A simple stepped terrain is created.
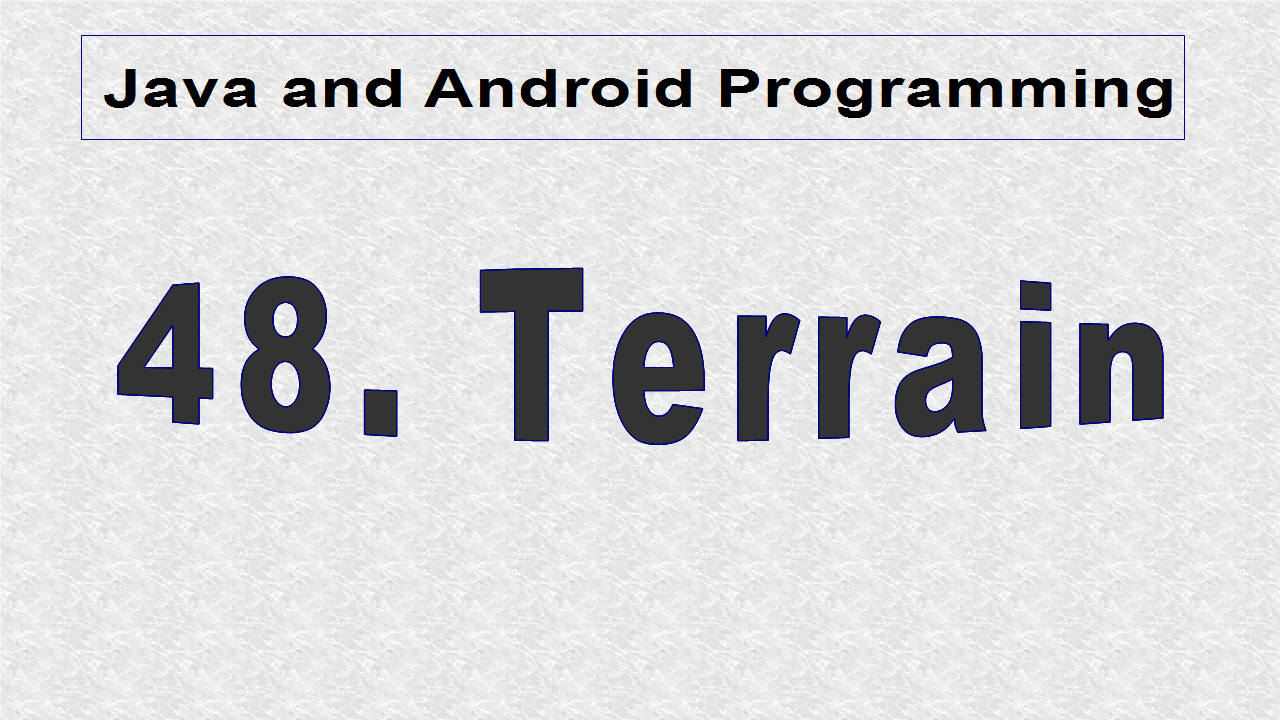
The terrain material is based on Terrain.j3md. This requires a few Image Textures. The Alpha gives weight of Red, Green, and Blue Textures. We also need a height map.
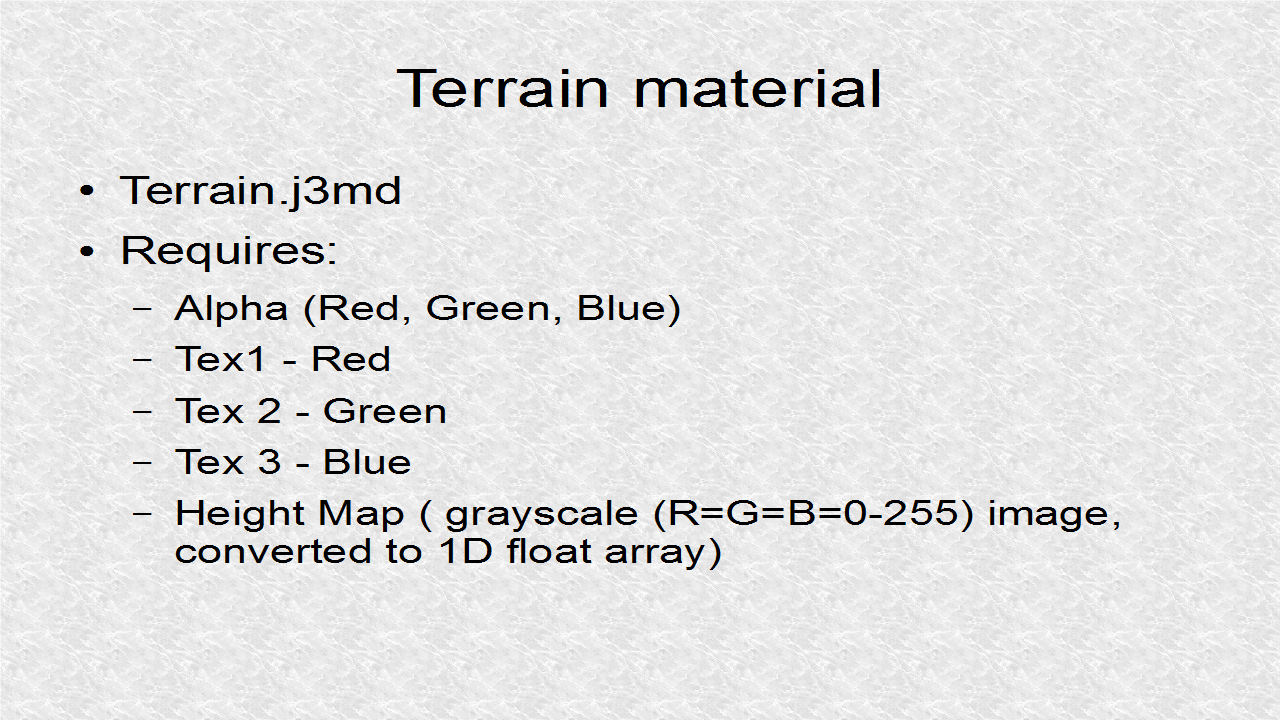
// *** 1. Start (Terrain Unshaded material) materialTerrain = new Material(assetManager, "Common/MatDefs/Terrain/Terrain.j3md"); // *** 1. End
This is a simple image for Alpha. The portion of terrain devoted to the three textures is about equal.
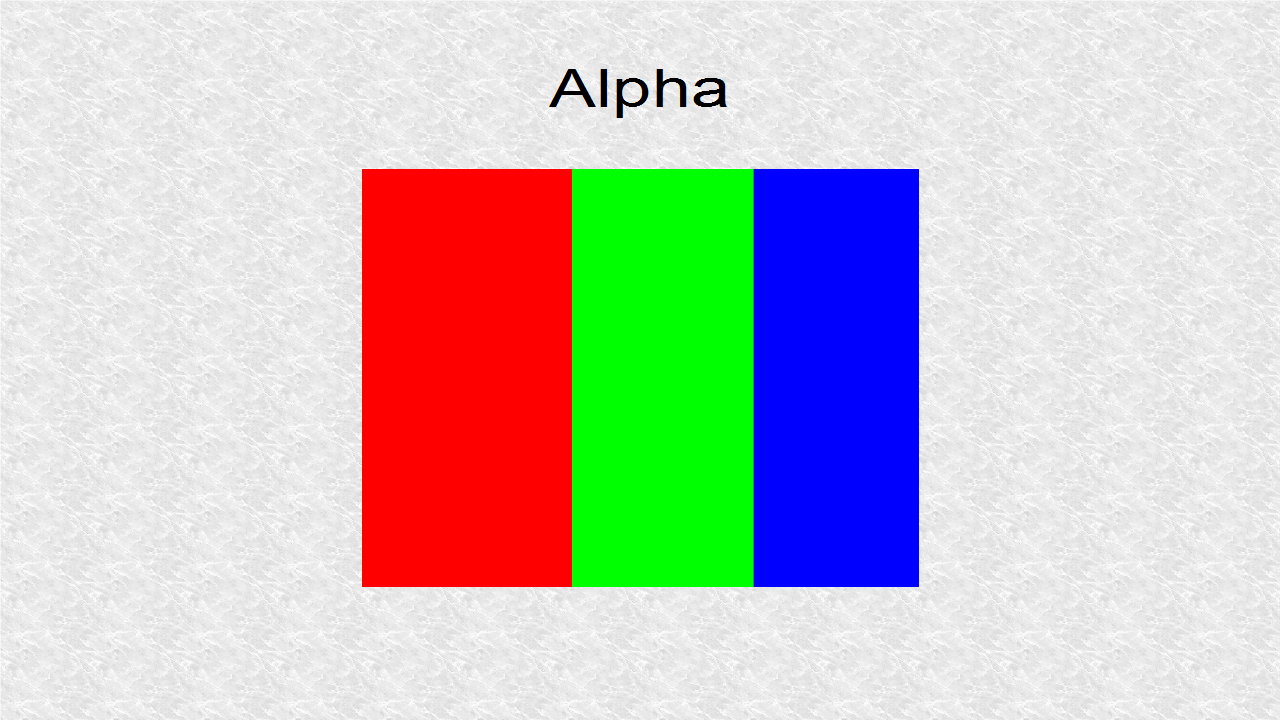
// *** 2. Start (Alpha Texture) materialTerrain.setTexture("Alpha", assetManager. loadTexture("Textures/Ex48/redgreenblue.png")); // *** 2. End
The red texture is based on this image, based on the burlwood pattern in GIMP.
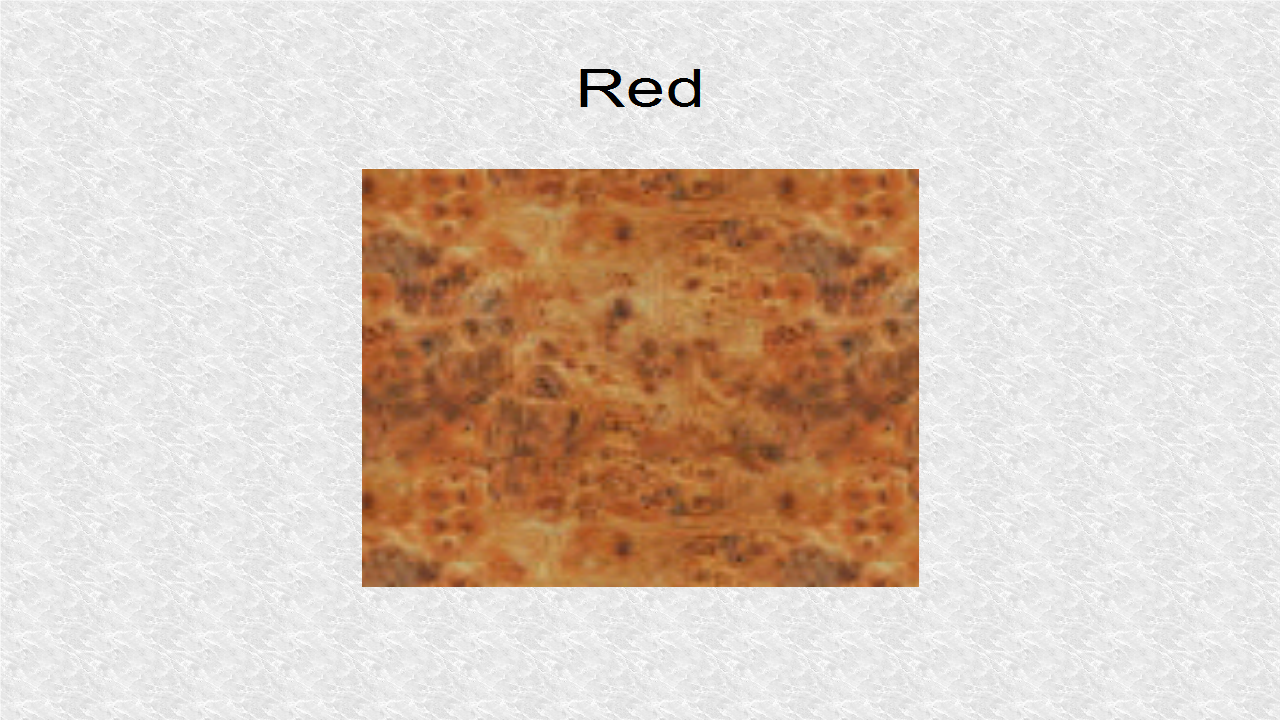
// *** 3. Start (Red Texture) Texture burlwood = assetManager.loadTexture( "Textures/Ex48/burlwood.png"); burlwood.setWrap(Texture.WrapMode.Repeat); materialTerrain.setTexture("Tex1", burlwood); materialTerrain.setFloat("Tex1Scale", 64); // *** 3. End
The green texture is based on this image, based on the craters pattern in GIMP.
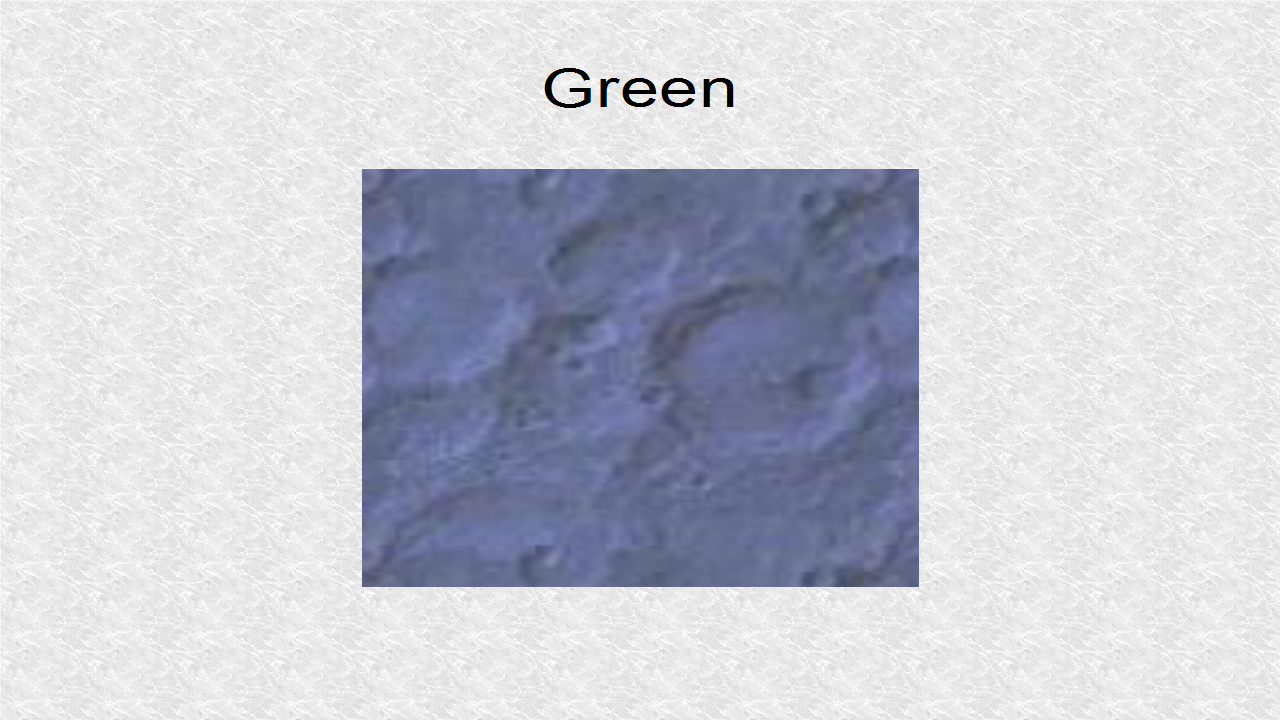
// *** 4. Start (Green Texture) Texture craters = assetManager.loadTexture( "Textures/Ex48/craters.png"); craters.setWrap(Texture.WrapMode.Repeat); materialTerrain.setTexture("Tex2", craters); materialTerrain.setFloat("Tex2Scale", 64); // *** 4. End
The blue texture is based on this image, based on the road pattern in GIMP.
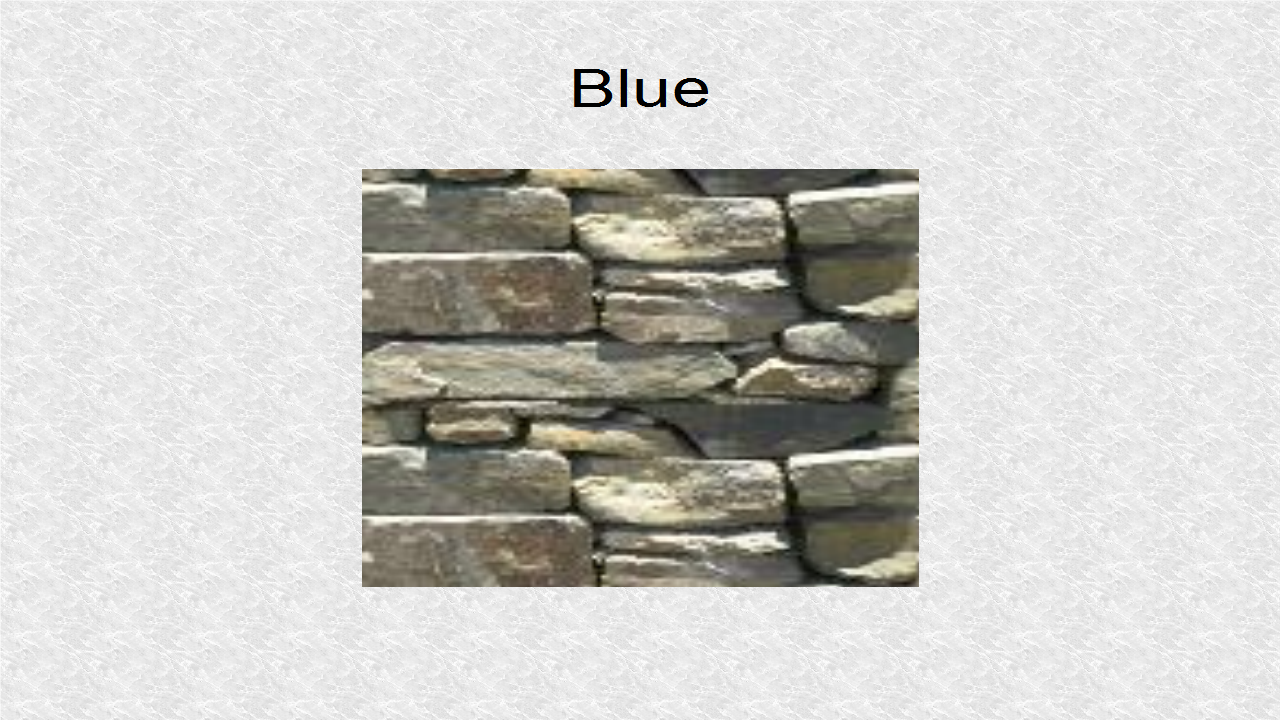
// *** 5. Start (Blue Texture) Texture road = assetManager.loadTexture( "Textures/Ex48/road.png"); road.setWrap(Texture.WrapMode.Repeat); materialTerrain.setTexture("Tex3", road); materialTerrain.setFloat("Tex3Scale", 64); // *** 5. End
This is a gray-scale height map. The three regions corresponds to 0, 128, and 255, so the two steps are almost equal in size.
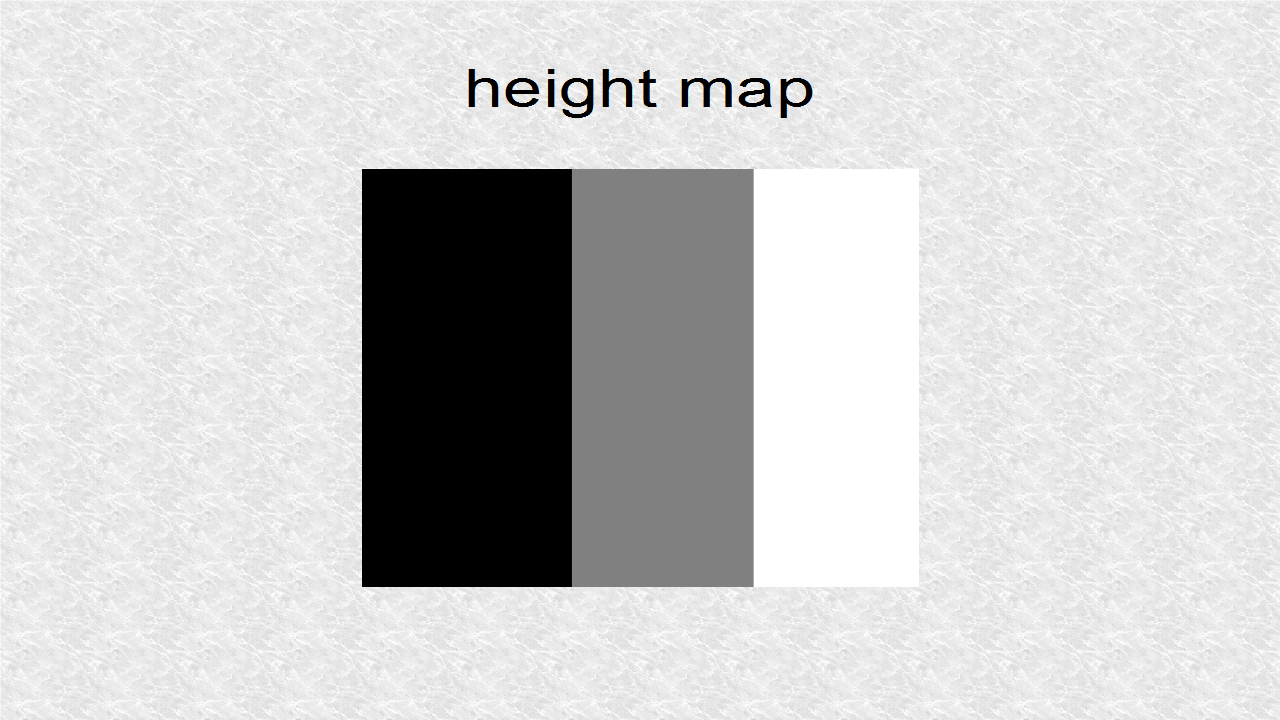
// *** 6. Start (Height map) AbstractHeightMap heightmap = null; Texture heightMapImage = assetManager.loadTexture( "Textures/Ex48/heightmap.png"); heightmap = new ImageBasedHeightMap( heightMapImage.getImage()); heightmap.load(); // *** 6. End
Finally, the terrain can be added to the rootNode.
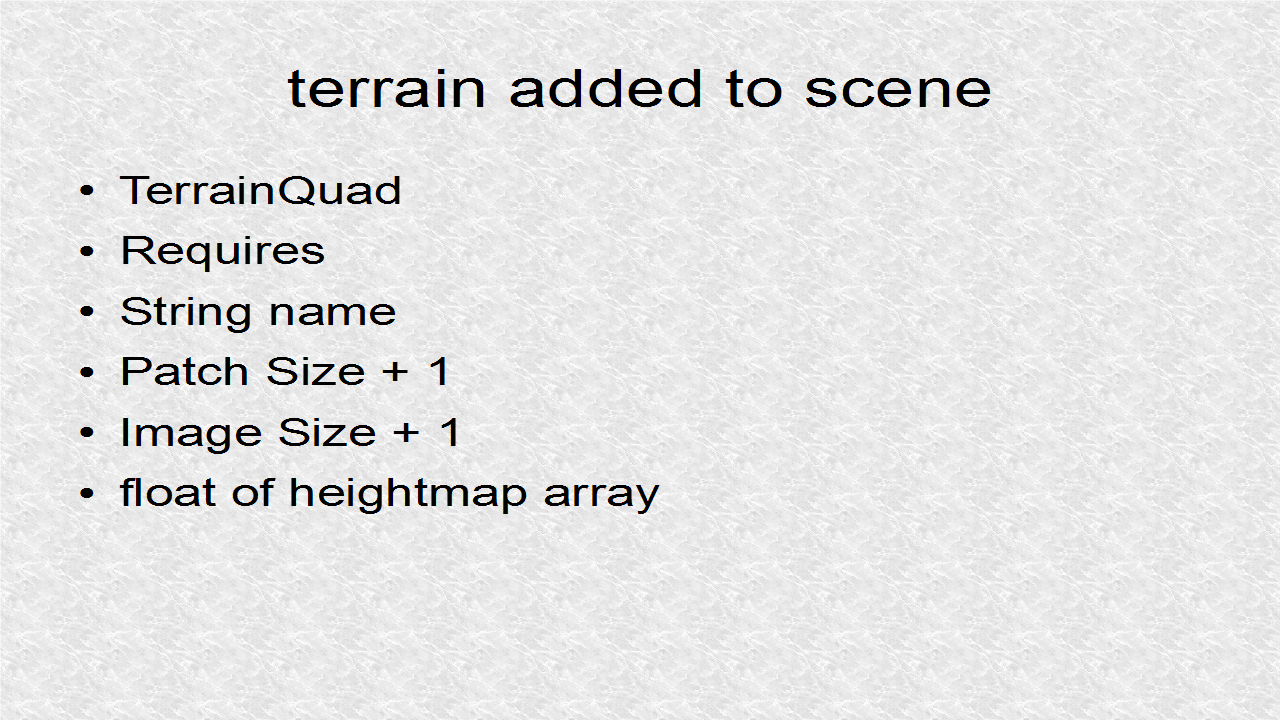
// *** 7. Start (terrain) terrain = new TerrainQuad("terrain", 129, 513, // patch + 1, height size + 1 heightmap.getHeightMap()); // float array terrain.setMaterial(materialTerrain); terrain.setLocalScale(.1f, .1f, .1f); float ang = 45*FastMath.DEG_TO_RAD; terrain.rotate(ang, 0, ang); rootNode.attachChild(terrain); cam.setLocation(new Vector3f(0,0,150)); // far away // *** 7. End
// JMonkey48.java package mygame; import com.jme3.app.SimpleApplication; import com.jme3.material.Material; import com.jme3.math.ColorRGBA; import com.jme3.math.FastMath; import com.jme3.math.Vector3f; import com.jme3.renderer.RenderManager; import com.jme3.terrain.geomipmap.TerrainQuad; import com.jme3.terrain.heightmap.AbstractHeightMap; import com.jme3.terrain.heightmap.ImageBasedHeightMap; import com.jme3.texture.Texture; public class JMonkey48 extends SimpleApplication { public static void main(String[] args) { JMonkey48 app = new JMonkey48(); app.start(); } private TerrainQuad terrain; Material materialTerrain; @Override public void simpleInitApp() { viewPort.setBackgroundColor(ColorRGBA.LightGray); setDisplayFps(false); setDisplayStatView(false); flyCam.setMoveSpeed(100); // faster camera // *** 1. Start (Terrain Unshaded material) materialTerrain = new Material(assetManager, "Common/MatDefs/Terrain/Terrain.j3md"); // *** 1. End // *** 2. Start (Alpha Texture) materialTerrain.setTexture("Alpha", assetManager. loadTexture("Textures/Ex48/redgreenblue.png")); // *** 2. End // *** 3. Start (Red Texture) Texture burlwood = assetManager.loadTexture( "Textures/Ex48/burlwood.png"); burlwood.setWrap(Texture.WrapMode.Repeat); materialTerrain.setTexture("Tex1", burlwood); materialTerrain.setFloat("Tex1Scale", 64); // *** 3. End // *** 4. Start (Green Texture) Texture craters = assetManager.loadTexture( "Textures/Ex48/craters.png"); craters.setWrap(Texture.WrapMode.Repeat); materialTerrain.setTexture("Tex2", craters); materialTerrain.setFloat("Tex2Scale", 64); // *** 4. End // *** 5. Start (Blue Texture) Texture road = assetManager.loadTexture( "Textures/Ex48/road.png"); road.setWrap(Texture.WrapMode.Repeat); materialTerrain.setTexture("Tex3", road); materialTerrain.setFloat("Tex3Scale", 64); // *** 5. End // *** 6. Start (Height map) AbstractHeightMap heightmap = null; Texture heightMapImage = assetManager.loadTexture( "Textures/Ex48/heightmap.png"); heightmap = new ImageBasedHeightMap( heightMapImage.getImage()); heightmap.load(); // *** 6. End // *** 7. Start (terrain) terrain = new TerrainQuad("terrain", 129, 513, // patch + 1, height size + 1 heightmap.getHeightMap()); // float array terrain.setMaterial(materialTerrain); terrain.setLocalScale(.1f, .1f, .1f); float ang = 45*FastMath.DEG_TO_RAD; terrain.rotate(ang, 0, ang); rootNode.attachChild(terrain); cam.setLocation(new Vector3f(0,0,150)); // far away // *** 7. End } @Override public void simpleUpdate(float tpf) { } @Override public void simpleRender(RenderManager rm) { } }
Output:
No comments:
Post a Comment