Two multiplications between vectors are dot product and cross product.
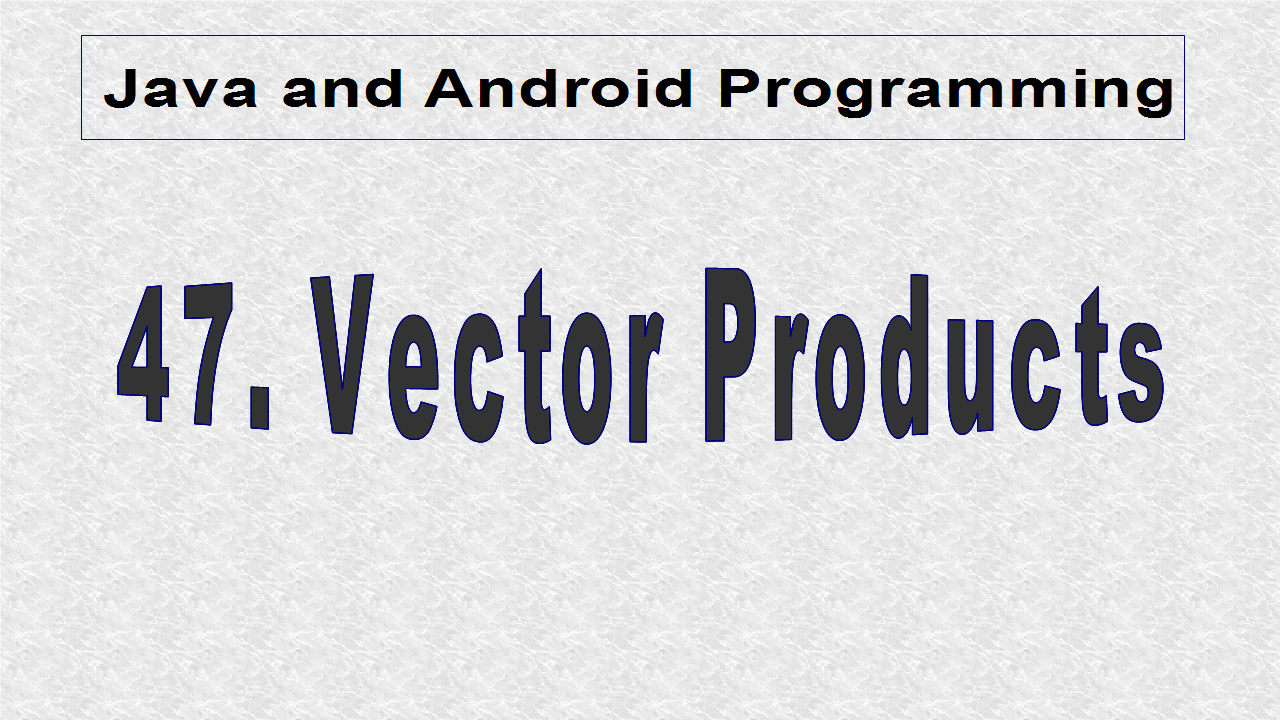
The example vectors are on the x-y plane, with vecA being 45 degrees below the +x axis and vecB 45 degrees above the +x axis.
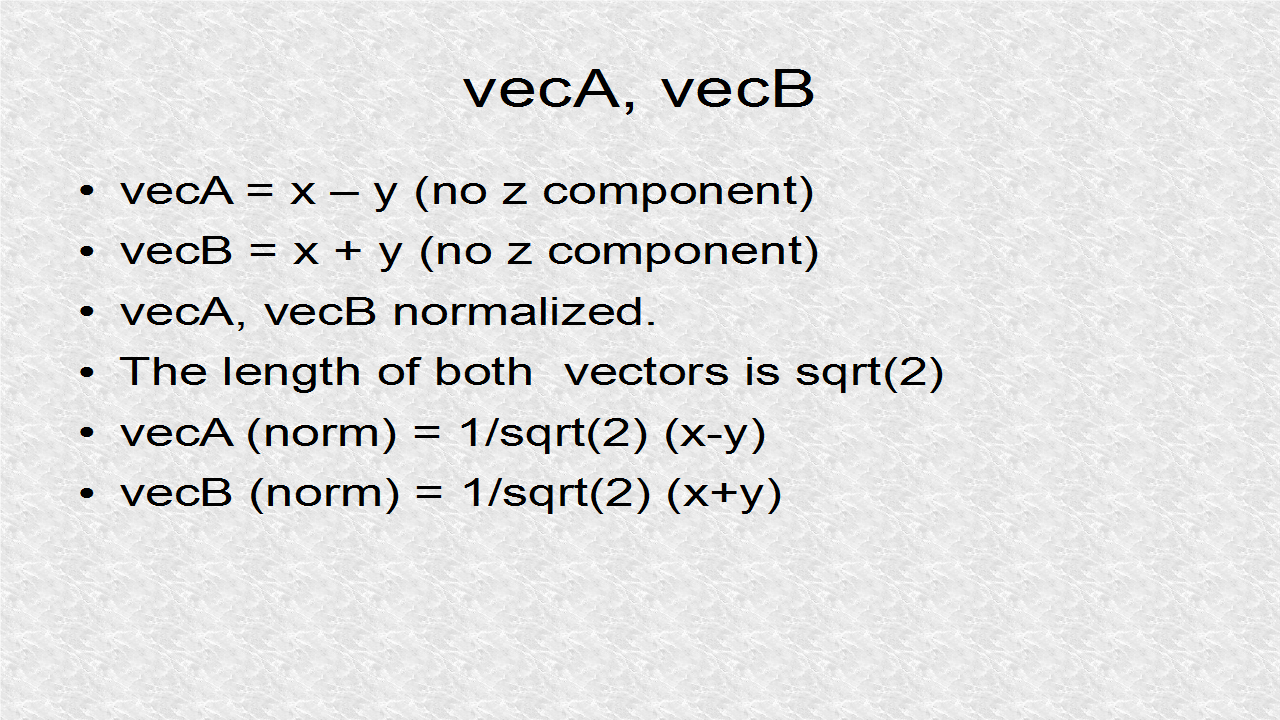
// *** 1. Start (vectors x-y, x+y, both in xy plane) Vector3f vecA = new Vector3f(1,-1,0); // x-y Vector3f vecB = new Vector3f(1,1,0); // x+y vecA.normalizeLocal(); // normalize them vecB.normalizeLocal(); // |vecA|=|vecB|=1 // *** 1. End
With the dot product, we can find the angle between two vectors.
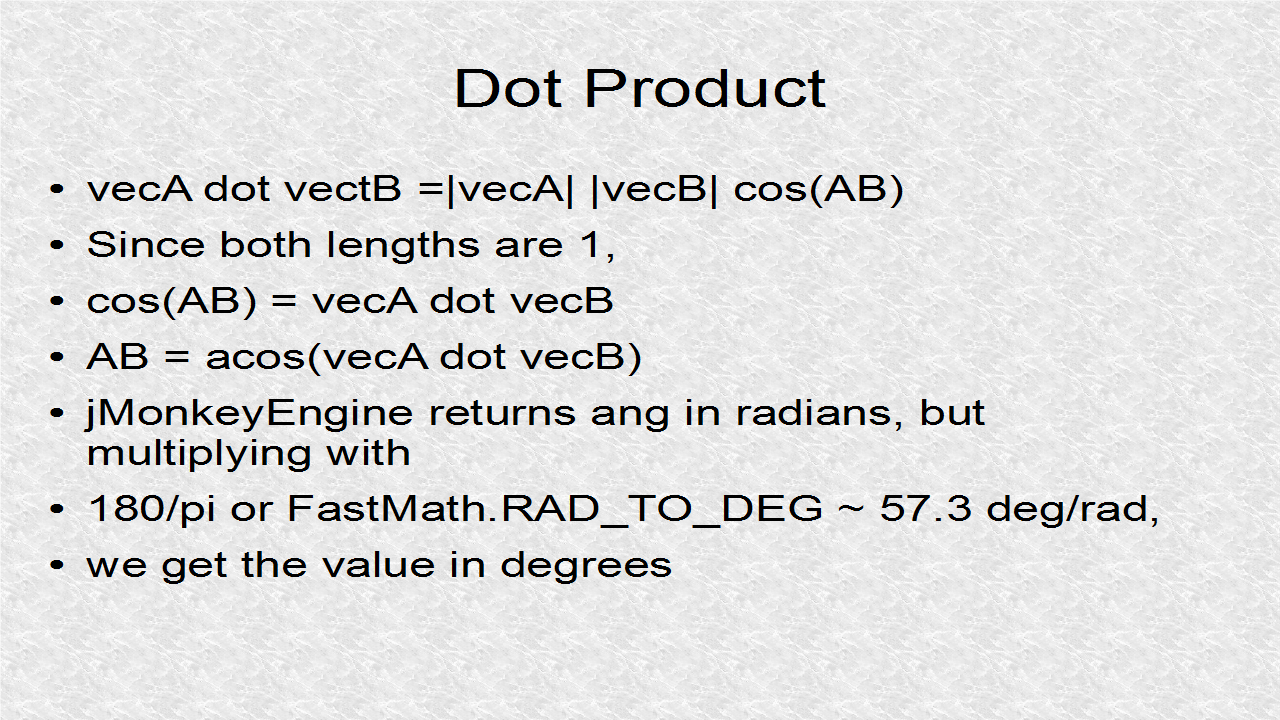
// *** 2. Start (dot product to find ang) float dot = vecA.dot(vecB); // vecA.vecB = |vecA| |vecB| cos(AB) = cos(AB) float angRad = FastMath.acos(dot); float ang = FastMath.RAD_TO_DEG*angRad; // 90 deg System.out.println("vecA = " + vecA); System.out.println("vecB = " + vecB); System.out.println("dot = " + dot); System.out.println("ang = " + ang); // *** 2. End
With the cross product, we can find the angle between two vectors, as well as the plane normal.
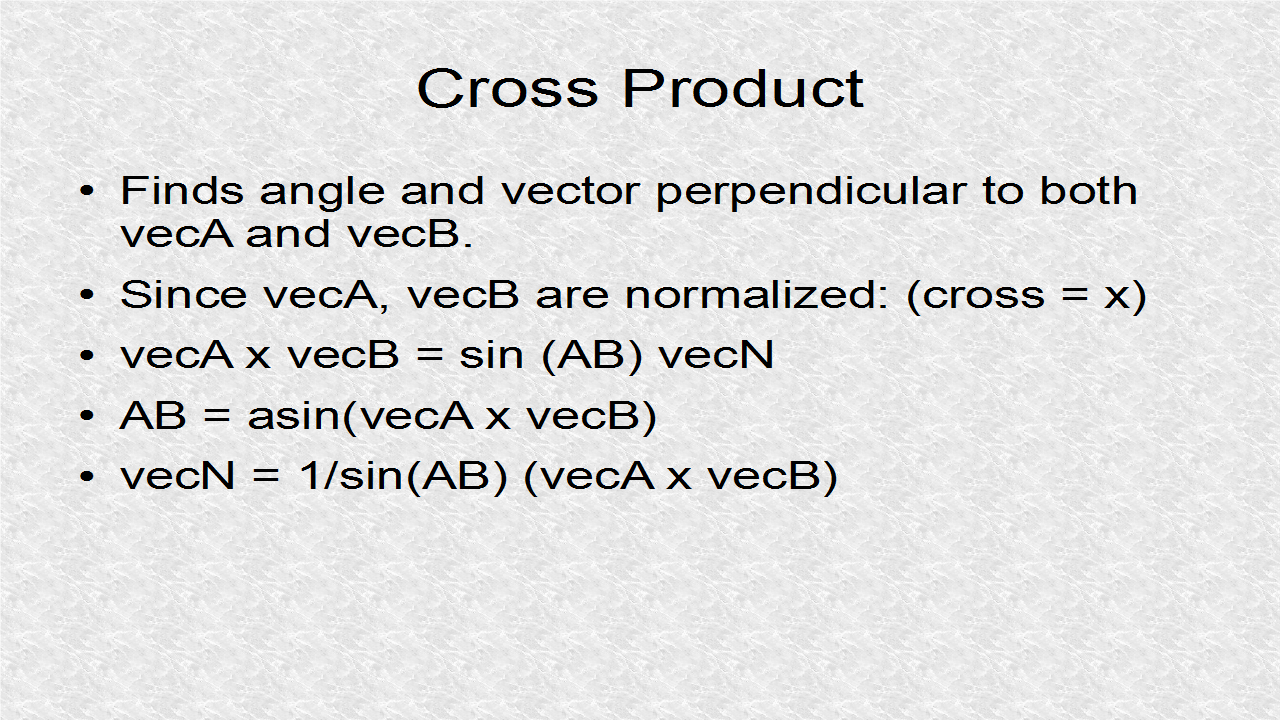
// *** 3. Start (cross product to find Ang, vecN) // vecN in z since vecA,vecB in x,y plane // direction of vecN depends on right-hand rule Vector3f cross = vecA.cross(vecB); // vecA x vecB = |vecA| |vecB| sin(AB) vecN // vecA x vecB = sin(AB) vecN float len = cross.length(); float angRad1 = FastMath.asin(len); float ang1 = FastMath.RAD_TO_DEG*angRad1; // 90 deg Vector3f vecN = new Vector3f(); // z vecN = cross.mult(1/len); System.out.println("cross = " + cross); System.out.println("ang1 = " + ang1); System.out.println("vecN = " + vecN); // *** 3. End
Three arrows are drawn. Two for the two example vectors, as well as the normal to the plane, formed by the 2 example vectors.
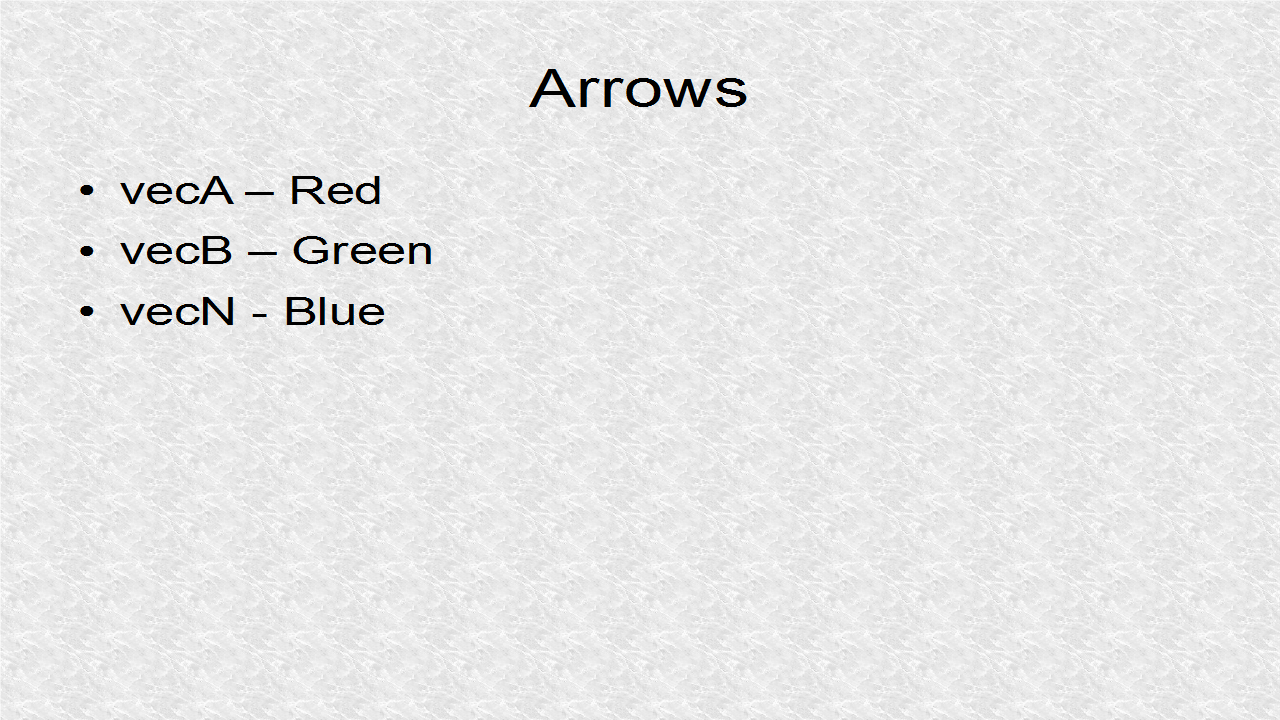
// *** 4. Starts (arrows for vecA, vecB, vecN) Arrow arrowA = new Arrow(vecA); arrowA.setLineWidth(4); Geometry geoArrowA = new Geometry("geoArrowA",arrowA); Material matArrowA = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matArrowA.setColor("Color", ColorRGBA.Red); geoArrowA.setMaterial(matArrowA); rootNode.attachChild(geoArrowA); Arrow arrowB = new Arrow(vecB); arrowB.setLineWidth(4); Geometry geoArrowB = new Geometry("geoArrowB",arrowB); Material matArrowB = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matArrowB.setColor("Color", ColorRGBA.Green); geoArrowB.setMaterial(matArrowB); rootNode.attachChild(geoArrowB); Arrow arrowN = new Arrow(vecN); arrowN.setLineWidth(4); Geometry geoArrowN = new Geometry("geoArrowN",arrowN); Material matArrowN = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matArrowN.setColor("Color", ColorRGBA.Blue); geoArrowN.setMaterial(matArrowN); rootNode.attachChild(geoArrowN); // *** 4. End
// JMonkey47.java package mygame; import com.jme3.app.SimpleApplication; import com.jme3.material.Material; import com.jme3.math.ColorRGBA; import com.jme3.math.FastMath; import com.jme3.math.Vector3f; import com.jme3.renderer.RenderManager; import com.jme3.scene.Geometry; import com.jme3.scene.debug.Arrow; import com.jme3.system.AppSettings; public class JMonkey47 extends SimpleApplication { public static void main(String[] args) { AppSettings settings = new AppSettings(true); settings.setFrameRate(60); settings.setTitle("Multiplying Vectors !"); JMonkey47 app = new JMonkey47(); app.setSettings(settings); app.start(); } @Override public void simpleInitApp() { flyCam.setEnabled(false); viewPort.setBackgroundColor(ColorRGBA.White); // *** 1. Start (vectors x-y, x+y, both in xy plane) Vector3f vecA = new Vector3f(1,-1,0); // x-y Vector3f vecB = new Vector3f(1,1,0); // x+y vecA.normalizeLocal(); // normalize them vecB.normalizeLocal(); // |vecA|=|vecB|=1 // *** 1. End // *** 2. Start (dot product to find ang) float dot = vecA.dot(vecB); // vecA.vecB = |vecA| |vecB| cos(AB) = cos(AB) float angRad = FastMath.acos(dot); float ang = FastMath.RAD_TO_DEG*angRad; // 90 deg System.out.println("vecA = " + vecA); System.out.println("vecB = " + vecB); System.out.println("dot = " + dot); System.out.println("ang = " + ang); // *** 2. End // *** 3. Start (cross product to find Ang, vecN) // vecN in z since vecA,vecB in x,y plane // direction of vecN depends on right-hand rule Vector3f cross = vecA.cross(vecB); // vecA x vecB = |vecA| |vecB| sin(AB) vecN // vecA x vecB = sin(AB) vecN float len = cross.length(); float angRad1 = FastMath.asin(len); float ang1 = FastMath.RAD_TO_DEG*angRad1; // 90 deg Vector3f vecN = new Vector3f(); // z vecN = cross.mult(1/len); System.out.println("cross = " + cross); System.out.println("ang1 = " + ang1); System.out.println("vecN = " + vecN); // *** 3. End // *** 4. Starts (arrows for vecA, vecB, vecN) Arrow arrowA = new Arrow(vecA); arrowA.setLineWidth(4); Geometry geoArrowA = new Geometry("geoArrowA",arrowA); Material matArrowA = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matArrowA.setColor("Color", ColorRGBA.Red); geoArrowA.setMaterial(matArrowA); rootNode.attachChild(geoArrowA); Arrow arrowB = new Arrow(vecB); arrowB.setLineWidth(4); Geometry geoArrowB = new Geometry("geoArrowB",arrowB); Material matArrowB = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matArrowB.setColor("Color", ColorRGBA.Green); geoArrowB.setMaterial(matArrowB); rootNode.attachChild(geoArrowB); Arrow arrowN = new Arrow(vecN); arrowN.setLineWidth(4); Geometry geoArrowN = new Geometry("geoArrowN",arrowN); Material matArrowN = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); matArrowN.setColor("Color", ColorRGBA.Blue); geoArrowN.setMaterial(matArrowN); rootNode.attachChild(geoArrowN); // *** 4. End } @Override public void simpleUpdate(float tpf) { rootNode.rotate(0,tpf,0); } @Override public void simpleRender(RenderManager rm) { } }
Output:
No comments:
Post a Comment