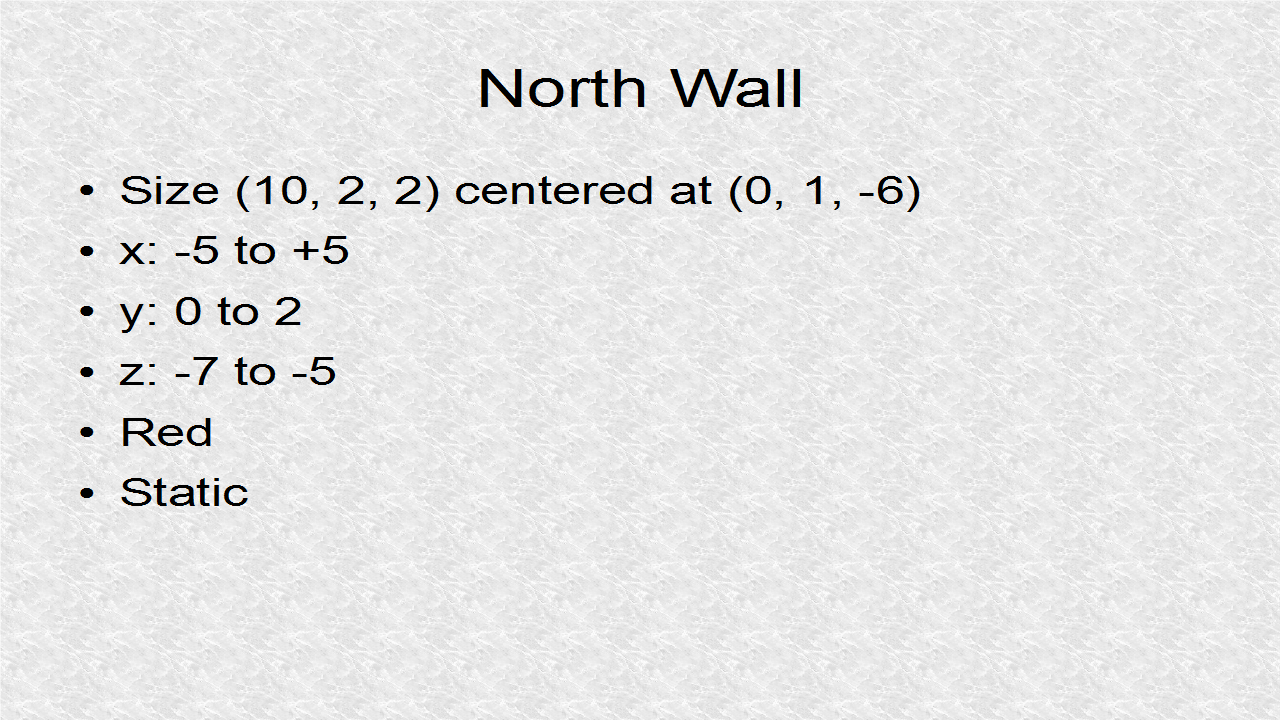
// *** 4. Start (North Wall) Box bWallN = new Box(5, 1, 1); Geometry geomWallN = new Geometry("WallN", bWallN); Material matWallN = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matWallN.setColor("Color", ColorRGBA.Red); geomWallN.setLocalTranslation(0, 1, -6); geomWallN.setMaterial(matWallN); rootNode.attachChild(geomWallN); RigidBodyControl wallNPhy = new RigidBodyControl(0.0f); geomWallN.addControl(wallNPhy); bulletAppState.getPhysicsSpace().add(wallNPhy); // *** 4. End
The South Wall is also a static Box mesh object.
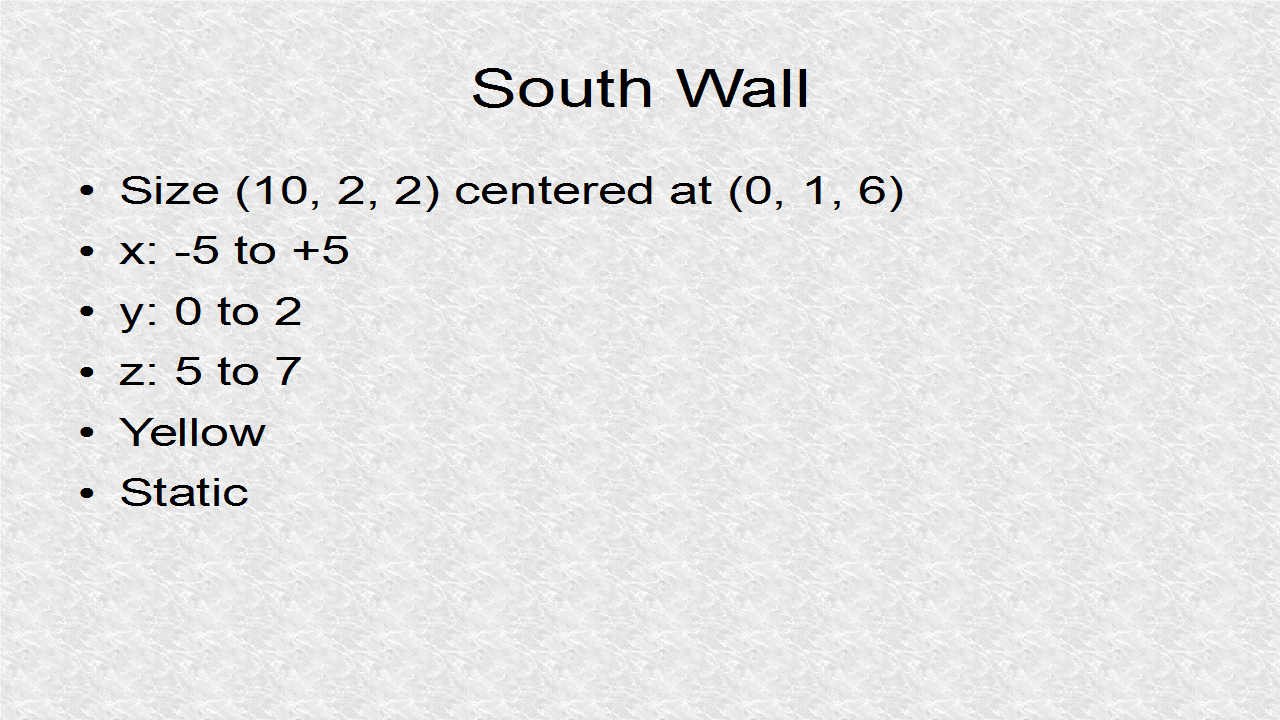
// *** 5. Start (South Wall) Box bWallS = new Box(5, 1, 1); Geometry geomWallS = new Geometry("WallS", bWallS); Material matWallS = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matWallS.setColor("Color", ColorRGBA.Yellow); geomWallS.setLocalTranslation(0, 1, 6); geomWallS.setMaterial(matWallS); rootNode.attachChild(geomWallS); RigidBodyControl wallSPhy = new RigidBodyControl(0.0f); geomWallS.addControl(wallSPhy); bulletAppState.getPhysicsSpace().add(wallSPhy); // *** 5. End
The Worth Wall is also a static Box mesh object.
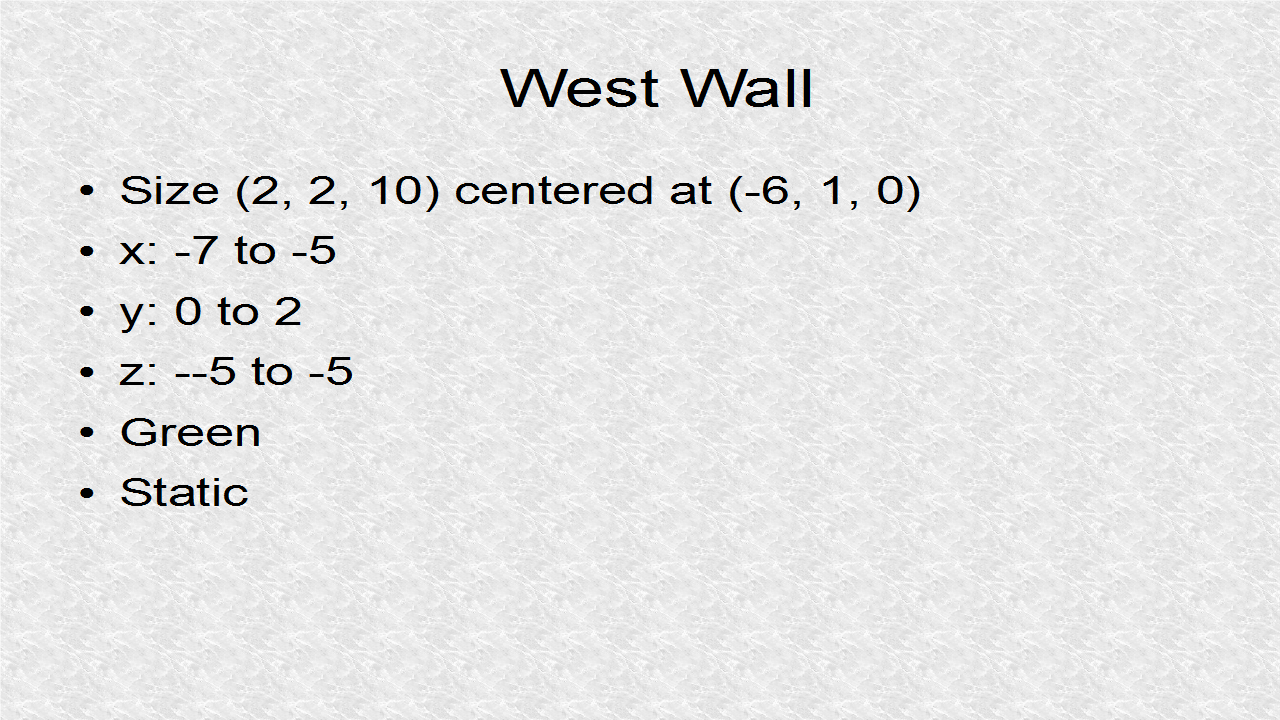
// *** 6. Start (West Wall) Box bWallW = new Box(1, 1, 5); Geometry geomWallW = new Geometry("WallW", bWallW); Material matWallW = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matWallW.setColor("Color", ColorRGBA.Green); geomWallW.setLocalTranslation(-6, 1, 0); geomWallW.setMaterial(matWallW); rootNode.attachChild(geomWallW); RigidBodyControl wallWPhy = new RigidBodyControl(0.0f); geomWallW.addControl(wallWPhy); bulletAppState.getPhysicsSpace().add(wallWPhy); // *** 6. End
Finally, the East Wall is also a static Box mesh object.
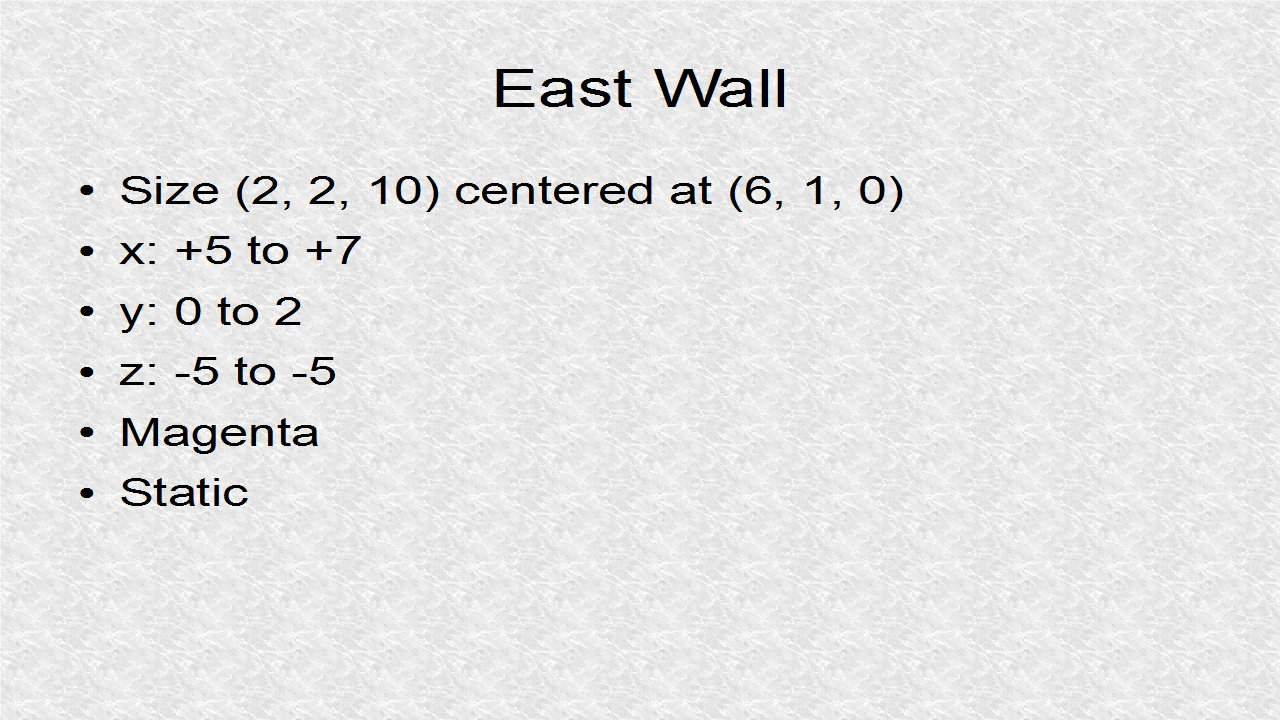
// *** 7. Start (East Wall) Box bWallE = new Box(1, 1, 5); Geometry geomWallE = new Geometry("WallE", bWallE); Material matWallE = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matWallE.setColor("Color", ColorRGBA.Magenta); geomWallE.setLocalTranslation(6, 1, 0); geomWallE.setMaterial(matWallE); rootNode.attachChild(geomWallE); RigidBodyControl wallEPhy = new RigidBodyControl(0.0f); geomWallE.addControl(wallEPhy); bulletAppState.getPhysicsSpace().add(wallEPhy); // *** 7. End
ball1 is a Sphere mesh object and is a BetterCharacterControl object.
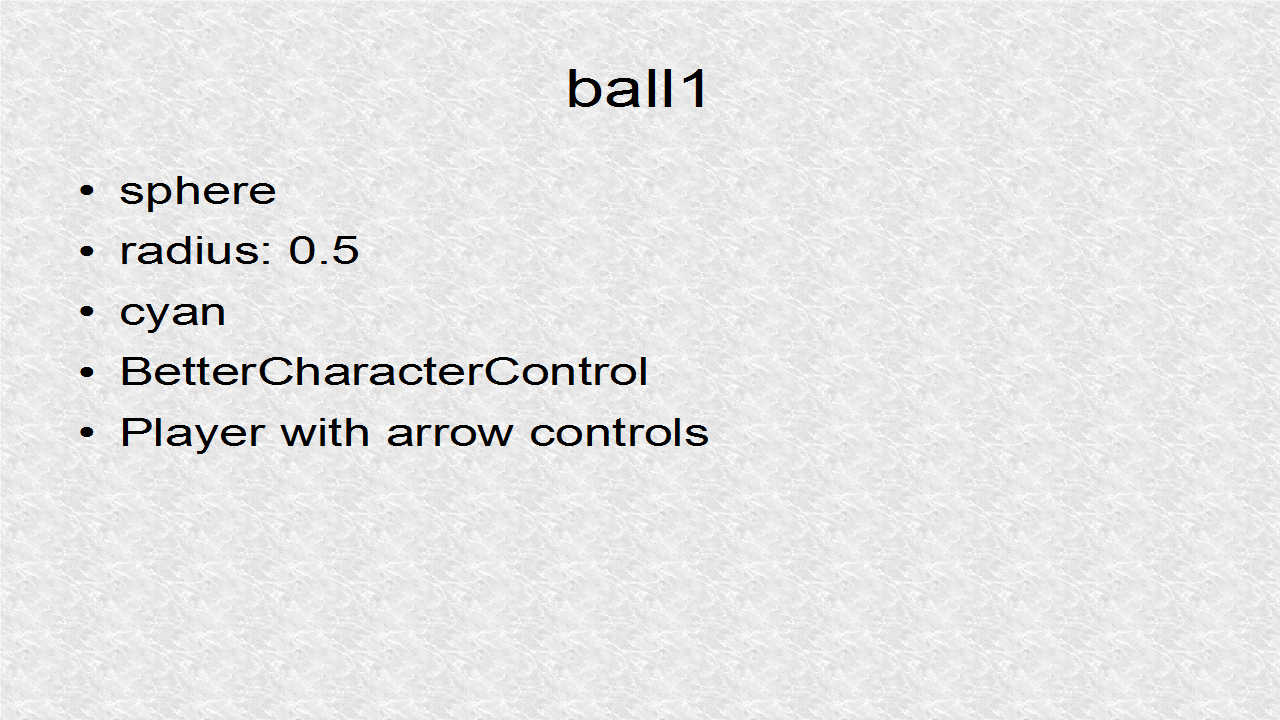
// *** 8. Start (ball1 - Player) Sphere ball1 = new Sphere (16,16,0.5f); geomBall1 = new Geometry("ball1", ball1); Material matBall1 = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matBall1.setColor("Color", ColorRGBA.Cyan); geomBall1.setLocalTranslation(0, 2, 0); geomBall1.setMaterial(matBall1); rootNode.attachChild(geomBall1); ball1Phy = new BetterCharacterControl(0.5f,5f,30f); geomBall1.addControl(ball1Phy); bulletAppState.getPhysicsSpace().add(ball1Phy); // *** 8. End
ball2 is a Sphere mesh object. It is RigidBodyControl with nonzero mass.
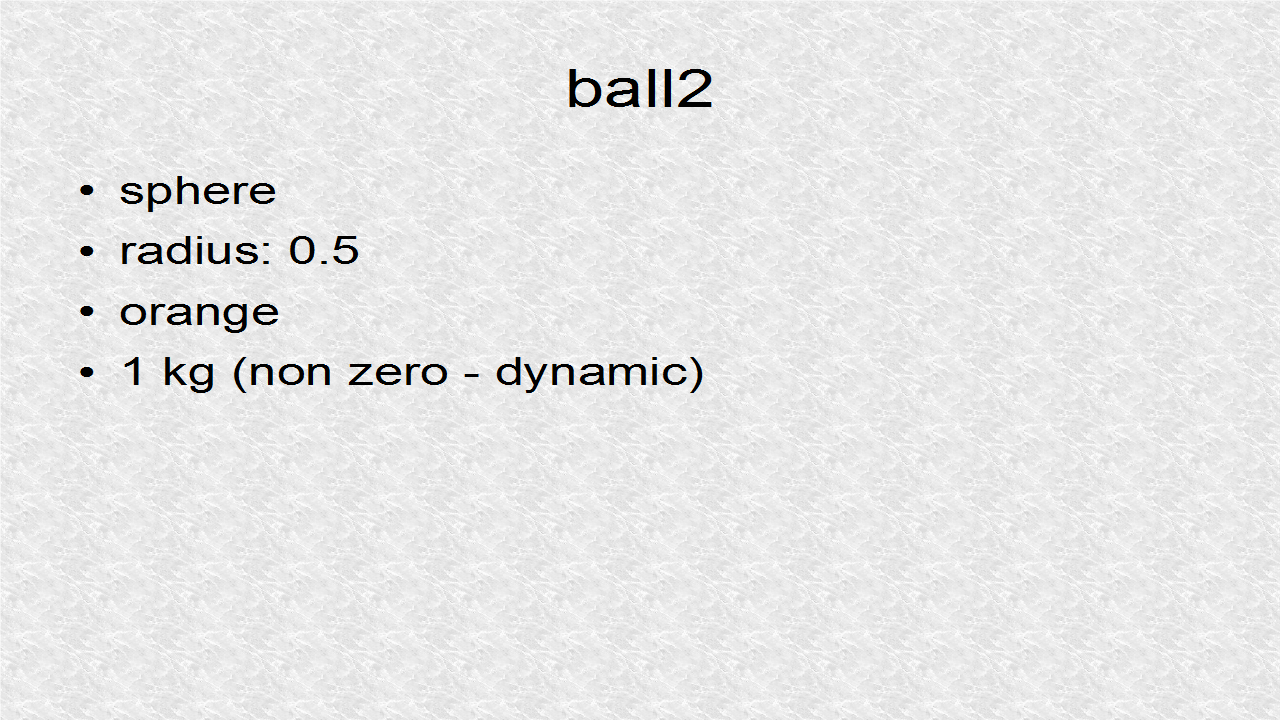
// *** 9. Start (ball2 - Can move) Sphere ball2 = new Sphere (16,16,0.5f); Geometry geomBall2 = new Geometry("ball2", ball2); Material matBall2 = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matBall2.setColor("Color", ColorRGBA.Orange); geomBall2.setLocalTranslation(2, 2, 1.5f); geomBall2.setMaterial(matBall2); rootNode.attachChild(geomBall2); RigidBodyControl ball2Phy = new RigidBodyControl(1); geomBall2.addControl(ball2Phy); bulletAppState.getPhysicsSpace().add(ball2Phy); // *** 9. End
ball3 is a Sphere mesh object. It is RigidBodyControl with nonzero mass.
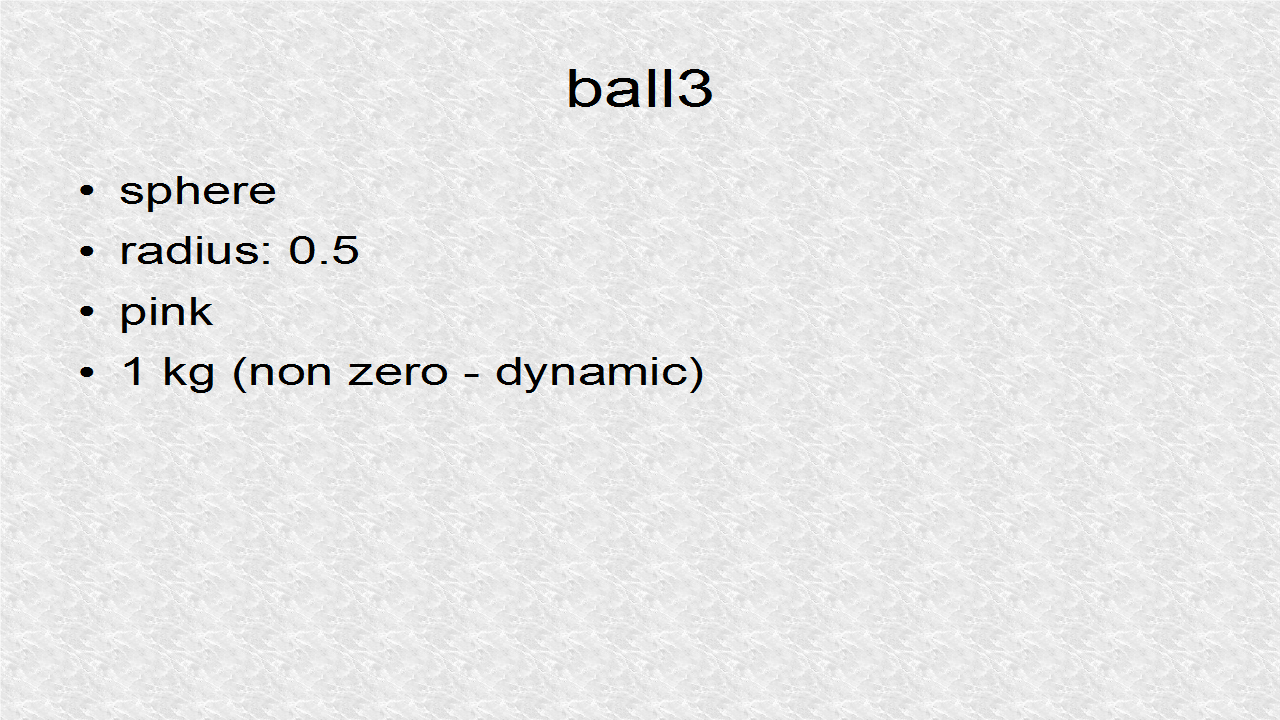
// *** 10. Start (ball2 - Can move) Sphere ball3 = new Sphere (16,16,0.5f); Geometry geomBall3 = new Geometry("ball3", ball3); Material matBall3 = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matBall3.setColor("Color", ColorRGBA.Pink); geomBall3.setLocalTranslation(-2, 2, -1.5f); geomBall3.setMaterial(matBall3); rootNode.attachChild(geomBall3); RigidBodyControl ball3Phy = new RigidBodyControl(1); geomBall3.addControl(ball3Phy); bulletAppState.getPhysicsSpace().add(ball3Phy); // *** 10. End
// JMonkey51.java package mygame; import com.jme3.app.SimpleApplication; import com.jme3.bullet.BulletAppState; import com.jme3.bullet.control.BetterCharacterControl; import com.jme3.bullet.control.RigidBodyControl; import com.jme3.input.KeyInput; import com.jme3.input.controls.ActionListener; import com.jme3.input.controls.KeyTrigger; import com.jme3.material.Material; import com.jme3.math.ColorRGBA; import com.jme3.math.Vector3f; import com.jme3.renderer.RenderManager; import com.jme3.scene.Geometry; import com.jme3.scene.shape.Box; import com.jme3.scene.shape.Sphere; public class JMonkey51 extends SimpleApplication { public static void main(String[] args) { JMonkey51 app = new JMonkey51(); app.start(); } Geometry geomBall1; // Player Geometry private BulletAppState bulletAppState; // Physics private BetterCharacterControl ball1Phy; // Player private Vector3f walkDirection = new Vector3f(); private boolean left = false, right = false, up = false, down = false; @Override public void simpleInitApp() { flyCam.setEnabled(false); viewPort.setBackgroundColor(ColorRGBA.LightGray); setDisplayFps(false); setDisplayStatView(false); // *** 1. Start (Camera +y looking down y) cam.setAxes(new Vector3f(-1,0,0), // left new Vector3f(0,0,-1), // up new Vector3f(0,-1,0)); // dir cam.setLocation(new Vector3f(0,20,0)); // above ground cam.update(); // *** 1. End // *** 2. Start (Set Physics) bulletAppState = new BulletAppState(); stateManager.attach(bulletAppState); // *** 2. End // *** 3. Start (Floor) Box bFloor = new Box(5, 1, 5); Geometry geomFloor = new Geometry("Floor", bFloor); Material matFloor = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matFloor.setColor("Color", ColorRGBA.Blue); geomFloor.setMaterial(matFloor); rootNode.attachChild(geomFloor); RigidBodyControl floorPhy = new RigidBodyControl(0.0f); geomFloor.addControl(floorPhy); bulletAppState.getPhysicsSpace().add(floorPhy); // *** 3. End // *** 4. Start (North Wall) Box bWallN = new Box(5, 1, 1); Geometry geomWallN = new Geometry("WallN", bWallN); Material matWallN = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matWallN.setColor("Color", ColorRGBA.Red); geomWallN.setLocalTranslation(0, 1, -6); geomWallN.setMaterial(matWallN); rootNode.attachChild(geomWallN); RigidBodyControl wallNPhy = new RigidBodyControl(0.0f); geomWallN.addControl(wallNPhy); bulletAppState.getPhysicsSpace().add(wallNPhy); // *** 4. End // *** 5. Start (South Wall) Box bWallS = new Box(5, 1, 1); Geometry geomWallS = new Geometry("WallS", bWallS); Material matWallS = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matWallS.setColor("Color", ColorRGBA.Yellow); geomWallS.setLocalTranslation(0, 1, 6); geomWallS.setMaterial(matWallS); rootNode.attachChild(geomWallS); RigidBodyControl wallSPhy = new RigidBodyControl(0.0f); geomWallS.addControl(wallSPhy); bulletAppState.getPhysicsSpace().add(wallSPhy); // *** 5. End // *** 6. Start (West Wall) Box bWallW = new Box(1, 1, 5); Geometry geomWallW = new Geometry("WallW", bWallW); Material matWallW = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matWallW.setColor("Color", ColorRGBA.Green); geomWallW.setLocalTranslation(-6, 1, 0); geomWallW.setMaterial(matWallW); rootNode.attachChild(geomWallW); RigidBodyControl wallWPhy = new RigidBodyControl(0.0f); geomWallW.addControl(wallWPhy); bulletAppState.getPhysicsSpace().add(wallWPhy); // *** 6. End // *** 7. Start (East Wall) Box bWallE = new Box(1, 1, 5); Geometry geomWallE = new Geometry("WallE", bWallE); Material matWallE = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matWallE.setColor("Color", ColorRGBA.Magenta); geomWallE.setLocalTranslation(6, 1, 0); geomWallE.setMaterial(matWallE); rootNode.attachChild(geomWallE); RigidBodyControl wallEPhy = new RigidBodyControl(0.0f); geomWallE.addControl(wallEPhy); bulletAppState.getPhysicsSpace().add(wallEPhy); // *** 7. End // *** 8. Start (ball1 - Player) Sphere ball1 = new Sphere (16,16,0.5f); geomBall1 = new Geometry("ball1", ball1); Material matBall1 = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matBall1.setColor("Color", ColorRGBA.Cyan); geomBall1.setLocalTranslation(0, 2, 0); geomBall1.setMaterial(matBall1); rootNode.attachChild(geomBall1); ball1Phy = new BetterCharacterControl(0.5f,5f,30f); geomBall1.addControl(ball1Phy); bulletAppState.getPhysicsSpace().add(ball1Phy); // *** 8. End // *** 9. Start (ball2 - Can move) Sphere ball2 = new Sphere (16,16,0.5f); Geometry geomBall2 = new Geometry("ball2", ball2); Material matBall2 = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matBall2.setColor("Color", ColorRGBA.Orange); geomBall2.setLocalTranslation(2, 2, 1.5f); geomBall2.setMaterial(matBall2); rootNode.attachChild(geomBall2); RigidBodyControl ball2Phy = new RigidBodyControl(1); geomBall2.addControl(ball2Phy); bulletAppState.getPhysicsSpace().add(ball2Phy); // *** 9. End // *** 10. Start (ball2 - Can move) Sphere ball3 = new Sphere (16,16,0.5f); Geometry geomBall3 = new Geometry("ball3", ball3); Material matBall3 = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matBall3.setColor("Color", ColorRGBA.Pink); geomBall3.setLocalTranslation(-2, 2, -1.5f); geomBall3.setMaterial(matBall3); rootNode.attachChild(geomBall3); RigidBodyControl ball3Phy = new RigidBodyControl(1); geomBall3.addControl(ball3Phy); bulletAppState.getPhysicsSpace().add(ball3Phy); // *** 10. End setupKeys(); } private void setupKeys() { inputManager.addMapping("Left", new KeyTrigger(KeyInput.KEY_LEFT)); inputManager.addMapping("Right", new KeyTrigger(KeyInput.KEY_RIGHT)); inputManager.addMapping("Up", new KeyTrigger(KeyInput.KEY_UP)); inputManager.addMapping("Down", new KeyTrigger(KeyInput.KEY_DOWN)); inputManager.addListener(actionListener, "Left","Right","Up","Down"); } private ActionListener actionListener = new ActionListener() { public void onAction(String binding, boolean isPressed, float tpf) { if (binding.equals("Left")) { left = isPressed; } else if (binding.equals("Right")) { right= isPressed; } else if (binding.equals("Up")) { up = isPressed; } else if (binding.equals("Down")) { down = isPressed; } } }; @Override public void simpleUpdate(float tpf) { walkDirection.set(0, 0, 0); if (left) { walkDirection.addLocal(-10f,0,0); } else if (right) { walkDirection.addLocal(10f,0,0); } if (up) { walkDirection.addLocal(0,0,-10f); } else if (down) { walkDirection.addLocal(0,0,+10f); } ball1Phy.setWalkDirection(walkDirection); } @Override public void simpleRender(RenderManager rm) { } }
Output:
No comments:
Post a Comment