Spatials can be under control of different influences.
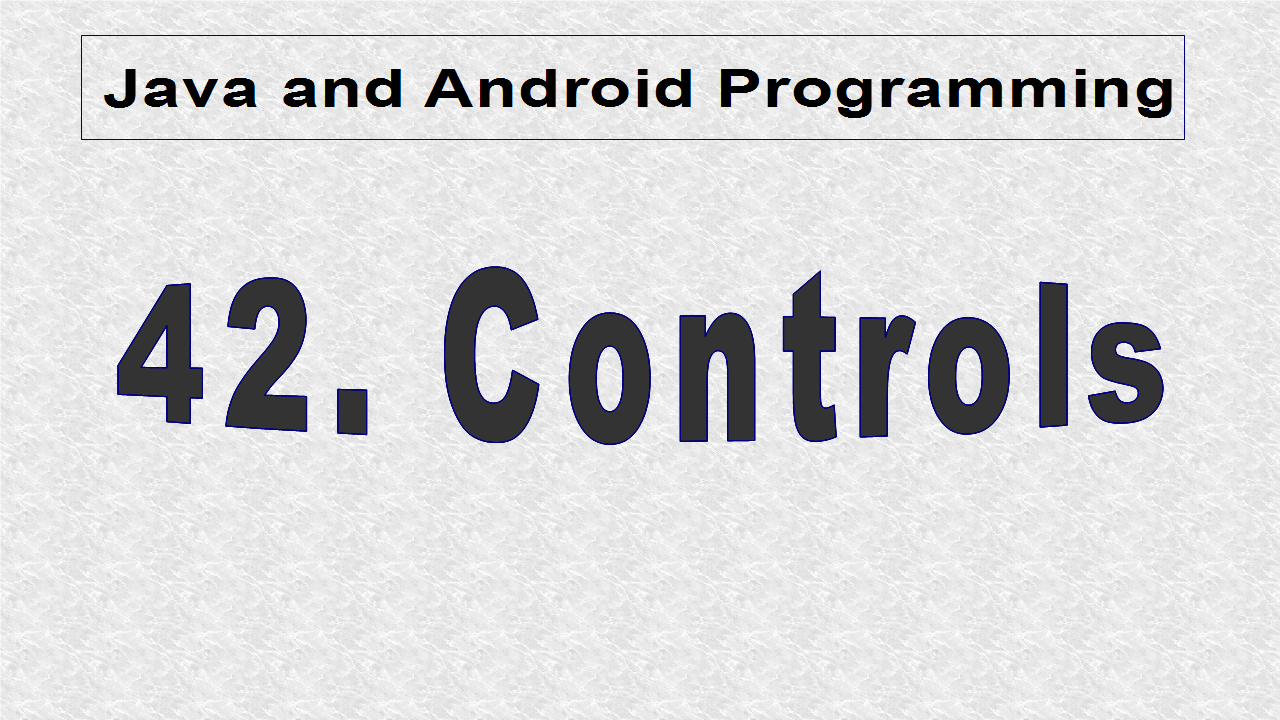
Here 3 state control classes are written. This is Control1.java with controlUpdate() rotating a spatial.
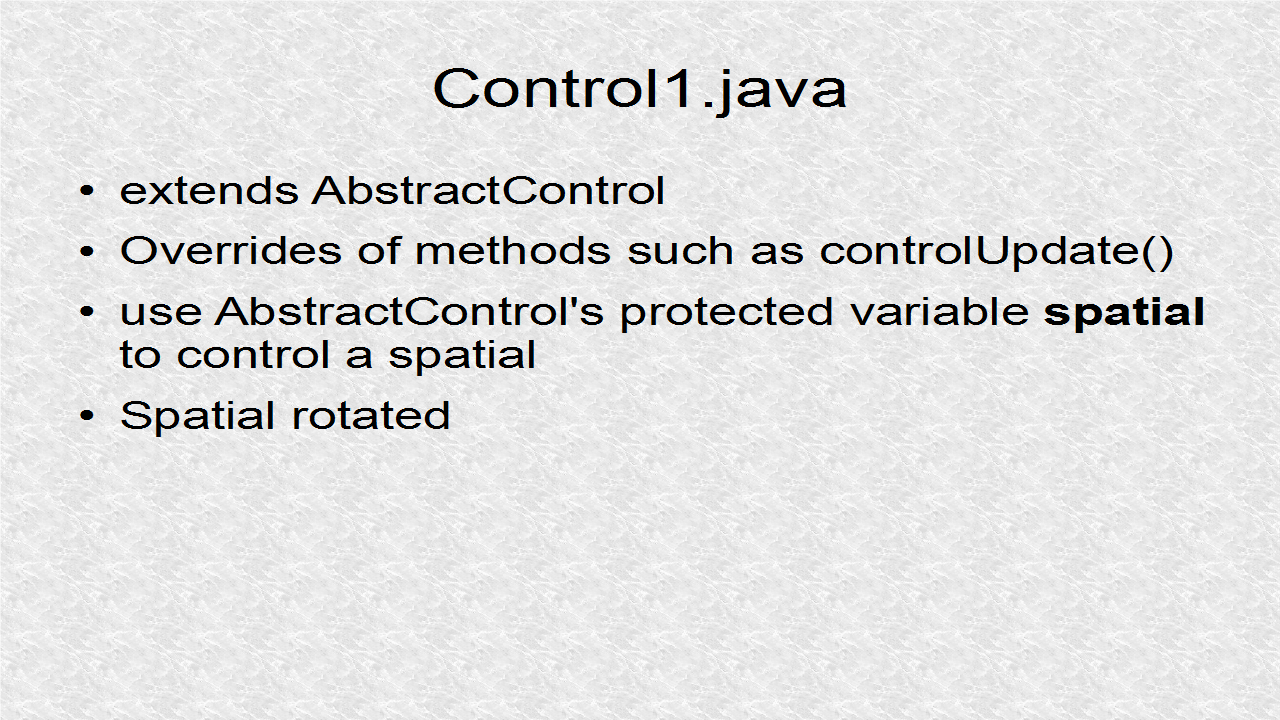
// Control1.java package mygame; import com.jme3.renderer.RenderManager; import com.jme3.renderer.ViewPort; import com.jme3.scene.control.AbstractControl; class Control1 extends AbstractControl{ @Override protected void controlUpdate(float tpf) { spatial.rotate(tpf,tpf,tpf); } @Override protected void controlRender(RenderManager rm, ViewPort vp) { } }
This is Control2.java with override for contorlUpdate(). It oscillates x motion.
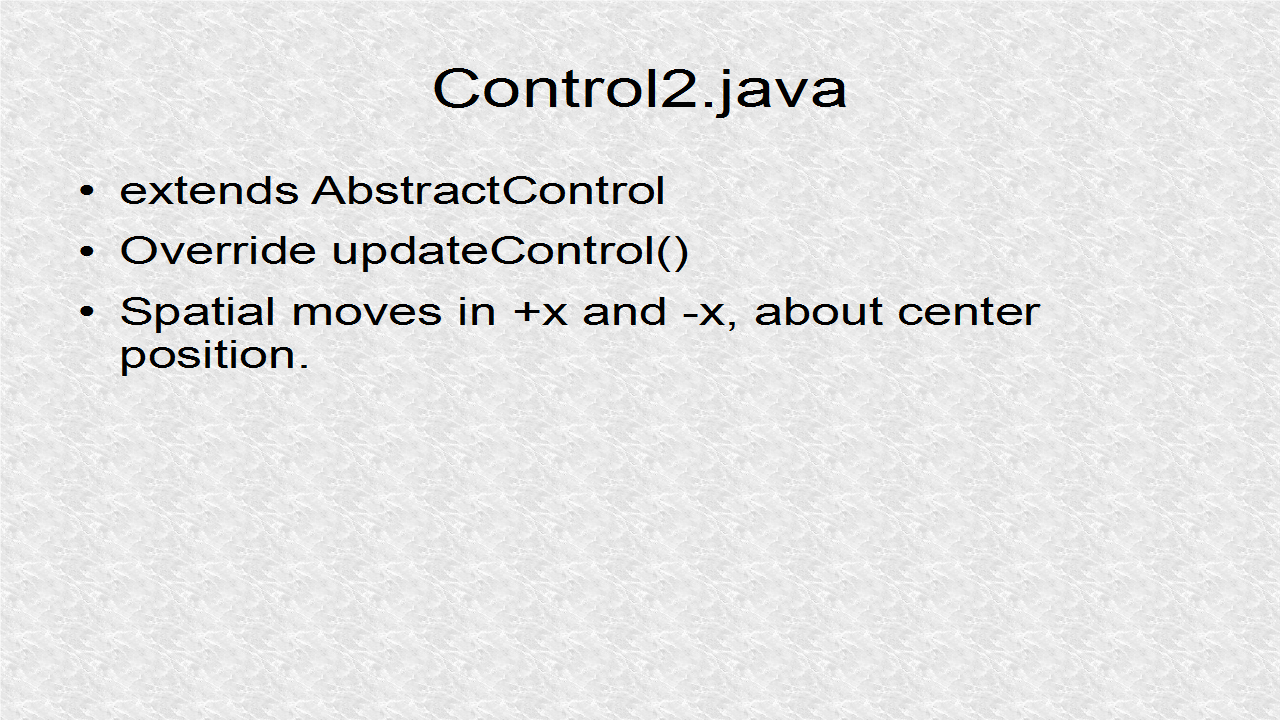
// Control2.java package mygame; import com.jme3.renderer.RenderManager; import com.jme3.renderer.ViewPort; import com.jme3.scene.control.AbstractControl; class Control2 extends AbstractControl{ private float current0, current; private int sign; public void Control2() { current0 = spatial.getLocalTranslation().x; } @Override protected void controlUpdate(float tpf) { current = spatial.getLocalTranslation().x; if ((current-current0)<-1f) { sign = 1; } if ((current-current0)>1f) { sign = -1; } spatial.move(sign*tpf,0,0); } @Override protected void controlRender(RenderManager rm, ViewPort vp) { } }
This is Control3.java with override for controlUpdate(). It oscillates y motion.
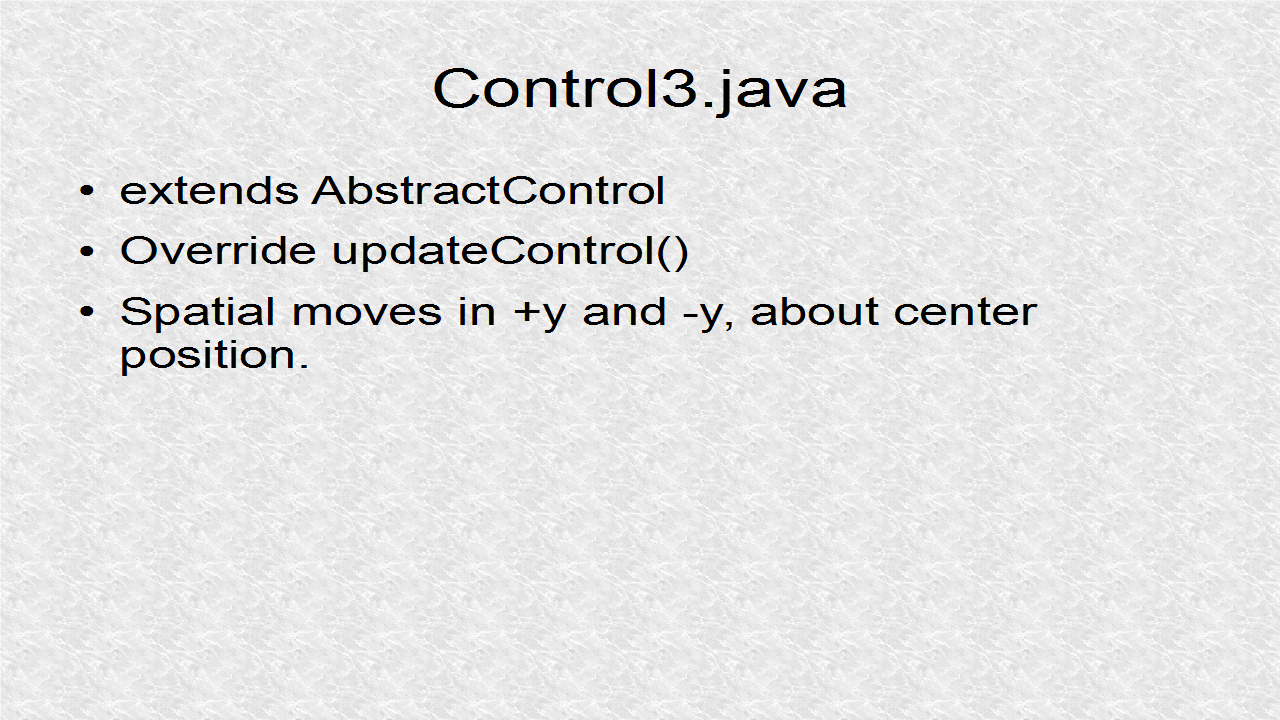
// Control3.java package mygame; import com.jme3.renderer.RenderManager; import com.jme3.renderer.ViewPort; import com.jme3.scene.control.AbstractControl; class Control3 extends AbstractControl { private float current0, current; private int sign; public void Control3() { current0 = spatial.getLocalTranslation().y; } @Override protected void controlUpdate(float tpf) { current = spatial.getLocalTranslation().y; if ((current-current0)<-1f) { sign = 1; } if ((current-current0)>1f) { sign = -1; } spatial.move(0,sign*tpf,0); } @Override protected void controlRender(RenderManager rm, ViewPort vp) { } }
We create spatial Geometries and then attach them to different controls.
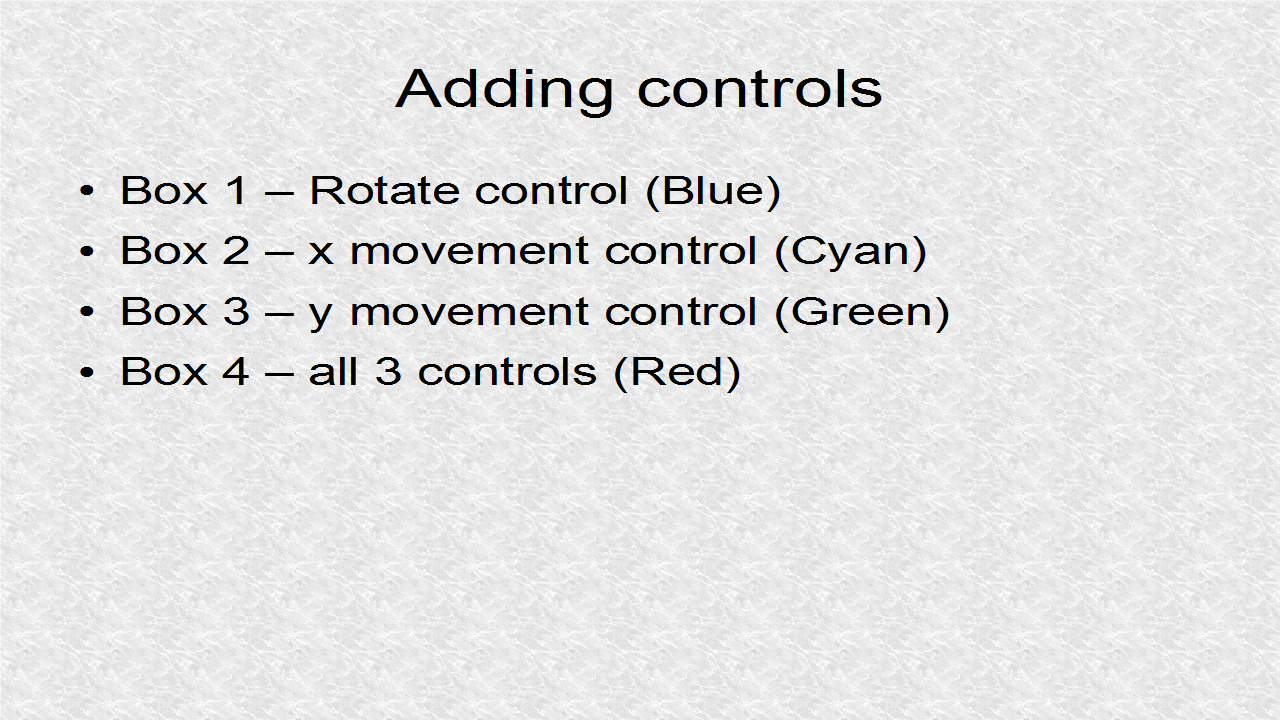
// *** 1. Start (adding controls to boxes) geom1 = makeGeometry( new Vector3f(.2f,.2f,.2f), new Vector3f(0,0,0)); geom1.addControl(control1a); // Control1 geom2 = makeGeometry( new Vector3f(.2f,.2f,.2f), new Vector3f(2.5f,2.5f,0)); geom2.addControl(control2a); // Control2 geom3 = makeGeometry( new Vector3f(.2f,.2f,.2f), new Vector3f(-2.5f,-2.5f,0)); geom3.addControl(control3a); // Control 3 geom4 = makeGeometry( new Vector3f(.5f,.5f,.5f), new Vector3f(2,-2,-1)); geom4.addControl(control1b); geom4.addControl(control3b); geom4.addControl(control2b); // All 3 Controls // *** 1. End
The spatial Geometries are created by this function.
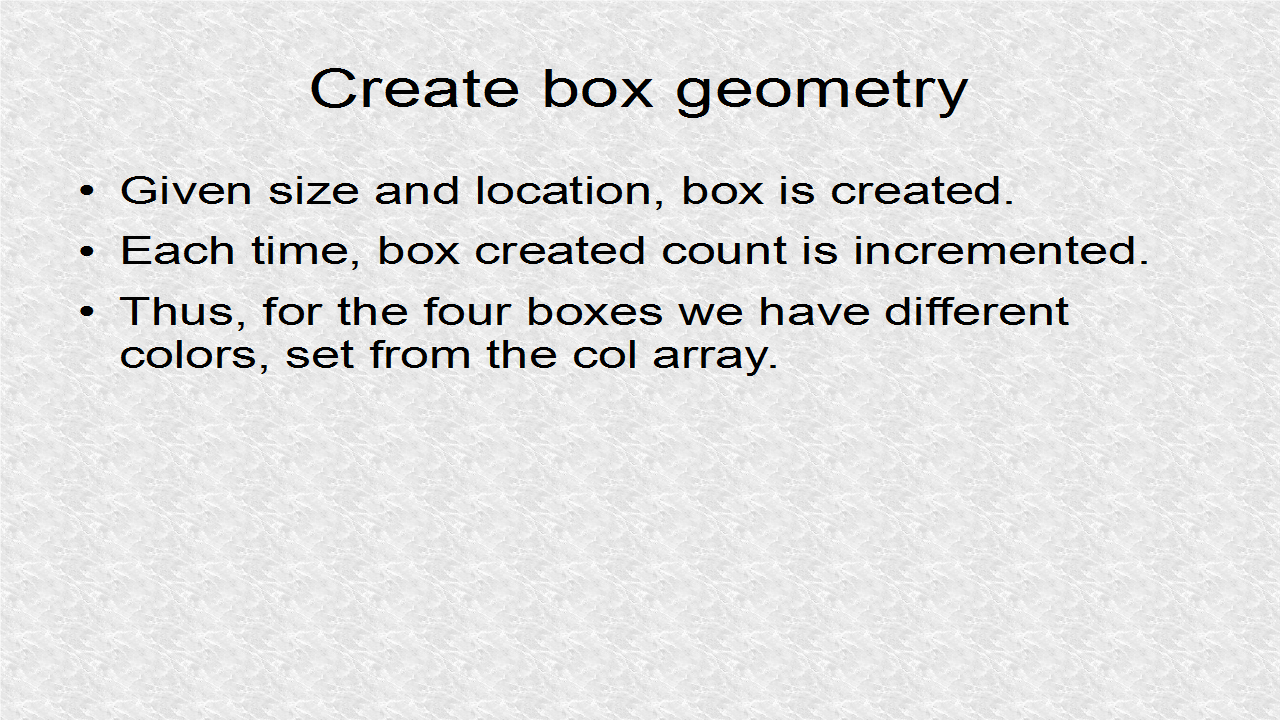
// *** 2. Start (Create box geometry given size + location) Box b = new Box(size.x, size.y, size.z); Geometry geom = new Geometry("Box", b); Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat.setColor("Color", col[count++]); geom.setMaterial(mat); geom.setLocalTranslation(loc); return geom; // *** 2. End
// JMonkey42.java package mygame; import com.jme3.app.SimpleApplication; import com.jme3.material.Material; import com.jme3.math.ColorRGBA; import com.jme3.math.Vector3f; import com.jme3.renderer.RenderManager; import com.jme3.scene.Geometry; import com.jme3.scene.shape.Box; import com.jme3.system.AppSettings; public class JMonkey42 extends SimpleApplication { public static void main(String[] args) { AppSettings settings = new AppSettings(true); settings.setFrameRate(60); settings.setTitle( "Boxes under different controls and multiple controls!"); JMonkey42 app = new JMonkey42(); app.setSettings(settings); app.start(); } Control1 control1a, control1b; Control2 control2a, control2b; Control3 control3a, control3b; Geometry geom1, geom2, geom3, geom4; final static ColorRGBA[] col = { ColorRGBA.Blue, ColorRGBA.Cyan, ColorRGBA.Green,ColorRGBA.Red}; // constant static int count = 0; // class variable @Override public void simpleInitApp() { viewPort.setBackgroundColor(ColorRGBA.White); setDisplayFps(false); setDisplayStatView(false); flyCam.setEnabled(false); control1a = new Control1(); control1b = new Control1(); control2a = new Control2(); control2b = new Control2(); control3a = new Control3(); control3b = new Control3(); // *** 1. Start (adding controls to boxes) geom1 = makeGeometry( new Vector3f(.2f,.2f,.2f), new Vector3f(0,0,0)); geom1.addControl(control1a); // Control1 geom2 = makeGeometry( new Vector3f(.2f,.2f,.2f), new Vector3f(2.5f,2.5f,0)); geom2.addControl(control2a); // Control2 geom3 = makeGeometry( new Vector3f(.2f,.2f,.2f), new Vector3f(-2.5f,-2.5f,0)); geom3.addControl(control3a); // Control 3 geom4 = makeGeometry( new Vector3f(.5f,.5f,.5f), new Vector3f(2,-2,-1)); geom4.addControl(control1b); geom4.addControl(control3b); geom4.addControl(control2b); // All 3 Controls // *** 1. End rootNode.attachChild(geom1); rootNode.attachChild(geom2); rootNode.attachChild(geom3); rootNode.attachChild(geom4); } private Geometry makeGeometry(Vector3f size, Vector3f loc) { // *** 2. Start (Create box geometry given size + location) Box b = new Box(size.x, size.y, size.z); Geometry geom = new Geometry("Box", b); Material mat = new Material(assetManager, "Common/MatDefs/Misc/Unshaded.j3md"); mat.setColor("Color", col[count++]); geom.setMaterial(mat); geom.setLocalTranslation(loc); return geom; // *** 2. End } @Override public void simpleUpdate(float tpf) { } @Override public void simpleRender(RenderManager rm) { } }
Output:
No comments:
Post a Comment